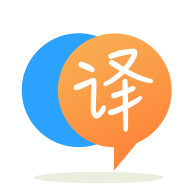
[英]how can get the list of Sql Server instance(or SqlExpress) that are installed and exist in local network
[英]How to list the SQL Server Instances installed on a local machine? ( Only local )
我想知道是否有辦法列出安裝在本地計算機上的 SQL Server 實例。
SqlDataSourceEnumerator
和EnumAvailableSqlServers
不能解決問題,因為我不需要本地網絡上的實例。
直接訪問 Windows 注冊表不是 MS 推薦的解決方案,因為它們可以更改鍵/路徑。 但我同意SmoApplication.EnumAvailableSqlServers()和SqlDataSourceEnumerator.Instance無法在 64 位平台上提供實例。
從 Windows 注冊表獲取數據,請記住x86和x64平台之間注冊表訪問的差異。 64 位版本的 Windows 將數據存儲在系統注冊表的不同部分並將它們組合成視圖。 所以使用RegistryView是必不可少的。
using Microsoft.Win32;
RegistryView registryView = Environment.Is64BitOperatingSystem ? RegistryView.Registry64 : RegistryView.Registry32;
using (RegistryKey hklm = RegistryKey.OpenBaseKey(RegistryHive.LocalMachine, registryView))
{
RegistryKey instanceKey = hklm.OpenSubKey(@"SOFTWARE\Microsoft\Microsoft SQL Server\Instance Names\SQL", false);
if (instanceKey != null)
{
foreach (var instanceName in instanceKey.GetValueNames())
{
Console.WriteLine(Environment.MachineName + @"\" + instanceName);
}
}
}
如果您正在 64 位操作系統上尋找 32 位實例(很奇怪,但可能),您需要查看:
HKEY_LOCAL_MACHINE\SOFTWARE\Wow6432Node\Microsoft\Microsoft SQL Server
您可以使用localOnly = True
調用 EnumAvailableSQlServers
public static DataTable EnumAvailableSqlServers(bool localOnly)
SqlDataSourceEnumerator instance = SqlDataSourceEnumerator.Instance;
System.Data.DataTable table = instance.GetDataSources();
foreach (System.Data.DataRow row in table.Rows)
{
if (row["ServerName"] != DBNull.Value && Environment.MachineName.Equals(row["ServerName"].ToString()))
{
string item = string.Empty;
item = row["ServerName"].ToString();
if(row["InstanceName"] != DBNull.Value || !string.IsNullOrEmpty(Convert.ToString(row["InstanceName"]).Trim()))
{
item += @"\" + Convert.ToString(row["InstanceName"]).Trim();
}
listview1.Items.Add(item);
}
}
您可以使用注冊表在本地系統中獲取 sql server 實例名稱
private void LoadRegKey()
{
RegistryKey key = Registry.LocalMachine.OpenSubKey(@"SOFTWARE\Microsoft\Microsoft SQL Server\Instance Names");
foreach (string sk in key.GetSubKeyNames())
{
RegistryKey rkey = key.OpenSubKey(sk);
foreach (string s in rkey.GetValueNames())
{
MessageBox.Show("Sql instance name:"+s);
}
}
}
結合幾種方法:
using Microsoft.SqlServer.Management.Smo;
using Microsoft.SqlServer.Management.Smo.Wmi;
using System;
using System.Collections.Generic;
using System.Data;
using System.Diagnostics;
using System.Linq;
using Microsoft.Win32;
namespace SqlServerEnumerator
{
class Program
{
static void Main(string[] args)
{
Dictionary<string, Func<List<string>>> methods = new Dictionary<string, Func<List<string>>>
{
{"CallSqlBrowser", GetLocalSqlServerInstancesByCallingSqlBrowser},
{"CallSqlWmi32", GetLocalSqlServerInstancesByCallingSqlWmi32},
{"CallSqlWmi64", GetLocalSqlServerInstancesByCallingSqlWmi64},
{"ReadRegInstalledInstances", GetLocalSqlServerInstancesByReadingRegInstalledInstances},
{"ReadRegInstanceNames", GetLocalSqlServerInstancesByReadingRegInstanceNames},
{"CallSqlCmd", GetLocalSqlServerInstancesByCallingSqlCmd},
};
Dictionary<string, List<string>> dictionary = methods
.AsParallel()
.ToDictionary(v => v.Key, v => v.Value().OrderBy(n => n, StringComparer.OrdinalIgnoreCase).ToList());
foreach (KeyValuePair<string, List<string>> pair in dictionary)
{
Console.WriteLine(string.Format("~~{0}~~", pair.Key));
pair.Value.ForEach(v => Console.WriteLine(" " + v));
}
Console.WriteLine("Press any key to continue.");
Console.ReadKey();
}
private static List<string> GetLocalSqlServerInstancesByCallingSqlBrowser()
{
DataTable dt = SmoApplication.EnumAvailableSqlServers(true);
return dt.Rows.Cast<DataRow>()
.Select(v => v.Field<string>("Name"))
.ToList();
}
private static List<string> GetLocalSqlServerInstancesByCallingSqlWmi32()
{
return LocalSqlServerInstancesByCallingSqlWmi(ProviderArchitecture.Use32bit);
}
private static List<string> GetLocalSqlServerInstancesByCallingSqlWmi64()
{
return LocalSqlServerInstancesByCallingSqlWmi(ProviderArchitecture.Use64bit);
}
private static List<string> LocalSqlServerInstancesByCallingSqlWmi(ProviderArchitecture providerArchitecture)
{
try
{
ManagedComputer managedComputer32 = new ManagedComputer();
managedComputer32.ConnectionSettings.ProviderArchitecture = providerArchitecture;
const string defaultSqlInstanceName = "MSSQLSERVER";
return managedComputer32.ServerInstances.Cast<ServerInstance>()
.Select(v =>
(string.IsNullOrEmpty(v.Name) || string.Equals(v.Name, defaultSqlInstanceName, StringComparison.OrdinalIgnoreCase)) ?
v.Parent.Name : string.Format("{0}\\{1}", v.Parent.Name, v.Name))
.OrderBy(v => v, StringComparer.OrdinalIgnoreCase)
.ToList();
}
catch (SmoException ex)
{
Console.WriteLine(ex.Message);
return new List<string>();
}
catch (Exception ex)
{
Console.WriteLine(ex);
return new List<string>();
}
}
private static List<string> GetLocalSqlServerInstancesByReadingRegInstalledInstances()
{
try
{
// HKEY_LOCAL_MACHINE\SOFTWARE\Microsoft\Microsoft SQL Server\InstalledInstances
string[] instances = null;
using (RegistryKey rk = Registry.LocalMachine.OpenSubKey(@"SOFTWARE\Microsoft\Microsoft SQL Server"))
{
if (rk != null)
{
instances = (string[])rk.GetValue("InstalledInstances");
}
instances = instances ?? new string[] { };
}
return GetLocalSqlServerInstances(instances);
}
catch (Exception ex)
{
Console.WriteLine(ex);
return new List<string>();
}
}
private static List<string> GetLocalSqlServerInstancesByReadingRegInstanceNames()
{
try
{
// HKEY_LOCAL_MACHINE\SOFTWARE\Microsoft\Microsoft SQL Server\Instance Names\SQL
string[] instances = null;
using (RegistryKey rk = Registry.LocalMachine.OpenSubKey(@"SOFTWARE\Microsoft\Microsoft SQL Server\Instance Names\SQL"))
{
if (rk != null)
{
instances = rk.GetValueNames();
}
instances = instances ?? new string[] { };
}
return GetLocalSqlServerInstances(instances);
}
catch (Exception ex)
{
Console.WriteLine(ex);
return new List<string>();
}
}
private static List<string> GetLocalSqlServerInstances(string[] instanceNames)
{
string machineName = Environment.MachineName;
const string defaultSqlInstanceName = "MSSQLSERVER";
return instanceNames
.Select(v =>
(string.IsNullOrEmpty(v) || string.Equals(v, defaultSqlInstanceName, StringComparison.OrdinalIgnoreCase)) ?
machineName : string.Format("{0}\\{1}", machineName, v))
.ToList();
}
private static List<string> GetLocalSqlServerInstancesByCallingSqlCmd()
{
try
{
// SQLCMD -L
int exitCode;
string output;
CaptureConsoleAppOutput("SQLCMD.exe", "-L", 200, out exitCode, out output);
if (exitCode == 0)
{
string machineName = Environment.MachineName;
return output.Split(new[] { "\r\n", "\r", "\n" }, StringSplitOptions.RemoveEmptyEntries)
.Select(v => v.Trim())
.Where(v => !string.IsNullOrEmpty(v))
.Where(v => string.Equals(v, "(local)", StringComparison.Ordinal) || v.StartsWith(machineName, StringComparison.OrdinalIgnoreCase))
.Select(v => string.Equals(v, "(local)", StringComparison.Ordinal) ? machineName : v)
.Distinct(StringComparer.OrdinalIgnoreCase)
.ToList();
}
return new List<string>();
}
catch (Exception ex)
{
Console.WriteLine(ex);
return new List<string>();
}
}
private static void CaptureConsoleAppOutput(string exeName, string arguments, int timeoutMilliseconds, out int exitCode, out string output)
{
using (Process process = new Process())
{
process.StartInfo.FileName = exeName;
process.StartInfo.Arguments = arguments;
process.StartInfo.UseShellExecute = false;
process.StartInfo.RedirectStandardOutput = true;
process.StartInfo.CreateNoWindow = true;
process.Start();
output = process.StandardOutput.ReadToEnd();
bool exited = process.WaitForExit(timeoutMilliseconds);
if (exited)
{
exitCode = process.ExitCode;
}
else
{
exitCode = -1;
}
}
}
}
}
public static string SetServer()
{
string serverName = @".\SQLEXPRESS";
var serverNameList = SqlHelper.ListLocalSqlInstances();
if (serverNameList != null)
{
foreach (var item in serverNameList)
serverName = @"" + item;
}
return serverName;
}
獲取本地服務器名稱
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.