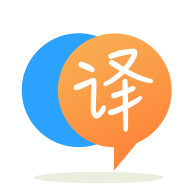
[英]Different stack trace when launching app using “Start Debugging” and “Start Without Debugging”
[英]XmlSerializer crashing only when “Start without debugging”, but not “Step Into”
因此,正如標題所述,XmlSerializer出現了問題。 如果我運行“ Step Into”,它將運行正常,並按原樣保存。 但是,如果我“開始而不調試”,它將一直運行到
XmlSerializer serial = new XmlSerializer(typeof(ObservableCollection<Bill>));
這時它崩潰了。 我已經嘗試過事先將thread.sleep放入,以確保它很清晰,但沒有幫助。 我一直在這里和Google搜尋一整天...請幫助我找出問題所在???
這是完整的代碼:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Data;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Imaging;
using System.Windows.Navigation;
using System.Windows.Shapes;
using Microsoft.Win32;
using System.IO;
using System.Data;
using System.Xml.Serialization;
using System.Collections.ObjectModel;
namespace BudgetHelper
{
/// <summary>
/// Interaction logic for MainWindow.xaml
/// </summary>
public partial class MainWindow : Window
{
public Bills billsClass = new Bills();
public MainWindow()
{
InitializeComponent();
upcomingDueBills.ItemsSource = billsClass.PopulateUpcomingBills();
}
public void RefreshBills()
{
upcomingDueBills.Items.Refresh();
}
private void addBill_Click(object sender, RoutedEventArgs e)
{
AddAccount addAccountWindow = new AddAccount();
addAccountWindow.ShowDialog();
RefreshBills();
}
private void exitMenu_Click(object sender, RoutedEventArgs e)
{
this.Close();
}
private void Window_Closing(object sender, System.ComponentModel.CancelEventArgs e)
{
MessageBoxResult key = MessageBox.Show(
"Are you sure you want to quit",
"Confirm",
MessageBoxButton.YesNo,
MessageBoxImage.Question,
MessageBoxResult.No);
e.Cancel = (key == MessageBoxResult.No);
}
private void openMenu_Click(object sender, RoutedEventArgs e)
{
OpenFileDialog openDialog = new OpenFileDialog();
openDialog.DefaultExt = ".txt";
openDialog.FileName = "Text Documents (.txt)";
Nullable<bool> result = openDialog.ShowDialog();
if (result == true)
{
string filename = openDialog.FileName;
}
}
private void updateBills_Click(object sender, RoutedEventArgs e)
{
upcomingDueBills.Items.Refresh();
}
private void saveMenu_Click(object sender, RoutedEventArgs e)
{
if (upcomingDueBills == null)
{
return;
}
else
{
billsClass.SaveBills();
}
}
}
}
using System;
using System.Collections;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.IO;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Data;
using System.ComponentModel;
using System.Collections.ObjectModel;
using System.Xml.Serialization;
using Microsoft.Win32;
using System.Threading;
namespace BudgetHelper
{
public class Bills : IEditableObject
{
ObservableCollection<Bill> billCollection = new ObservableCollection<Bill>();
public ObservableCollection<Bill> PopulateUpcomingBills()
{
using (StreamReader reader = new StreamReader(@"c:\users\Keven\Documents
\Test.txt"))
{
while (!reader.EndOfStream)
{
string line = reader.ReadLine();
string[] fields = line.Split(new char[] { '|' });
billCollection.Add(new Bill()
{
accountName = fields[0],
projectedDueDate = DateTime.Parse(fields[1]),
projectedAmount = decimal.Parse(fields[2]),
totalDue = decimal.Parse(fields[3]),
category = fields[4],
notes = fields[5],
paidStatus = bool.Parse(fields[6])
});
}
}
return billCollection;
}
public void AddNewBill(string account, DateTime dueDate, decimal amount, decimal
total, string category, string notes, bool isPaid)
{
billCollection.Add(new Bill()
{
accountName = account,
projectedDueDate = dueDate,
projectedAmount = amount,
totalDue = total,
category = category,
notes = notes,
paidStatus = isPaid
});
}
public void SaveBills()
{
SaveFileDialog saveDialog = new SaveFileDialog();
saveDialog.AddExtension = true;
saveDialog.DefaultExt = ".xml";
saveDialog.Filter = "xml files (*.xml)|*.xml|All files (*.*)|*.*";
saveDialog.InitialDirectory = @"C:\Users\Keven\Documents";
saveDialog.OverwritePrompt = true;
saveDialog.Title = "New Account Details";
saveDialog.ValidateNames = true;
if (saveDialog.ShowDialog().Value)
{
Thread.Sleep(2000);
MessageBox.Show("2");
XmlSerializer serial = new XmlSerializer(typeof
(ObservableCollection<Bill>));
using (StreamWriter writer = new StreamWriter(saveDialog.FileName))
{
serial.Serialize(writer, billCollection);
}
}
}
public static MainWindow main;
public object UpcomingDueBills
{
get;
set;
}
public class Bill
{
public string accountName { get; set; }
public DateTime projectedDueDate { get; set; }
public decimal projectedAmount { get; set; }
public decimal totalDue { get; set; }
public string category { get; set; }
public string notes { get; set; }
public bool paidStatus { get; set; }
}
void IEditableObject.BeginEdit()
{
throw new NotImplementedException();
}
void IEditableObject.CancelEdit()
{
throw new NotImplementedException();
}
void IEditableObject.EndEdit()
{
throw new NotImplementedException();
}
}
}
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Data;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Imaging;
using System.Windows.Shapes;
using System.IO;
using Microsoft.Win32;
using System.Windows.Threading;
using System.Threading;
using System.Data;
namespace BudgetHelper
{
/// <summary>
/// Interaction logic for AddAccount.xaml
/// </summary>
public partial class AddAccount : Window
{
public Bills bills = new Bills();
public AddAccount()
{
InitializeComponent();
}
private void cancel_Click(object sender, RoutedEventArgs e)
{
this.Close();
}
private void saveAccountInfo_Click(object sender, RoutedEventArgs e)
{
SaveFileDialog saveDialog = new SaveFileDialog();
saveDialog.AddExtension = true;
saveDialog.DefaultExt = "txt";
saveDialog.InitialDirectory = @"C:\Users\Keven\Documents";
saveDialog.OverwritePrompt = true;
saveDialog.Title = "New Account Details";
saveDialog.ValidateNames = true;
if (saveDialog.ShowDialog().Value)
{
}
this.Close();
}
private void done_Click(object sender, RoutedEventArgs e)
{
bills.AddNewBill(newAccountName.Text, projectedDueDate.SelectedDate.Value,
decimal.Parse(projectedAmount.Text.ToString()),
decimal.Parse(totalDue.Text.ToString()), accountCategory.Text,
accountNotes.Text, (bool)isPaid.IsChecked);
}
}
}
找到了答案,這是自動將應用程序沙盒化為Comodo。 如果其他人遇到此問題,只需轉到Comodo中的Defense +,打開“無法識別的文件”窗口,然后將該程序添加到“受信任的文件”列表中。
另一方面,有人知道是否有辦法讓Comodo信任我正在處理的所有Visual Studio項目嗎?
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.