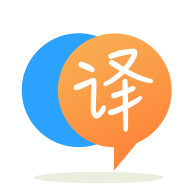
[英]How can I validate the date of birth once the user clicks in another form group?
[英]How can I validate that someone is over 18 from their date of birth?
我正在驗證司機的出生日期,從當前日期起至少應該是 18 歲。
var Dates = $get('<%=ui_txtDOB.ClientID %>');
var Split = Dates.value.split("/");
if (parseInt(Split[2]) > 1993)
{
alert("DOB year should be less than 1993");
Dates.focus();
return false;
}
我使用上面的 JavaScript 驗證來檢查一個人的 DOB 是否高於 18,但它不正確。 我需要檢查今天的日期,它應該在 18 歲以上。如何與當前日期進行比較和檢查?
嘗試這個。
var enteredValue = $get('<%=ui_txtDOB.ClientID %>');;
var enteredAge = getAge(enteredValue.value);
if( enteredAge > 18 ) {
alert("DOB not valid");
enteredValue.focus();
return false;
}
使用這個 function。
function getAge(DOB) {
var today = new Date();
var birthDate = new Date(DOB);
var age = today.getFullYear() - birthDate.getFullYear();
var m = today.getMonth() - birthDate.getMonth();
if (m < 0 || (m === 0 && today.getDate() < birthDate.getDate())) {
age--;
}
return age;
}
<script>
function dobValidate(birth) {
var today = new Date();
var nowyear = today.getFullYear();
var nowmonth = today.getMonth();
var nowday = today.getDate();
var b = document.getElementById('<%=TextBox2.ClientID%>').value;
var birth = new Date(b);
var birthyear = birth.getFullYear();
var birthmonth = birth.getMonth();
var birthday = birth.getDate();
var age = nowyear - birthyear;
var age_month = nowmonth - birthmonth;
var age_day = nowday - birthday;
if (age > 100) {
alert("Age cannot be more than 100 Years.Please enter correct age")
return false;
}
if (age_month < 0 || (age_month == 0 && age_day < 0)) {
age = parseInt(age) - 1;
}
if ((age == 18 && age_month <= 0 && age_day <= 0) || age < 18) {
alert("Age should be more than 18 years.Please enter a valid Date of Birth");
return false;
}
}
</script>
在查看了執行此操作的各種方法后,我決定最簡單的方法是將日期編碼為 8 位整數。 然后,您可以從 DOB 代碼中減去今天的代碼,並檢查它是否大於或等於 180000。
function isOverEighteen(year, month, day) {
var now = parseInt(new Date().toISOString().slice(0, 10).replace(/-/g, ''));
var dob = year * 10000 + month * 100 + day * 1; // Coerces strings to integers
return now - dob > 180000;
}
let TODAY = new Date(Date.now());
let EIGHTEEN_YEARS_BACK = new Date(new Date(TODAY).getDate() + "/" + new Date(TODAY).getMonth() + "/" + (new Date(TODAY).getFullYear() - 18));
let USER_INPUT = new Date("2003/12/13");
// Validate Now
let result = EIGHTEEN_YEARS_BACK > USER_INPUT // true if over 18, false if less than 18
我認為這是最接近的檢查方法。
我的方法是從今天開始找到 18 年前(或任何數字)的日期,然后看看它是否在(大於)他們的出生日期之后。 通過將所有值設置為日期對象,可以輕松進行比較。
function is_of_age(dob, age) {
// dates are all converted to date objects
var my_dob = new Date(dob);
var today = new Date();
var max_dob = new Date(today.getFullYear() - age, today.getMonth(), today.getDate());
return max_dob.getTime() > my_dob.getTime();
}
因為Date object可以解析多種格式的字符串,所以不用太擔心dob是從哪里來的。 只需調用 is_of_age("1980/12/4", 18); 或 is_of_age("2005-04-17", 13); 或者基本上可以解析為日期參數的任何字符串格式或數字。
我最喜歡的方法是這個:
var dateOfBirth = new Date("02/23/1900");
// calculate difference between now and the dateOfBirth (in milliseconds)
var differenceMs = Date.now() - dateOfBirth.getTime();
// convert the calculated difference in date format
var dateFromEpoch = new Date(differenceMs);
// extract year from dateFromEpoch
var yearFromEpoch = dateFromEpoch.getUTCFullYear();
// calculate the age of the user
var age = Math.abs(yearFromEpoch - 1970);
console.log("Age of the user: " + age + " years")
您可以使用以下內容:
var birthDate = new Date("2018-06-21"); var birthDate1 = new Date("1975-06-21"); function underAgeValidate(birthday) { const diff = Date.now() - birthday.getTime(); const ageDate = new Date(diff); let age = Math.abs(ageDate.getUTCFullYear() - 1970); return age < 18; }; console.log(underAgeValidate(birthDate)); console.log(underAgeValidate(birthDate1));
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.