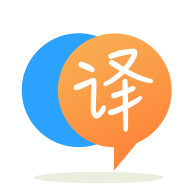
[英]Python binary search-like function to find first number in sorted list greater than a specific value
[英]In Python, how do you find the index of the first value greater than a threshold in a sorted list?
在Python中,如何在排序列表中找到大於閾值的第一個值的索引?
我可以想到幾種方法來做到這一點(線性搜索,手寫二分法,..),但我正在尋找一種干凈且合理有效的方法。 由於這可能是一個非常普遍的問題,我相信有經驗的 SOers 可以提供幫助!
謝謝!
看看bisect 。
import bisect
l = [1, 4, 9, 16, 25, 36, 49, 64, 81, 100]
bisect.bisect(l, 55) # returns 7
將其與線性搜索進行比較:
timeit bisect.bisect(l, 55)
# 375ns
timeit next((i for i,n in enumerate(l) if n > 55), len(l))
# 2.24us
timeit next((l.index(n) for n in l if n > 55), len(l))
# 1.93us
您可能會比使用 itertools 的枚舉/生成器方法獲得更好的時間; 我認為 itertools 為我們所有人的性能販子提供了更快的底層算法實現。 但是 bisect 可能仍然更快。
from itertools import islice, dropwhile
threshold = 5
seq = [1,4,6,9,11]
first_val = islice(dropwhile(lambda x: x<=threshold, seq),0,1)
result = seq.index(first_val)
我想知道這里顯示的二等分方法與文檔示例中為您的問題列出的方法之間的區別,就習慣用法/速度而言。 他們展示了一種查找值的方法,但被截斷到第一行,它返回索引。 我猜因為它被稱為“bisect_right”而不是“bisect”,它可能只從一個方向看。 鑒於您的列表已排序並且您想要大於,這可能是最大的搜索經濟。
from bisect import bisect_right
def find_gt(a, x):
'Find leftmost value(switching this to index) greater than x'
return bisect_right(a, x)
有趣的問題。
大於閾值的最后一個元素的相關索引和值
l = [1, 4, 9, 16, 25, 36, 49, 64, 100, 81, 100]
max((x,i) for i, x in enumerate(l) if x > 4)
(100, 10)
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.