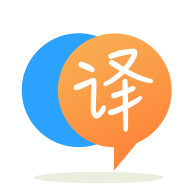
[英]Python: how to get all the first values row-wise from a 2D numpy array when using a 2D boolean mask
[英]how to get the first occurring of an element from every row in a 2D numpy array?
我有一個二維的numpy數組。 我正在尋找每一行中特定元素首次出現的索引。 輸出將是一個(nx 2)數組,其中n是行數,每個條目都包含該特定元素第一次出現的x和y坐標。
非常感謝。
如果我正確理解了該問題,則需要以下內容:
import numpy as N
#
nrows=5
ncols=10
#
a=N.random.random((nrows,ncols))
b=-99*N.ones((nrows,2))
#
for j in range(nrows):
for i in range(ncols):
if(a[j,i]<0.5):
b[j,0]=i
b[j,1]=j
continue
我不確定“ s和y坐標”到底是什么意思,所以我假設您的意思是行和列的位置。
import numpy as np
np.array([(s, list(row).index(your_element)) for s,row in enumerate(your_array)])
注意,如果某行中未包含your_element
,則會引發ValueError
。
以下版本將為您提供輸出,其中可能包含的行數少於輸入的行數,但your_element
中缺少your_element
的情況下,不會引發ValueError
。
np.array([(s, list(row).index(your_element)) for s,row in enumerate(your_array) if your_element in row])
>>> # generate some fake data:
>>> A = NP.random.randint(5, 10, 100).reshape(10, 10)
>>> A
array([[5, 7, 8, 8, 5, 6, 6, 9, 6, 9],
[9, 8, 8, 9, 5, 6, 6, 9, 8, 9],
[8, 5, 6, 7, 8, 9, 5, 8, 6, 7],
[5, 8, 8, 6, 9, 6, 8, 5, 8, 9],
[6, 9, 8, 8, 5, 7, 6, 5, 7, 6],
[7, 8, 6, 7, 6, 6, 7, 8, 6, 8],
[8, 6, 8, 9, 8, 8, 9, 6, 8, 7],
[8, 7, 8, 5, 9, 5, 7, 8, 6, 9],
[9, 6, 5, 9, 9, 8, 8, 9, 8, 8],
[6, 8, 5, 8, 6, 5, 8, 6, 8, 5]])
>>> # sort this 2D array along one axis (i chose row-wise)
>>> A = NP.sort(A, axis=1)
>>> A
array([[5, 5, 6, 6, 6, 7, 8, 8, 9, 9],
[5, 6, 6, 8, 8, 8, 9, 9, 9, 9],
[5, 5, 6, 6, 7, 7, 8, 8, 8, 9],
[5, 5, 6, 6, 8, 8, 8, 8, 9, 9],
[5, 5, 6, 6, 6, 7, 7, 8, 8, 9],
[6, 6, 6, 6, 7, 7, 7, 8, 8, 8],
[6, 6, 7, 8, 8, 8, 8, 8, 9, 9],
[5, 5, 6, 7, 7, 8, 8, 8, 9, 9],
[5, 6, 8, 8, 8, 8, 9, 9, 9, 9],
[5, 5, 5, 6, 6, 6, 8, 8, 8, 8]])
>>> # now diff the sorted array along the same axis
>>> A1 = NP.diff(A ,axis=1)
>>> # A1 contains non-zero values for "first occurrences" and
>>> # zero values for repeat values
>>> A1
array([[0, 1, 0, 0, 1, 1, 0, 1, 0],
[1, 0, 2, 0, 0, 1, 0, 0, 0],
[0, 1, 0, 1, 0, 1, 0, 0, 1],
[0, 1, 0, 2, 0, 0, 0, 1, 0],
[0, 1, 0, 0, 1, 0, 1, 0, 1],
[0, 0, 0, 1, 0, 0, 1, 0, 0],
[0, 1, 1, 0, 0, 0, 0, 1, 0],
[0, 1, 1, 0, 1, 0, 0, 1, 0],
[1, 2, 0, 0, 0, 1, 0, 0, 0],
[0, 0, 1, 0, 0, 2, 0, 0, 0]])
您可以根據需要重新構造結果A1,例如,與原始數組中的A1形狀相同的布爾數組 ,取決於原始矩陣中的值是否代表該值的首次出現,每個單元格均為T / F:
>>> ndx = A1==0
>>> ndx
array([[ True, False, True, True, False, False, True, False, True],
[False, True, False, True, True, False, True, True, True],
[ True, False, True, False, True, False, True, True, False],
[ True, False, True, False, True, True, True, False, True],
[ True, False, True, True, False, True, False, True, False],
[ True, True, True, False, True, True, False, True, True],
[ True, False, False, True, True, True, True, False, True],
[ True, False, False, True, False, True, True, False, True],
[False, False, True, True, True, False, True, True, True],
[ True, True, False, True, True, False, True, True, True]], dtype=bool)
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.