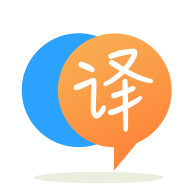
[英]Global const initializing and difference between constructor in .h or .cpp file
[英]Inheriting a constructor in a .h and .cpp file
所以我有兩個類:Object和Player。 我希望Player從Object繼承,因為Object具有我需要的基本功能。 Player具有它的附加功能,這些功能從Object的功能開始擴展。 我有四個文件:Object.cpp,Object.h,Player.cpp和Player.h。
為了舉例說明,我在Player類中添加了一個變量:playerVariable。 我的Object構造函數參數不包含此參數,但是我的Player構造函數包含該參數,因此您可以看到Player擴展了Object。
無論如何,這是我的代碼:
Object.h:
#include <hge.h>
#include <hgesprite.h>
#include <hgeanim.h>
#include <math.h>
class Object{
int x, y;
HTEXTURE tex;
hgeAnimation *anim;
float angle, FPS, velocity;
public:
Object(int x, int y, HTEXTURE tex, float FPS);
//Setters
void SetX(int x);
void SetY(int y);
void SetSpeed(int FPS); //Image Speed
void SetAngle(float angle); //Image Angle
void SetVelocity(float velocity); //Object Speed/Velocity
//Getters
};
Object.cpp:
/*
** Object
**
*/
#include <hge.h>
#include <hgesprite.h>
#include <hgeanim.h>
#include <math.h>
#include "Object.h"
Object::Object(int x, int y, HTEXTURE tex, float FPS){
this->x = x;
this->y = y;
this->tex = tex;
this->FPS = FPS;
}
//Setters
void Object::SetX(int x){
this->x = x;
}
void Object::SetY(int y){
this->x = x;
}
void Object::SetSpeed(int FPS){
this->FPS = FPS;
anim->SetSpeed(FPS);
}
void Object::SetAngle(float angle){
this->angle = angle;
}
void Object::SetVelocity(float velocity){
this->velocity = velocity;
}
//Getters
Player.h:
#include <hge.h>
#include <hgesprite.h>
#include <hgeanim.h>
#include <math.h>
#include "Object.h"
class Player : public Object{
int playerVariable;
public:
Player(int x, int y, HTEXTURE tex, float FPS, int playerVariable);
//Setters
void SetX(int x);
void SetY(int y);
void SetSpeed(int FPS); //Image Speed
void SetAngle(float angle); //Image Angle
void SetVelocity(float velocity); //Object Speed/Velocity
//Getters
};
Player.cpp:
/*
** Object
**
*/
#include <hge.h>
#include <hgesprite.h>
#include <hgeanim.h>
#include <math.h>
#include "Object.h"
#include "Player.h"
Player::Player(int x, int y, HTEXTURE tex, float FPS, playerVariable){
this->x = x;
this->y = y;
this->tex = tex;
this->FPS = FPS;
}
//Setters
void Object::SetX(int x){
this->x = x;
}
void Object::SetY(int y){
this->x = x;
}
void Object::SetSpeed(int FPS){
this->FPS = FPS;
anim->SetSpeed(FPS);
}
void Object::SetAngle(float angle){
this->angle = angle;
}
void Object::SetVelocity(float velocity){
this->velocity = velocity;
}
//Getters
我的問題是,我不確定是否要正確設置此設置。 我看過有關繼承的教程,但是我不知道如何為類設置.h文件和.cpp文件。 有人可以幫忙嗎?
您要兩次定義Object
功能。 您無需定義它,它將從Object
繼承並自動在Player
上可用。
您的Player
構造函數需要調用基礎Object
構造函數。 這是通過構造函數初始化列表完成的:
Player::Player(int x, int y, HTEXTURE tex, float FPS, playerVariable)
: Object(x, y, tex, FPS) // forward arguments to base constructor
{}
您可以以這種方式調用基類構造函數,並指定每個參數:
Player::Player(int x, int y, HTEXTURE tex, float FPS, playerVariable): Object(x, y, tex, FPS) {
它看起來像你實際上並不需要一流的Player
覆蓋SetX(int)
SetY(int)
SetSpeed(int)
SetAngle(float)
,並SetVelocity(float)
的Object
。 如果這樣做,則可以將這些Object
成員函數virtual
。
其他一些注意事項:
Player.cpp
重新定義Object
成員函數。 Player
是一個Object
,因此它繼承了Object
類的成員函數。 另外,如果Object.cpp
和Player.cpp
的實現之間存在任何差異,則由於違反“一個定義規則”,您將收到鏈接器錯誤。 Object
析構函數聲明為virtual
。 您可以使用初始化列表來Object
直接在Player
構造函數中設置Object
的成員:
Player::Player(int x, int y, HTEXTURE tex, float FPS, playerVariable) : Object(x, y, tex, FPS), playerVariable(playerVariable) { }
使用初始化列表效率更高。 在這種情況下甚至有必要,因為Object::x
, Object::y
, Object::tex
和Object::FPS
都是Object
和其朋友專用的。
Object
,您應該提供一個自定義的復制構造函數和copy-assign運算符重載( Object::operator=(const Object&)
)。 C ++編譯器為您提供了這些成員函數的默認實現,但是由於您具有指針成員和HTEXTURE
成員,因此可能需要提供顯式實現來處理深層副本。 復制構造函數和復制分配重載必須始終進行深復制。 FPS
的類型不匹配。 Object::FPS
成員被聲明為float
,但是Object::SetSpeed(int)
的參數是int
。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.