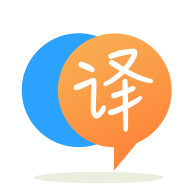
[英]How do you pad a string with a given character or make it empty if length to pad is 0 in C#?
[英]How can I zero pad a 4 character string in C#
我正在使用以下類方法從數字創建Base36字符串:
private const string CharList = "0123456789ABCDEFGHIJKLMNOPQRSTUVWXYZ";
public static String Encode(long input) {
if (input < 0) throw new ArgumentOutOfRangeException("input", input, "input cannot be negative");
char[] clistarr = CharList.ToCharArray();
var result = new Stack<char>();
while (input != 0)
{
result.Push(clistarr[input % 36]);
input /= 36;
}
return new string(result.ToArray());
}
要求之一是該字符串應始終填充零,並且最多應為四位數。 誰能建議我可以編碼前導零並限制函數,使其永遠不會返回超過“ ZZZZ”的方式嗎? C#中是否有一些函數可以做到這一點。 抱歉,這段代碼縮進了。 我不確定為什么縮進不正確。
基數36中的ZZZZ是基數10中的1679615(36 ^ 4-1)。 因此,您可以簡單地測試數字是否大於此數目並拒絕它。
要填充,可以使用String.PadLeft
。
如果總有正好四位數字,那真的很容易:
const long MaxBase36Value = (36L * 36L * 36L * 36L) - 1L;
public static string EncodeBase36(long input)
{
if (input < 0L || input > MaxBase36Value)
{
throw new ArgumentOutOfRangeException();
}
char[] chars = new char[4];
chars[3] = CharList[(int) (input % 36)];
chars[2] = CharList[(int) ((input / 36) % 36)];
chars[1] = CharList[(int) ((input / (36 * 36)) % 36)];
chars[0] = CharList[(int) ((input / (36 * 36 * 36)) % 36)];
return new string(chars);
}
或使用循環:
const long MaxBase36Value = (36L * 36L * 36L * 36L) - 1L;
public static string EncodeBase36(long input)
{
if (input < 0L || input > MaxBase36Value)
{
throw new ArgumentOutOfRangeException();
}
char[] chars = new char[4];
for (int i = 3; i >= 0; i--)
{
chars[i] = CharList[(int) (input % 36)];
input = input / 36;
}
return new string(chars);
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.