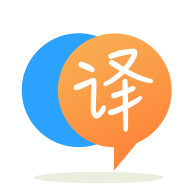
[英]how to get method through reflection of extension overloaded generic method?
[英]HOW TO get an overloaded private/protected method using reflection
using System;
using System.Reflection;
namespace Reflection
{
class Test
{
protected void methodname(int i)
{
Console.WriteLine(("in the world of the reflection- only i"));
Console.Read();
}
protected void methodname(int i, int j)
{
Console.WriteLine(("in the world of the reflection i , j"));
Console.Read();
}
}
class Program
{
static void Main(string[] args)
{
// BindingFlags eFlags = BindingFlags.Default | BindingFlags.Instance | BindingFlags.Public|BindingFlags.NonPublic;
BindingFlags eFlags = BindingFlags.Instance|BindingFlags.NonPublic;
Test aTest = new Test();
MethodInfo mInfoMethod = typeof(Reflection.Test).GetMethod("methodname", eFlags);
mInfoMethod.Invoke(aTest, new object[] { 10 ,20});
}
}
}
我想調用兩個Getmethod()重載方法。 如果我給出方法名稱,則拋出運行時錯誤(ambigous方法調用)。 如何避免這種情況以及如何調用每種方法。
您必須傳遞重載方法的類型,這是反射在出現過載時對您所需方法進行排序的方式。
您不能同時調用這兩個方法,因為它具有不同類型的輸入參數。 您必須准確知道您確切要調用哪一個,並傳遞Type[]
,例如:
// invoking overload with two parameters
MethodInfo mInfoMethod =
typeof(Reflection.Test).GetMethod(
"methodname",
BindingFlags.Instance | BindingFlags.NonPublic,
Type.DefaultBinder,
new[] {typeof (int), typeof (int)},
null);
mInfoMethod.Invoke(aTest, new object[] { 10 ,20});
要么
// invoking overload with one parameters
MethodInfo mInfoMethod =
typeof(Reflection.Test).GetMethod(
"methodname",
vBindingFlags.Instance | BindingFlags.NonPublic,
Type.DefaultBinder,
new[] { typeof (int) },
null);
mInfoMethod.Invoke(aTest, new object[] { 10 });
使用'GetMethods'來檢索所有重載,然后選擇你想要的重載。
請在下面找到一個工作示例:
public class ReflectionSample
{
protected void Method(int i)
{
Console.WriteLine(string.Format("in the world of the reflection- only {0}", i));
Console.Read();
}
protected void Method(int i, int j)
{
Console.WriteLine(string.Format("in the world of the reflection {0} , {1}", i,j));
Console.Read();
}
}
class Program
{
static void Main(string[] args)
{
var eFlags = BindingFlags.Instance | BindingFlags.NonPublic;
var objType = Type.GetType("Sample.ReflectionSample");
var methods = objType.GetMethods(eFlags);
foreach (var method in methods)
{
if (method.Name == "Method")
{
Console.WriteLine("Method name is :" + method.Name);
var parameters = method.GetParameters();
int value = 10;
List<object> param = new List<object>();
for (int i = 0; i < parameters.Count(); i++)
{
param.Add(value * 5);
}
Console.WriteLine(parameters.Count());
method.Invoke(new ReflectionSample(), param.ToArray());
}
}
}
}
你能嘗試這樣嗎?
您必須指定所需的方法:
class SomeType
{
void Foo(int size, string bar) { }
void Foo() { }
}
SomeType obj = new SomeType();
// call with int and string arguments
obj.GetType().GetMethod("Foo", new Type[] { typeof(int), typeof(string)).Invoke(obj, new object[] { 42, "Hello" });
// call without arguments
obj.GetType().GetMethod("Foo", new Type[0]).Invoke(obj, new object[0]);
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.