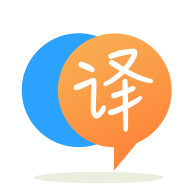
[英]How to Fix: Uppercase first letter of every word in the string - except for one letter words
[英]How can I uppercase the first letter of all words in my string?
首先,我的所有城市都以大寫形式返回,因此我將它們切換為小寫。 我怎樣才能將第一個字母作為大寫字母? 謝謝你的幫助!
List<string> cities = new List<string>();
foreach (DataRow row in dt.Rows)
{
cities.Add(row[0].ToString().ToLower());
**ADDED THIS BUT NOTHING HAPPENED**
CultureInfo.CurrentCulture.TextInfo.ToTitleCase(row[0] as string);
}
return cities;
使用TextInfo.ToTitleCase方法:
System.Globalization.TextInfo.ToTitleCase();
來自MSDN示例的一點,修改為使用OP的代碼:
// Defines the string with mixed casing.
string myString = row[0] as String;
// Creates a TextInfo based on the "en-US" culture.
TextInfo myTI = new CultureInfo("en-US", false).TextInfo;
// Retrieve a titlecase'd version of the string.
string myCity = myTI.ToTitleCase(myString);
全部在一行:
string myCity = new CultureInfo("en-US", false).TextInfo.ToTitleCase(row[0] as String);
我知道我在這里復活了一個鬼,但我遇到了同樣的問題,想分享我認為最好的解決方案。 有幾種方法可以做到這一點,要么拆分字符串並替換第一個字母,要么將其轉換為char數組以獲得更好的性能。 但是,最好的表現是使用正則表達式。
您可以使用一些Regex voodoo來查找每個單詞的第一個字母。 您正在尋找的模式是\\ b \\ w ( \\ b表示單詞的開頭, \\ w表示字母字符)。 使用MatchEvaluator委托(或等效的lambda表達式)來修改字符串(模式找到的第一個字符)。
這是一個字符串的擴展方法,它將大寫ify字符串中每個單詞的第一個字母:
static string UpperCaseFirst(this string input)
{
return Regex.Replace(input, @"\b\w", (Match match)=> match.ToString().ToUpper())
}
正則表達式似乎有點長,但有效
List<string> cities = new List<string>();
foreach (DataRow row in dt.Rows)
{
string city = row[0].ToString();
cities.Add(String.Concat(Regex.Replace(city, "([a-zA-Z])([a-zA-Z]+)", "$1").ToUpper(System.Globalization.CultureInfo.InvariantCulture), Regex.Replace(city, "([a-zA-Z])([a-zA-Z]+)", "$2").ToLower(System.Globalization.CultureInfo.InvariantCulture)));
}
return cities;
new CultureInfo("en-US",false).TextInfo.ToTitleCase(myString);
這是您可以使用的擴展方法。 它支持當前的文化,或允許您傳遞文化。
使用:
cities.Add(row[0].ToString().ToTitleCase()
public static class StringExtension
{
/// <summary>
/// Use the current thread's culture info for conversion
/// </summary>
public static string ToTitleCase(this string str)
{
var cultureInfo = System.Threading.Thread.CurrentThread.CurrentCulture;
return cultureInfo.TextInfo.ToTitleCase(str.ToLower());
}
/// <summary>
/// Overload which uses the culture info with the specified name
/// </summary>
public static string ToTitleCase(this string str, string cultureInfoName)
{
var cultureInfo = new CultureInfo(cultureInfoName);
return cultureInfo.TextInfo.ToTitleCase(str.ToLower());
}
/// <summary>
/// Overload which uses the specified culture info
/// </summary>
public static string ToTitleCase(this string str, CultureInfo cultureInfo)
{
return cultureInfo.TextInfo.ToTitleCase(str.ToLower());
}
}
使用linq:
String newString = new String(str.Select((ch, index) => (index == 0) ? ch : Char.ToLower(ch)).ToArray()); *
您可以使用此方法(或從中創建擴展方法):
static string UpperCaseFirst(this string s)
{
// Check for empty string.
if (string.IsNullOrEmpty(s))
{
return string.Empty;
}
// Return char and concat substring.
return char.ToUpper(s[0]) + s.Substring(1);
}
這是一個快速的小方法:
public string UpperCaseFirstLetter(string YourLowerCaseWord)
{
if (string.IsNullOrEmpty(YourLowerCaseWord))
return string.Empty;
return char.ToUpper(YourLowerCaseWord[0]) + YourLowerCaseWord.Substring(1);
}
public static string UppercaseFirst(string value)
{
// Check for empty string.
if (string.IsNullOrEmpty(value))
{
return string.Empty;
}
// Return char and concat substring.
return char.ToUpper(value[0]) + value.Substring(1);
}
cities.Select(UppercaseFirst).ToList();
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.