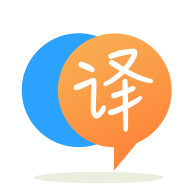
[英]Java - Help me Understanding Access to protected members from subclass (Again)
[英]Java access protected variable from subclass
我正在做一項家庭作業,查看 java 中的 inheritance。我在理解如何從子類訪問超類中的數組時遇到了一些麻煩。 我查看了其他幾個問題,由於我對 java 還很陌生,所以我還是不太明白。
這里是超級 class
import java.text.NumberFormat;
/**
* Bank represents a single Bank containing a number of BankAccounts.
*/
public class Bank {
// Member variables:
/** The array of BankAccount objects contained in this bank. */
protected BankAccount[] myAccounts = new BankAccount[2000];
/** The number of BankAccount objects stored in the array in this bank. */
protected int numberOfAccounts = 0;
// Constructors:
/**
* Creates a Bank.
*/
public Bank() {}
// Methods:
/**
* Creates an account with the name and balance, and adds it to
* the bank's list of accounts.
* If the name already exists, no account will be created.
* @param aName The name for the new account.
* @param aBalance The initial balance for the new account.
*/
public void createAccount( String aName, double aBalance) {
BankAccount existing = this.findAccount( aName);
if( existing != null) return;
BankAccount anAccount = new BankAccount( aBalance, aName);
this.myAccounts[ numberOfAccounts] = anAccount;
this.numberOfAccounts++;
}
/**
* Finds an account in the bank's list of accounts by name.
* If no account is found, this method returns null.
* @param aName The name of the BankAccount to search for.
* @return The BankAccount bearing the given name, if found.
*/
public BankAccount findAccount( String aName) {
BankAccount answer = null;
for( int index = 0; index < numberOfAccounts; index++) {
BankAccount anAccount = this.myAccounts[ index];
if( aName.equals( anAccount.getName())) {
return( anAccount);
}
}
return( answer);
}
/**
* Returns a String which represents a short summary of
* all the accounts in the bank.
* @return A String representation of all accounts and their balances in the bank.
*/
public String toString() {
String answer = "";
NumberFormat currencyFormatter = NumberFormat.getCurrencyInstance();
for( int index = 0; index < numberOfAccounts; index++) {
BankAccount anAccount = this.myAccounts[ index];
String money = currencyFormatter.format( anAccount.getBalance());
answer += anAccount.getName() + " \t" + money + "\n";
}
return( answer);
}
}
這是子類的開始
public class BankSubClass extends Bank{
private double interestPaid;
// Constructor
public BankSubClass(String aName, double aBalance, double aInterest) {
super();
this.interestPaid = aInterest;
}
// Getters
public double getInterestPaid() {return(this.interestPaid);}
// Setters
public void setInterestPaid(double setInterestPaid) {this.interestPaid = setInterestPaid;}
// Other methods
public double endOfYear() {
for (i=0;i<BankAccount.length();i++) {
}
}
}
最后的 for 循環是事情變得有點絆倒的地方。 Netbeans 拋出錯誤“找不到符號:變量 i”。 也許這與我嘗試使用的銀行帳戶數組無關,我不知道。 任何幫助深表感謝
謝謝你的時間!
編輯
所以這是相同作業的延續
感謝大家的回復,您的建議解決了這個問題,但是我目前正在遇到另一個減速帶。 for 循環所在方法背后的想法是遍歷 BankAccount 對象數組,檢查它們是否屬於 InterestAccount 類型(我之前構建的 class),如果是,則調用 yearlyUpdate()來自 class 的方法
這是 InterestAccount class
public class InterestAccount extends BankAccount {
private double interestRate;
// Constructor
/**
* Create and interest bearing bank account with a balance, name,
* and interest rate
* @param aBalance The balance of the account
* @param aName The name tied to the account
* @param myInterestRate The interest rate of the account
*/
public InterestAccount(double aBalance, String aName, double myInterestRate) {
super(aBalance, aName);
this.interestRate = myInterestRate;
}
// Getters
/**
* Gets the interest rate of the account
* @return the interest rate of the account
*/
public double getInterestRate() {return(this.interestRate);}
// Setters
/**
* Sets the interest rate of the account
* @param interestSet The new interest rate of the account
*/
public void setInterestRate(int interestSet) {this.interestRate = interestSet;}
// Other Methods
/**
* Calculates the interest earned on the account over a year
* @return the interest earned over a year
*/
public double yearlyUpdate() {
double answer = (super.getBalance()*this.interestRate);
return answer;
}
}
這是我目前正在使用的超級 class
import java.text.NumberFormat;
/**
* Bank represents a single Bank containing a number of BankAccounts.
*/
public class Bank {
// Member variables:
/** The array of BankAccount objects contained in this bank. */
protected BankAccount[] myAccounts = new BankAccount[2000];
/** The number of BankAccount objects stored in the array in this bank. */
protected int numberOfAccounts = 0;
// Constructors:
/**
* Creates a Bank.
*/
public Bank() {}
// Methods:
/**
* Creates an account with the name and balance, and adds it to
* the bank's list of accounts.
* If the name already exists, no account will be created.
* @param aName The name for the new account.
* @param aBalance The initial balance for the new account.
*/
public void createAccount( String aName, double aBalance) {
BankAccount existing = this.findAccount( aName);
if( existing != null) return;
BankAccount anAccount = new BankAccount( aBalance, aName);
this.myAccounts[ numberOfAccounts] = anAccount;
this.numberOfAccounts++;
}
/**
* Finds an account in the bank's list of accounts by name.
* If no account is found, this method returns null.
* @param aName The name of the BankAccount to search for.
* @return The BankAccount bearing the given name, if found.
*/
public BankAccount findAccount( String aName) {
BankAccount answer = null;
for( int index = 0; index < numberOfAccounts; index++) {
BankAccount anAccount = this.myAccounts[ index];
if( aName.equals( anAccount.getName())) {
return( anAccount);
}
}
return( answer);
}
/**
* Returns a String which represents a short summary of
* all the accounts in the bank.
* @return A String representation of all accounts and their balances in the bank.
*/
public String toString() {
String answer = "";
NumberFormat currencyFormatter = NumberFormat.getCurrencyInstance();
for( int index = 0; index < numberOfAccounts; index++) {
BankAccount anAccount = this.myAccounts[ index];
String money = currencyFormatter.format( anAccount.getBalance());
answer += anAccount.getName() + " \t" + money + "\n";
}
return( answer);
}
}
最后,這是我試圖在其中運行此 for 循環的子類
public class BankSubClass extends Bank{
private double interestPaid;
// Constructor
public BankSubClass(String aName, double aBalance, double aInterest) {
super();
this.interestPaid = aInterest;
}
// Getters
public double getInterestPaid() {return(this.interestPaid);}
// Setters
public void setInterestPaid(double setInterestPaid) {this.interestPaid = setInterestPaid;}
// Other methods
public double endOfYear() {
double trackInterest=0;
for (int i=0;i<numberOfAccounts;i++) {
BankAccount working = myAccounts[i];
boolean hasInterest = working instanceof InterestAccount;
if (hasInterest) {
trackInterest = trackInterest + working.yearlyUpdate();
}
return trackInterest;
}
}
}
currently.netbeans 在嘗試在“工作”時調用它時找不到“yearlyUpdate()”方法我不理解的是,因為前面的代碼驗證了工作 object 是 InterestAccount 類型,它應該有那個方法可用的
謝謝您的幫助!
// Other methods
public double endOfYear() {
for (i=0;i<BankAccount.length();i++) {
}
}
你需要聲明i
// Other methods
public double endOfYear() {
for (int i=0;i<BankAccount.length();i++) {
}
}
就像錯誤所說:我是未定義的。
嘗試:
for (int i=0;i<BankAccount.length();i++)
我猜這仍然很糟糕,因為你想要數組的長度,而不是 BankAccount class(可能未定義)
for (int i=0;i<myAccounts.length();i++)
對於錯誤,您需要在 BankSubClass.endOfYear 中使用它之前定義變量 i。 聲明應該是
for (int i=0;i<BankAccount.length();i++)
你沒有在 for 循環中聲明i
for (int i=0;i<BankAccount.length();i++)
在您的循環中,變量i
未聲明。 此外,由於我認為您希望遍歷數組中的帳戶,因此我認為您應該使用以下內容:
for(int i = 0; < numberOfAccounts; i++)
{
BankAccount bankAccount = myAccounts[i];
// other stuff
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.