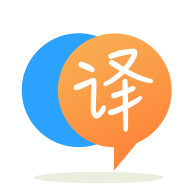
[英]How to make TextBox to use password characters instead of using passwordbox in wpf?
[英]showing password characters on some event for passwordbox
我正在開發一個 windows 電話應用程序。我要求用戶登錄。
在登錄頁面上,用戶必須輸入密碼。
Now what I want is that i give user a check box which when selected should show the characters of the password.
我沒有在密碼框上看到任何屬性來顯示密碼字符。
請建議一些方法來做到這一點。
不要認為使用 PasswordBox 是不可能的……只是一個想法,但是您可以使用隱藏的 TextBox 實現相同的結果,並且當用戶單擊 CheckBox 時,您只需隱藏 PasswordBox 並顯示 TextBox; 如果他再次點擊,你再次切換他們的可見性 state,依此類推......
編輯
就是這樣!
只需添加一個頁面,將 ContentPanel 更改為 StackPanel 並添加此 XAML 代碼:
<PasswordBox x:Name="MyPasswordBox" Password="{Binding Text, Mode=TwoWay, ElementName=MyTextBox}"/>
<TextBox x:Name="MyTextBox" Text="{Binding Password, Mode=TwoWay, ElementName=MyPasswordBox}" Visibility="Collapsed" />
<CheckBox x:Name="ShowPasswordCharsCheckBox" Content="Show password" Checked="ShowPasswordCharsCheckBox_Checked" Unchecked="ShowPasswordCharsCheckBox_Unchecked" />
接下來,在頁面代碼中,添加以下內容:
private void ShowPasswordCharsCheckBox_Checked(object sender, RoutedEventArgs e)
{
MyPasswordBox.Visibility = System.Windows.Visibility.Collapsed;
MyTextBox.Visibility = System.Windows.Visibility.Visible;
MyTextBox.Focus();
}
private void ShowPasswordCharsCheckBox_Unchecked(object sender, RoutedEventArgs e)
{
MyPasswordBox.Visibility = System.Windows.Visibility.Visible;
MyTextBox.Visibility = System.Windows.Visibility.Collapsed;
MyPasswordBox.Focus();
}
這工作正常,但再做一些工作,您就可以完全使用 MVVM 了!
使用默認密碼框無法實現您想要的功能。
您可以在這里找到更多信息: http://social.msdn.microsoft.com/Forums/en/wpf/thread/98d0d4d4-1463-481f-b8b1-711119a6ba99
我創建了一個 MVVM 示例,我也在現實生活中使用它。 請注意,PasswordBox.Password 不是依賴屬性,因此不能直接綁定。 出於安全原因,它是這樣設計的,有關詳細信息,請參見: How to bind to a PasswordBox in MVVM
如果你仍然想這樣做,你必須使用代碼隱藏來建立一個到你的視圖 model 的橋。 我不提供轉換器,因為您可能正在使用自己的轉換器集。 如果沒有,請向谷歌詢問合適的實現。
輸入密碼Window.xaml
<Window x:Class="MyDemoApp.Controls.EnterPasswordWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:local="clr-namespace:MyDemoApp.Controls"
mc:Ignorable="d" d:DataContext="{d:DesignInstance local:EnterPasswordViewModel}"
WindowStartupLocation="CenterOwner" ResizeMode="NoResize" SizeToContent="WidthAndHeight"
Title="Enter Password">
<StackPanel Margin="4">
<TextBlock Margin="4">Please enter a password:</TextBlock>
<TextBox Margin="4" Text="{Binding Password, UpdateSourceTrigger=PropertyChanged}" Visibility="{Binding ShowPassword, Converter={StaticResource BoolToVisibleConverter}}"/>
<PasswordBox Margin="4" Name="PasswordBox" Visibility="{Binding ShowPassword, Converter={StaticResource BoolToCollapsedConverter}}" PasswordChanged="PasswordBox_PasswordChanged"/>
<CheckBox Margin="4" IsChecked="{Binding ShowPassword}">Show password</CheckBox>
<DockPanel>
<Button Margin="4" Width="150" Height="30" IsDefault="True" IsEnabled="{Binding Password, Converter={StaticResource StringIsNotNullOrEmptyConverter}}" Click="Button_Click">OK</Button>
<Button Margin="4" Width="150" Height="30" IsCancel="True" HorizontalAlignment="Right">Cancel</Button>
</DockPanel>
</StackPanel>
</Window>
EnterPasswordWindow.xaml.cs
using System.ComponentModel;
using System.Windows;
using System.Windows.Controls;
namespace MyDemoApp.Controls
{
/// <summary>
/// Interaction logic for EnterPasswordWindow.xaml
/// </summary>
public partial class EnterPasswordWindow : Window
{
public EnterPasswordWindow()
{
InitializeComponent();
DataContext = ViewModel = new EnterPasswordViewModel();
ViewModel.PropertyChanged += ViewModel_PropertyChanged;
}
public EnterPasswordViewModel ViewModel { get; set; }
private void Button_Click(object sender, RoutedEventArgs e)
{
DialogResult = true;
Close();
}
private void ViewModel_PropertyChanged(object sender, PropertyChangedEventArgs e)
{
if (!mSuppressPropertyChangedEvent && e.PropertyName == nameof(ViewModel.Password))
{
PasswordBox.Password = ViewModel.Password;
}
}
private bool mSuppressPropertyChangedEvent;
private void PasswordBox_PasswordChanged(object sender, RoutedEventArgs e)
{
mSuppressPropertyChangedEvent = true;
ViewModel.Password = ((PasswordBox)sender).Password;
mSuppressPropertyChangedEvent = false;
}
}
}
EnterPasswordViewModel.cs
using System.ComponentModel;
using System.Runtime.CompilerServices;
namespace MyDemoApp.Controls
{
public class EnterPasswordViewModel : INotifyPropertyChanged
{
public string Password
{
get => mPassword;
set
{
if (mPassword != value)
{
mPassword = value;
NotifyPropertyChanged();
}
}
}
private string mPassword;
public bool ShowPassword
{
get => mShowPassword;
set
{
if (mShowPassword != value)
{
mShowPassword = value;
NotifyPropertyChanged();
}
}
}
private bool mShowPassword;
public event PropertyChangedEventHandler PropertyChanged;
private void NotifyPropertyChanged([CallerMemberName]string propertyName = null)
{
if (string.IsNullOrEmpty(propertyName))
{
return;
}
PropertyChanged?.Invoke(this, new PropertyChangedEventArgs(propertyName));
}
}
}
您可以創建自己的繼承自文本框的控件,但是在每個字符之后用 * 替換它,將真實值存儲在頁面上的私有變量中。 然后,您可以使用復選框切換文本框中的值是顯示真值還是 * 值。
這不是一個優雅的解決方案,也不是最佳實踐,但我認為如果您願意接受它,它仍然是一個替代方案。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.