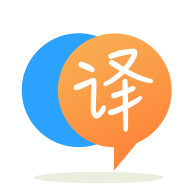
[英]Javascript Object Properties Calculated (on the fly) from Other Object Properties
[英]Property of a Javascript Class calculated from other properties in same Class
這可能是我很遺憾的東西,但是如何根據同一類中其他屬性的值自動重新計算類的屬性?
例如
function Test() {
this.prop1 = 1;
this.prop2 = 2;
this.prop3 = this.prop1 + this.prop2;
}
tester = new Test();
alert (tester.prop1); // expect 1
alert (tester.prop2); // expect 2
alert (tester.prop3); // expect 3
tester.prop1 += 1;
alert (tester.prop1); // expect 2
alert (tester.prop2); // expect 2
alert (tester.prop3); // expect 4
或者我是否需要將prop3設置為= calcProp3(),然后包含如下函數:
this.calcProp3 = function() {
var calc = this.prop1 + this.prop2;
return calc;
}
謝謝大家。
或者我需要將prop3設置為= calcProp3()然后包含一個函數
你有兩個選擇:
使用getter創建一個屬性,當你使用它時看起來像一個簡單的屬性訪問,但實際上是調用一個函數,或者
你的calcProp3
函數是什么,這使得編碼器使用Test
表明他們正在調用一個函數
如果你需要支持像IE8這樣的真正過時的瀏覽器,那么選項2是你唯一的選擇,因為IE8不支持getter。
在2017年你可能會在一個class
定義它(如果不支持ES2015的[又名“ES6”] class
瀏覽器需要進行轉換):
class Test { constructor() { this.prop1 = 1; this.prop2 = 2; } get prop3() { return this.prop1 + this.prop2; } } const t = new Test(); console.log(t.prop3); // 3 t.prop1 = 2; console.log(t.prop3); // 4
如果你想限制自己(在IE8不支持2009年12月公布的規范)ES5的功能,您可以定義上形成消氣Test.prototype
,無論是使用Object.defineProperty
( 規格 , MDN ):
function Test() { this.prop1 = 1; this.prop2 = 2; } Object.defineProperty(Test.prototype, "prop3", { get: function() { return this.prop1 + this.prop2; } }); var t = new Test(); console.log(t.prop3); // 3 t.prop1 = 2; console.log(t.prop3); // 4
...或者通過替換Test.prototype
並使用getter的對象初始化器語法(記得設置constructor
):
function Test() { this.prop1 = 1; this.prop2 = 2; } Test.prototype = { constructor: Test, get prop3() { return this.prop1 + this.prop2; } }; var t = new Test(); console.log(t.prop3); // 3 t.prop1 = 2; console.log(t.prop3); // 4
在2017年,您可能將其定義為使用class
語法的方法(如果舊瀏覽器需要,則進行轉換):
class Test { constructor() { this.prop1 = 1; this.prop2 = 2; } calcProp3() { return this.prop1 + this.prop2; } } const t = new Test(); console.log(t.calcProp3()); // 3 t.prop1 = 2; console.log(t.calcProp3()); // 4
如果你想堅持ES5(實際上在這種情況下是ES3)功能來支持過時的瀏覽器,只需在原型中添加一個函數:
function Test() { this.prop1 = 1; this.prop2 = 2; } Test.prototype.calcProp3 = function calcProp3() { return this.prop1 + this.prop2; }; var t = new Test(); console.log(t.calcProp3()); // 3 t.prop1 = 2; console.log(t.calcProp3()); // 4
Javascript現在支持通過Object.defineProperties()
實現setter和getter的標准方法。
function Test() {
this.prop1 = 1;
this.prop2 = 2;
}
Object.defineProperties(Test.prototype, {
prop3: {
get: function test_prop3_get(){ return this.prop1 + this.prop2 },
},
});
tester = new Test();
alert (tester.prop1); //=> 1
alert (tester.prop2); //=> 2
alert (tester.prop3); //=> 3
tester.prop1 += 1;
alert (tester.prop1); //=> 2
alert (tester.prop2); //=> 2
alert (tester.prop3); //=> 4
在ES6之后你可以在構造函數中計算:
class Test { constructor() { this.prop1 = 1; this.prop2 = 2; this.suma = this.prop1 + this.prop2; } get prop3() { return this.suma; } } const t = new Test(); console.log(t.suma); console.log(t.prop3); // 3
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.