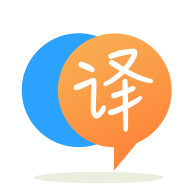
[英]Python & Ctypes: Passing a struct to a function as a pointer to get back data
[英]Python Ctypes Passing in Pointer and Getting Struct Back
這是我在解決實際有用問題之前嘗試工作的一個簡單示例。 C代碼:
typedef struct {
uint32_t seconds;
uint32_t nanoseconds;
} geoTime;
int myTest(geoTime *myTime){
printf("Time: %d %d\n", myTime->seconds, myTime->nanoseconds);
myTime->seconds = myTime->nanoseconds;
geoTime T = {314, 159};
printf("MyTime: %d %d retValue: %d %d\n", myTime->seconds, myTime->nanoseconds, T.seconds, T.nanoseconds);
return 314;
}
Python代碼:
import ctypes
import time
import math
lib_astro = ctypes.CDLL("libastroC.so")
class geoTime(ctypes.Structure):
_fields_ = [("seconds", ctypes.c_uint),
("nanoseconds", ctypes.c_uint)]
now = time.time()
print "Python Now: ", now
now_geoTime = geoTime()
now_geoTime.seconds = ctypes.c_uint(int((math.floor(now))))
now_geoTime.nanoseconds = ctypes.c_uint(int(math.floor(math.modf(now)[0] * 1000000000)))
print "Python geoTime now:", now_geoTime.seconds, now_geoTime.nanoseconds
lib_astro.myTest.argtypes = [ctypes.POINTER(geoTime)]
lib_astro.myTest.restype = geoTime
print "************* ENTERING C ********************"
test = lib_astro.myTest(ctypes.byref(now_geoTime))
print "************* EXITING C **********************"
print "Modified now_geoTime: ",now_geoTime.seconds, now_geoTime.nanoseconds
print "test: ",test
輸出:
Python Now: 1336401085.43
Python geoTime now: 1336401085 432585000
************* ENTERING C ********************
Time: 1336401085 432585000
MyTime: 432585000 432585000 retValue: 314 159
************* EXITING C **********************
Modified now_geoTime: 432585000 432585000
test: 314
上面的代碼完全按照我的預期工作,我的指針進入並被修改,我得到了我的整數。 當我嘗試在C中創建一個geoTime結構並將其返回給Python時,就會出現問題。
在C中添加/修改代碼:
geoTime patTest(geoTime *myTime){
printf("Time: %d %d\n", myTime->seconds, myTime->nanoseconds);
myTime->seconds = myTime->nanoseconds;
geoTime T = {314, 159};
printf("MyTime: %d %d retValue: %d %d\n", myTime->seconds, myTime->nanoseconds, T.seconds, T.nanoseconds);
return T;
}
修改后的Python代碼:
lib_astro.patTest.argtypes = [ctypes.POINTER(geoTime)]
lib_astro.patTest.restype = geoTime
print "************* ENTERING C ********************"
test = lib_astro.patTest(ctypes.byref(now_geoTime))
print "************* EXITING C **********************"
print "Modified now_geoTime: ",now_geoTime.seconds, now_geoTime.nanoseconds
print "Type of test: ",test
print "Information in test: ", test.seconds, test.nanoseconds
一旦我改變我的代碼,C代碼就會變成myTime而不是來自Python的信息,並且返回值被放入now_geoTime而不是test。 關於可能出錯的任何想法? 看起來python代碼沒有像我期望的那樣做,因為C代碼似乎與傳入的值一起正常工作。
上一個例子的輸出:
Python Now: 1336404920.77
Python geoTime now: 1336404920 773674011
************* ENTERING C ********************
Time: 90500 -17037640
MyTime: -17037640 -17037640 retValue: 314 159
************* EXITING C **********************
Modified now_geoTime: 314 159
Type of test: <__main__.geoTime object at 0x82bedf4>
Information in test: 137096800 134497384
任何想法都會非常感激,我一直試圖讓它工作一段時間。 提前致謝!
我切換到64位python / 64位dll,問題就消失了。 當我感覺有點靈感時,我可能會嘗試將它分離到編譯器,os或python但是現在我將用它來運行。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.