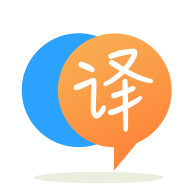
[英]My program has included a DLL but only runs on my computer. My program runs on another computer but it doesn't see the hardware
[英]A program worked fine on my computer but when I sent it on another one with a Dutch Windows it has problems with the DateTimePicker
該程序與C#WinForms和SQL Server 2008一起使用。當我想輸入包含DateTimePicker中的值的數據時,我可以看到措辭是荷蘭語,然后出現有關轉換值的錯誤。 有什么辦法可以對其進行預編程以解決此問題嗎? 我發現了錯誤,就在這里。
try
{
SqlConnection connect = new SqlConnection("Data Source=Localhost\\SQLExpress;Initial Catalog=DataBase;Integrated Security=True");
SqlDataAdapter da = new SqlDataAdapter();
/******************** Inserting ********************/
string query = "INSERT INTO spending VALUES (";
query += "'" + date_dateTimePicker.Value + "', "; // Date
query += "'" + Convert.ToDecimal(amount_spent_textBox.Text) + "', "; // Amount spent
query += "'" + spent_on_textBox.Text + "')"; // Spent on
connect.Open();
da.InsertCommand = new SqlCommand(query, connect);
da.InsertCommand.ExecuteNonQuery();
connect.Close();
}
catch (Exception error)
{
MessageBox.Show(error.ToString());
}
事情變得越來越復雜。.在嘗試將dateTimePicker值插入數據庫時,出現了此錯誤,就像使用上面的代碼一樣。 它在我的計算機上可以正常工作,但在這里無法正常工作。 有人可以解釋嗎? 這是錯誤:
使用的代碼:
string update = "UPDATE table SET the_date = '" + the_date_dateTimePicker.Value + "' WHERE instance_ID = 1";
connect.Open();
da.InsertCommand = new SqlCommand(update, connect);
da.InsertCommand.ExecuteNonQuery();
connect.Close();
好的,這是我現在正在處理的表單的完整代碼,該代碼顯示了此錯誤 。 大多數表單的結構都是這樣的,因此,如果我正確地做到這一點,其余的表單應該不會有任何問題。 我正在計算機上對此進行測試,因此,如果它在這里可以正常工作,那么它也應該可以在這里正常工作。
看一看,我不知道該怎么辦了。
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
using System.Data.SqlClient;
namespace TheNamespace
{
public partial class submit_spending : Form
{
public submit_spending()
{
InitializeComponent();
}
private void submit_button_Click(object sender, EventArgs e)
{
try
{
SqlConnection connect = new SqlConnection("Data Source=Localhost\\SQLExpress;Initial Catalog=TheDataBase;Integrated Security=True");
SqlDataAdapter da = new SqlDataAdapter();
/******************** Inserting ********************/
string query = "INSERT INTO spending VALUES (@date, @amount_spent, @spent_on)";
SqlCommand command = new SqlCommand(query);
command.Parameters.AddWithValue("date", date_dateTimePicker.Value);
command.Parameters.AddWithValue("amount_spent", Convert.ToDecimal(amount_spent_textBox.Text));
command.Parameters.AddWithValue("spent_on", spent_on_textBox.Text);
connect.Open();
da.InsertCommand = new SqlCommand(query, connect);
da.InsertCommand.ExecuteNonQuery();
connect.Close();
if (MessageBox.Show("Submitted.", "Spending submitted", MessageBoxButtons.OK) == DialogResult.OK)
{
this.Close();
}
}
catch (Exception error)
{
MessageBox.Show(error.ToString());
}
}
private void cancel_button_Click(object sender, EventArgs e)
{
this.Close();
}
}
}
您應該使用Parameters.AddWithValue()
方法。 我希望它可以正確格式化DateTime
。
string cmdText = "UPDATE table SET colX = @value WHERE id = @currId";
var cmd = new SqlCommand(cmdText, conn);
cmd.Parameters.AddWithValue("value", dateTimePicker1.Value);
cmd.Parameters.AddWithValue("currId", id);
可能您對日期時間格式有疑問,可以通過將系統日期時間格式更改為與正在使用的計算機相同的格式來解決,或者可以在代碼中進行一些其他工作。 對於自定義日期時間格式,請在此處查看: http : //msdn.microsoft.com/zh-cn/library/8kb3ddd4.aspx
理想情況下,您應該在T-SQL語句中使用datetime
參數編寫應用程序,以使轉換永遠不會出現。
您可以使用的另一種方法是在應用程序使用的連接上添加SET DATEFORMAT
。 因此,您可以將格式更改為您的應用程序期望/使用的格式。
我認為您需要在command.Parameters中為日期分配值時指定SqlDBType,並且請交叉檢查您在insert語句中指定的參數名稱與parameters.Add中指定的參數。
string sql = "INSERT INTO spending VALUES (@date, @amount_spent, @spent_on)";
using (var cn = new SqlConnection("..connection string.."))
using (var cmd = new SqlCommand(sql, cn))
{
cmd.Parameters.Add("@date", SqlDbType.DateTime).Value = date_dateTimePicker.Value;
command.Parameters.AddWithValue("@amount_spent",Convert.ToDecimal(amount_spent_textBox.Text));
command.Parameters.AddWithValue("@spent_on", spent_on_textBox.Text);
connect.Open();
有時最好使用不變文化在數據庫中存儲日期/時間之類的東西。您可以嘗試使用CultureInfo.Invariantculture
DateTime date = date_dateTimePicker.Value.Date;
string sDate = date.ToString("dd-MM-yy", System.Globalization.CultureInfo.InvariantCulture);
DateTime dateInsert = Convert.ToDateTime(sDate);
您可能可以使用具有區域性不變格式的顯式ToString來確保它在任何語言環境中均有效。 這有點hack,但替換此行:
command.Parameters.AddWithValue("date", date_dateTimePicker.Value);
這應該工作:
command.Parameters.AddWithValue("date", date_dateTimePicker.Value.ToString("s"));
這將為您提供ISO 8601格式的日期時間字符串,這是具有明確規范的國際標准。 另外,此格式不受SQL Server實例上的SET DATEFORMAT或SET LANGUAGE設置影響。
只需使用dateTime.ToString("s")
,它將以通用格式為您提供日期時間,而與計算機的文化設置無關。
"UPDATE table SET the_date = '" + the_date_dateTimePicker.Value + "' WHERE instance_ID = 1";
使用默認的CultureInfo轉換為String的情況在這里發生。 根據在工作站上選擇的區域設置,可以將DateTime轉換為“ 29/05/2012”和“ 05/29/2012”。 但是,可能為其他區域設置配置了Sql Server,因此您遇到了問題(sql server無法解析傳遞的DateTime字符串表示形式)
如果您以后不想再遇到與CultureInfo相關的其他問題,則必須使用參數化查詢。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.