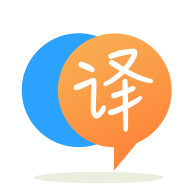
[英]C# Array of strings contains string part from another array of strings
[英]c# - search a string array using strings from another string array
就像標題中一樣。 我得到了一個字符串數組和第二個字符串數組。 我想以這種模式顯示結果:第一個數組的第一個元素-然后出現在第一個數組的第一個元素中的第二個數組的所有元素。 在第一數組的第二個元素和第二數組的所有元素之后出現在第一數組的第二個元素之后。 等等。 例如:
string[] arrayA = {"Lorem ipsum dolor sit amet, justo", "notgin like good cold beer"};
string[] arrayB = {"justo","beer","lorem"}
for (int i = 0; i < arrayA.Length; i++)
{
Console.WriteLine(arrayA[i]);
for (int j = 0; j < arrayB.Length; j++)
{
int controlIndex = arrayA[i].IndexOf(arrayB[j]);
if (controlIndex != -1)
{
Console.Write(" :--contains-->" + arrayB[j]);
}
}
}
因此結果應如下所示:
但是我的結果是:-Lorem ipsum dolor坐着,justo:-包含-> justo -notgin像優質冷啤酒:-包含-> beer 。
因此,如您所見,沒有列出lorem
如果您將問題分解成若干部分,這一點都不難。 首先,擺脫對數組和索引的處理。 只需使用IEnumerable<T>
,它將使您的生活更輕松。
這是我的看法:
首先,您要從數組needles
haystack
找到所有字符串。
public static IEnumerable<string> MatchingStrings(string haystack, IEnumerable<string> needles)
{
return needles.Where(needle => haystack.Contains(needle));
}
這將返回一個IEnumerable所有字符串從needles
是一部分haystack
。
然后,您只想簡單地遍歷所有搜索字符串,我稱其為haystacks
。
static void Main(string[] args)
{
var haystacks = new[] {
"Lorem ipsum dolor sit amet, justo",
"notgin like good cold beer"
};
var needles = new[] {"justo", "beer", "lorem"};
foreach (var haystack in haystacks) {
Console.Write(haystack + " contains --> ");
var matches = MatchingStrings(haystack, needles);
Console.WriteLine(String.Join(",", matches));
}
Console.ReadLine();
}
請注意, String.Contains()
區分大小寫 。 因此,“ Lorem”將與“ lorem”不匹配。 如果您想要這種行為,則必須先將它們轉換為小寫。
public static IEnumerable<string> MatchingStringsCaseInsensitive(string haystack, IEnumerable<string> needles)
{
var h = haystack.ToLower();
return needles.Where(needle => h.Contains(needle.ToLower()));
}
string[] arrayA = {"Lorem ipsum dolor sit amet, justo", "notgin like good cold beer"};
string[] arrayB = {"justo","beer","lorem"};
foreach (var s in arrayA)
{
Console.Write(s + " contains: " +
string.Join(",", arrayB.Where(s.Contains)));
}
如果您想忽略大小寫:
foreach (var s in arrayA)
{
Console.Write(s + " contains: " +
string.Join(",", arrayB.Where(x =>
s.IndexOf(x, StringComparison.OrdinalIgnoreCase) != -1)));
}
foreach(var a in arrayA)
{
Console.WriteLine("a: " + a);
Console.WriteLine("bs: " +
String.Join(", ", arrayB.Where(b => a.IndexOf(b) > -1)));
}
另外,如果您不關心大小寫,則a.IndexOf(b)
將是a.IndexOf(b, StringComparison.OrdinalIgnoreCase)
。
這是Linq解決方案:
var result = arrayA.Select(a => new{
A = a,
bContains = arrayB.Where(b => a.IndexOf(b, 0, StringComparison.CurrentCultureIgnoreCase) > -1)
});
foreach(var x in result)
{
Console.WriteLine("{0}:--contains-->{1}", x.A, string.Join(",", x.bContains));
}
這是一個演示: http : //ideone.com/wxl6I
這是我的嘗試
string[] arrayA = {"lorem ipsum dolor sit amet, justo", "notgin like good cold beer"};
string[] arrayB = {"justo", "beer", "lorem"};
foreach (var item in from a in arrayA from b in arrayB where a.Contains(b) select new {a, b})
{
Console.WriteLine(item.a);
Console.WriteLine(item.b);
}
注意: Contains
是區分大小寫的比較,您需要編寫一個自定義比較器(因為其他答案已經完成)
Lorem是大寫的。 嘗試使用不區分大小寫的搜索: .indexOf(string, StringComparison.CurrentCultureIgnoreCase)
我已經給了您所有可能的答案,但是contains方法會產生一個問題,在下述情況下,它也會返回true。
reference_string = "Hello Stack Overflow"
test_string = "Over"
所以盡量避免包含,因為包含方法將
“返回一個值,該值指示指定的System.String對象是否出現在此字符串中”
注意:添加了StringComparer.OrdinalIgnoreCase以便區分大小寫。
/// <summary>
/// Compares using binary search
/// </summary>
/// <param name="input"> search input</param>
/// <param name="reference"> reference string</param>
/// <returns> returns true or false</returns>
public bool FatMan(string input, string reference)
{
string[] temp = reference.Split();
Array.Sort(temp);
List<string> refe = new List<string> { };
refe.AddRange(temp);
string[] subip = input.Split();
foreach (string str in subip)
{
if (refe.BinarySearch(str, StringComparer.OrdinalIgnoreCase) < 0)
{
return false;
}
}
return true;
}
/// <summary>
/// compares using contains method
/// </summary>
/// <param name="input"> search input</param>
/// <param name="reference"> reference string</param>
/// <returns> returns true or false</returns>
public bool Hiroshima(string input, string reference)
{
string[] temp = reference.Split();
Array.Sort(temp);
List<string> refe = new List<string> { };
refe.AddRange(temp);
string[] subip = input.Split();
foreach (string str in subip)
{
if (!refe.Contains(str, StringComparer.OrdinalIgnoreCase))
{
return false;
}
}
return true;
}
public bool Nakashaki(string input, string reference)
{
string[] temp = reference.Split();
Array.Sort(temp);
List<string> refe = new List<string> { };
refe.AddRange(temp);
string[] subip = input.Split();
int result = (from st in subip where temp.Contains(st, StringComparer.OrdinalIgnoreCase) select st).Count();
if (result <= 0)
{
return false;
}
return true;
}
/// <summary>
/// compares using contains method
/// </summary>
/// <param name="input"> search input</param>
/// <param name="reference"> reference string</param>
/// <returns> returns true or false</returns>
public bool LittleBoy(string input, string reference)
{
string[] subip = input.Split();
foreach (string str in subip)
{
if (!reference.Contains(str))
{
return false;
}
}
return true;
}
bool oupt ;
string[] strarray1 = new string[3]{"abc","def","ghi" };
string[] strarray2 = new string[4] { "648", "888", "999", "654" };
if (strarray1.All(strarray.Contains))
oupt = true;
else
oupt = false;
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.