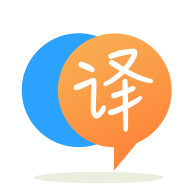
[英]What is the difference between object of an abstract class and list of objects of abstract class?
[英]Returning a Sorted List of Objects based on an Abstract Class
我有一個抽象類,它定義了整個站點中社交使用對象的基本行為(社交協作對象)。
internal abstract class SCO
{
public double HotScore { get; set; }
public double UpVotes { get; set; }
public double DownVotes { get; set; }
public double VoteTotal { get; set; }
public DateTime Created { get; set; }
public SCO(DBItem item, List<Vote> votes )
{
var voteMeta = InformationForThisVote(votes, item.ID);
UpVotes = voteMeta.UpVotes;
DownVotes = voteMeta.DownVotes;
VoteTotal = UpVotes - DownVotes;
HotScore = Calculation.HotScore(Convert.ToInt32(UpVotes), Convert.ToInt32(DownVotes), Convert.ToDateTime(item["Created"]));
Created = Convert.ToDateTime(item["Created"]);
}
private static VoteMeta InformationForThisVote(List<Vote> votes, int itemId)
{
// Loop through votes, find matches by id,
// and record number of upvotes and downvotes
}
private User GetCreatorFromItemValue(DBItem item)
{
// Cast User Object from property of DataBase information
}
}
以下是繼承對象的示例:
class Post : SCO
{
public string Summary { get; set; }
public Uri Link { get; set; }
public Uri ImageUrl { get; set; }
public Post(DBItem item, List<Vote> votes)
: base(item, votes)
{
Summary = (string) item["Summary"];
Link = new UriBuilder((string) item["Link"]).Uri;
ImageUrl = new UriBuilder((string) item["ImageUrl"]).Uri;
}
}
這些類的共同之處在於,大部分時間它們將作為Sorted Collection返回。 這里的難點是你不能將一個集合嵌入到一個抽象類中,因為沒有辦法實例化抽象類本身。
到目前為止我所做的工作是將Sort方法作為此處顯示的摘要的一部分:
protected static List<ESCO> SortedItems(List<ESCO> escoList, ListSortType sortType)
{
switch (sortType)
{
case ListSortType.Hot:
escoList.Sort(delegate(ESCO p1, ESCO p2) { return p2.HotScore.CompareTo(p1.HotScore); });
return escoList;
case ListSortType.Top:
escoList.Sort(delegate(ESCO p1, ESCO p2) { return p2.VoteTotal.CompareTo(p1.VoteTotal); });
return escoList;
case ListSortType.Recent:
escoList.Sort(delegate(ESCO p1, ESCO p2) { return p2.Created.CompareTo(p1.Created); });
return escoList;
default:
throw new ArgumentOutOfRangeException("sortType");
}
}
這允許我在我的繼承子類中有這個:
public static List<Post> Posts(SPListItemCollection items, ListSortType sortType, List<Vote> votes)
{
var returnlist = new List<ESCO>();
for (int i = 0; i < items.Count; i++) { returnlist.Add(new Post(items[i], votes)); }
return SortedItems(returnlist, sortType).Cast<Post>().ToList();
}
這有效,但感覺有點笨重。 我仍然在我的Sub-Classes中重復了很多代碼,我覺得這個演員是不必要的性能演繹。
我如何最好地提供一種方法來返回基於抽象類的對象的排序列表,這些抽象類以相同的方式排序,具有相同的屬性?
看起來不需要抽象類,因為沒有抽象成員。 使用具體的基類(如果需要添加虛擬成員)不是最好的嗎?
更好的是,使用包含排序所需的所有成員(分數,投票等)的界面; 將接口的集合傳遞給sort方法。
編輯這是一個簡化的例子:
internal interface ISCO
{
double HotScore { get; set; }
}
class SCO : ISCO
{
public double HotScore { get; set; }
public static IEnumerable<T> Sort<T>(IEnumerable<T> items) where T : ISCO
{
var sorted = items.ToList();
sorted.Sort();
return sorted;
}
}
然后,您可以為每個派生類使用該單一排序方法:
List<Post> PostItems = // ...
IEnumerable<Post> sorted = SCO.Sort<Post>(PostItems);
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.