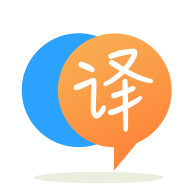
[英]How do i determine which files are created or manipulated by a specific process programatically in c# windows OS
[英]How to programatically (C#) determine the pages count of .docx files
我有大約400個.docx格式的文件,我需要在#pages中確定每個文件的長度。
所以,我想編寫C#代碼來選擇包含文檔的文件夾,然后返回每個.docx文件的#pages。
為了說明如何做到這一點,我剛剛創建了一個基於.NET 4.5和一些Microsoft Office 2013 COM對象的C#控制台應用程序。
using System;
using Microsoft.Office.Interop.Word;
namespace WordDocStats
{
class Program
{
// Based on: http://www.dotnetperls.com/word
static void Main(string[] args)
{
// Open a doc file.
var application = new Application();
var document = application.Documents.Open(@"C:\Users\MyName\Documents\word.docx");
// Get the page count.
var numberOfPages = document.ComputeStatistics(WdStatistic.wdStatisticPages, false);
// Print out the result.
Console.WriteLine(String.Format("Total number of pages in document: {0}", numberOfPages));
// Close word.
application.Quit();
}
}
}
為此,您需要引用以下COM對象:
兩個COM對象使您可以訪問所需的命名空間。
有關如何引用正確組件的詳細信息,請參閱“3.設置工作環境:”部分: http : //www.c-sharpcorner.com/UploadFile/amrish_deep/WordAutomation05102007223934PM/WordAutomation.aspx
有關通過C#進行Word自動化的快速和一般性介紹,請參閱: http : //www.dotnetperls.com/word
- 更新
可以在此處找到有關方法Document.ComputeStatistics
,該方法可讓您訪問頁數: http : //msdn.microsoft.com/en-us/library/microsoft.office.tools.word.document.computestatistics.aspx
如文檔中所示,該方法采用WdStatistic
枚舉,使您能夠檢索不同類型的統計信息,例如頁面總量。 有關您可以訪問的完整統計信息的概述,請參閱WdStatistic
枚舉文檔,該文檔可在此處找到: http : //msdn.microsoft.com/en-us/library/microsoft.office。 interop.word.wdstatistic.aspx
使用DocumentFormat.OpenXml.dll你可以在C:\\ Program Files \\ Open XML SDK \\ V2.0 \\ lib中找到dll
示例代碼:
DocumentFormat.OpenXml.Packaging.WordprocessingDocument doc = DocumentFormat.OpenXml.Packaging.WordprocessingDocument.Open(docxPath, false);
MessageBox.Show(doc.ExtendedFilePropertiesPart.Properties.Pages.InnerText.ToString());
要使用DocumentFormat.OpenXml.Packaging.WordprocessingDocument類,您需要在項目中添加以下引用
DocumentFormat.OpenXml.dll和Windowsbase.dll
現代解決方案(基於Jignesh Thakker的回答 ):Open XML SDK不再存在,但它在Github上發布 ,甚至支持.NET Core。 您不需要在服務器/運行的計算機上使用MS Office。
安裝Nuget包 :
Install-Package DocumentFormat.OpenXml
代碼:
using DocumentFormat.OpenXml.Packaging;
private int CountWordPage(string filePath)
{
using (var wordDocument = WordprocessingDocument.Open(filePath, false))
{
return int.Parse(wordDocument.ExtendedFilePropertiesPart.Properties.Pages.Text);
}
}
你可以使用Spire.Doc頁數是免費的:)
using Spire.Doc;
public sealed class TestNcWorker
{
[TestMethod]
public void DocTemplate3851PageCount()
{
var docTemplate3851 = Resource.DocTemplate3851;
using (var ms = new MemoryStream())
{
ms.Write(docTemplate3851, 0, docTemplate3851.Length);
Document document = new Document();
document.LoadFromStream(ms, FileFormat.Docx);
Assert.AreEqual(2,document.PageCount);
}
var barCoder = new BarcodeAttacher("8429053", "1319123", "HR3514");
var barcoded = barCoder.AttachBarcode(docTemplate3851).Value;
using (var ms = new MemoryStream())
{
ms.Write(barcoded, 0, barcoded.Length);
Document document = new Document();
document.LoadFromStream(ms, FileFormat.Docx);
Assert.AreEqual( 3, document.PageCount);
}
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.