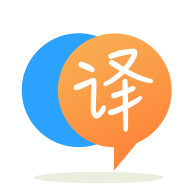
[英]How to check for date in C# textbox and display whether the date is before or later than DateTime.Now
[英]C# attribute to check whether one date is earlier than the other
我的MVC4 Prject有一個ViewModel,其中包含兩個DateTime屬性:
[Required]
[DataType(DataType.Date)]
public DateTime RentDate { get; set; }
[Required]
[DataType(DataType.Date)]
public DateTime ReturnDate { get; set; }
有沒有一種簡單的方法可以將C#屬性用作[Compare("someProperty")]
來檢查天氣,RentDate屬性的值早於ReturnDate的值?
這是一個非常快速的基本實現(不進行錯誤檢查等),該操作應該按照您的要求進行(僅在服務器端...不會對asp.net客戶端進行JavaScript驗證)。 我還沒有測試過,但應該足以讓您入門。
using System;
using System.ComponentModel.DataAnnotations;
namespace Test
{
[AttributeUsage(AttributeTargets.Property)]
public class DateGreaterThanAttribute : ValidationAttribute
{
public DateGreaterThanAttribute(string dateToCompareToFieldName)
{
DateToCompareToFieldName = dateToCompareToFieldName;
}
private string DateToCompareToFieldName { get; set; }
protected override ValidationResult IsValid(object value, ValidationContext validationContext)
{
DateTime earlierDate = (DateTime)value;
DateTime laterDate = (DateTime)validationContext.ObjectType.GetProperty(DateToCompareToFieldName).GetValue(validationContext.ObjectInstance, null);
if (laterDate > earlierDate)
{
return ValidationResult.Success;
}
else
{
return new ValidationResult("Date is not later");
}
}
}
public class TestClass
{
[DateGreaterThan("ReturnDate")]
public DateTime RentDate { get; set; }
public DateTime ReturnDate { get; set; }
}
}
看來您正在使用DataAnnotations,所以另一種選擇是在視圖模型中實現IValidatableObject
:
public IEnumerable<ValidationResult> Validate(ValidationContext validationContext)
{
if (this.RentDate > this.ReturnDate)
{
yield return new ValidationResult("Rent date must be prior to return date", new[] { "RentDate" });
}
}
如果您使用的是.Net Framework 3.0或更高版本,則可以將其作為類擴展名進行...
/// <summary>
/// Determines if a <code>DateTime</code> falls before another <code>DateTime</code> (inclusive)
/// </summary>
/// <param name="dt">The <code>DateTime</code> being tested</param>
/// <param name="compare">The <code>DateTime</code> used for the comparison</param>
/// <returns><code>bool</code></returns>
public static bool isBefore(this DateTime dt, DateTime compare)
{
return dt.Ticks <= compare.Ticks;
}
/// <summary>
/// Determines if a <code>DateTime</code> falls after another <code>DateTime</code> (inclusive)
/// </summary>
/// <param name="dt">The <code>DateTime</code> being tested</param>
/// <param name="compare">The <code>DateTime</code> used for the comparison</param>
/// <returns><code>bool</code></returns>
public static bool isAfter(this DateTime dt, DateTime compare)
{
return dt.Ticks >= compare.Ticks;
}
不確定在MVC / Razor中使用它,但是..
您可以使用DateTime.Compare(t1,t2)-t1和t2是您要比較的時間。 它將返回-1、0或1,具體取決於結果。
在此處閱讀更多信息: http : //msdn.microsoft.com/zh-cn/library/system.datetime.compare.aspx
模型:
[DateCorrectRange(ValidateStartDate = true, ErrorMessage = "Start date shouldn't be older than the current date")]
public DateTime StartDate { get; set; }
[DateCorrectRange(ValidateEndDate = true, ErrorMessage = "End date can't be younger than start date")]
public DateTime EndDate { get; set; }
屬性類:
[AttributeUsage(AttributeTargets.Property)]
public class DateCorrectRangeAttribute : ValidationAttribute
{
public bool ValidateStartDate { get; set; }
public bool ValidateEndDate { get; set; }
protected override ValidationResult IsValid(object value, ValidationContext validationContext)
{
var model = validationContext.ObjectInstance as YourModelType;
if (model != null)
{
if (model.StartDate > model.EndDate && ValidateEndDate
|| model.StartDate > DateTime.Now.Date && ValidateStartDate)
{
return new ValidationResult(string.Empty);
}
}
return ValidationResult.Success;
}
}
框架中沒有類似的東西。 常見的類似屬性是RequiredIf
。 在這篇文章中對此進行了描述。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.