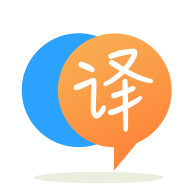
[英]How to determine a session variable contains data table is null or empty in C#
[英]What is the best way to determine a session variable is null or empty in C#?
在 ASP.NET C# 中檢查會話變量是否存在的最佳方法是什么?
我喜歡將String.IsNullOrEmpty()
用於字符串,並想知道Session
是否有類似的方法。 目前我所知道的唯一方法是:
var session;
if (Session["variable"] != null)
{
session = Session["variable"].ToString();
}
else
{
session = "set this";
Session["variable"] = session;
}
遵循別人所說的。 我傾向於有兩層:
核心層。 這是在添加到幾乎所有 Web 應用程序項目的 DLL 中。 在這個我有一個 SessionVars 類,它為會話狀態 getter/setter 做繁重的工作。 它包含如下代碼:
public class SessionVar
{
static HttpSessionState Session
{
get
{
if (HttpContext.Current == null)
throw new ApplicationException("No Http Context, No Session to Get!");
return HttpContext.Current.Session;
}
}
public static T Get<T>(string key)
{
if (Session[key] == null)
return default(T);
else
return (T)Session[key];
}
public static void Set<T>(string key, T value)
{
Session[key] = value;
}
}
注意獲取任何類型的泛型。
然后我還為特定類型添加 Getter/Setter,尤其是字符串,因為我經常更喜歡使用 string.Empty 而不是 null 來處理呈現給用戶的變量。
例如:
public static string GetString(string key)
{
string s = Get<string>(key);
return s == null ? string.Empty : s;
}
public static void SetString(string key, string value)
{
Set<string>(key, value);
}
等等...
然后我創建包裝器來抽象它並將它帶到應用程序模型中。 例如,如果我們有客戶詳細信息:
public class CustomerInfo
{
public string Name
{
get
{
return SessionVar.GetString("CustomerInfo_Name");
}
set
{
SessionVar.SetString("CustomerInfo_Name", value);
}
}
}
你明白嗎? :)
注意:在為已接受的答案添加評論時只是有一個想法。 使用狀態服務器將對象存儲在 Session 中時,始終確保對象是可序列化的。 在網絡農場上嘗試使用泛型來保存對象可能太容易了,並且它會蓬勃發展。 我在工作時部署在 Web 場上,因此在核心層中添加了對我的代碼的檢查,以查看對象是否可序列化,這是封裝 Session Getter 和 Setter 的另一個好處:)
這幾乎就是你這樣做的方式。 但是,您可以使用更短的語法。
sSession = (string)Session["variable"] ?? "set this";
這是說如果會話變量為空,則將 sSession 設置為“設置此”
將它包裝在一個屬性中可能會使事情變得更加優雅。
string MySessionVar
{
get{
return Session["MySessionVar"] ?? String.Empty;
}
set{
Session["MySessionVar"] = value;
}
}
那么你可以把它當作一個字符串。
if( String.IsNullOrEmpty( MySessionVar ) )
{
// do something
}
c# 3.0 中的“as”表示法非常簡潔。 由於所有會話變量都是可為空的對象,因此您可以獲取值並將其放入您自己的類型化變量中,而不必擔心拋出異常。 大多數對象都可以通過這種方式處理。
string mySessionVar = Session["mySessionVar"] as string;
我的概念是你應該把你的 Session 變量拉到局部變量中,然后適當地處理它們。 始終假設您的 Session 變量可以為 null,並且永遠不要將它們轉換為不可為 null 的類型。
如果您需要一個不可為空的類型變量,您可以使用 TryParse 來獲取它。
int mySessionInt;
if (!int.TryParse(mySessionVar, out mySessionInt)){
// handle the case where your session variable did not parse into the expected type
// e.g. mySessionInt = 0;
}
這個方法也不假設Session變量中的對象是字符串
if((Session["MySessionVariable"] ?? "").ToString() != "")
//More code for the Code God
所以基本上在將空變量轉換為字符串之前用空字符串替換空變量,因為ToString
是Object
類的一部分
在我看來,做到這一點的最簡單、清晰易讀的方法是:
String sVar = (string)(Session["SessionVariable"] ?? "Default Value");
它可能不是最有效的方法,因為即使在默認情況下(將字符串轉換為字符串),它也會轉換默認字符串值,但是如果您將其設為標准編碼實踐,您會發現它適用於所有數據類型,並且易於閱讀。
例如(一個完全虛假的例子,但它表明了這一點):
DateTime sDateVar = (datetime)(Session["DateValue"] ?? "2010-01-01");
Int NextYear = sDateVar.Year + 1;
String Message = "The Procrastinators Club will open it's doors Jan. 1st, " +
(string)(Session["OpeningDate"] ?? NextYear);
我喜歡泛型選項,但它似乎有點矯枉過正,除非你希望到處都需要它。 可以修改擴展方法以專門擴展 Session 對象,以便它具有“安全”獲取選項,如 Session.StringIfNull("SessionVar") 和 Session["SessionVar"] = "myval"; 它打破了通過 Session["SessionVar"] 訪問變量的簡單性,但它是干凈的代碼,並且仍然允許在需要時驗證是否為 null 或字符串。
檢查空/空是這樣做的方法。
處理對象類型不是要走的路。 聲明一個嚴格類型並嘗試將對象強制轉換為正確的類型。 (並使用強制轉換提示或轉換)
private const string SESSION_VAR = "myString";
string sSession;
if (Session[SESSION_VAR] != null)
{
sSession = (string)Session[SESSION_VAR];
}
else
{
sSession = "set this";
Session[SESSION_VAR] = sSession;
}
抱歉有任何語法違規,我是每日 VB'er
通常,我為會話中的項目創建具有強類型屬性的 SessionProxy。 訪問這些屬性的代碼檢查空值並將轉換為正確的類型。 這樣做的好處是我所有與會話相關的項目都保存在一個地方。 我不必擔心在代碼的不同部分使用不同的鍵(並想知道為什么它不起作用)。 通過依賴注入和模擬,我可以使用單元測試對其進行全面測試。 如果遵循 DRY 原則,還可以讓我定義合理的默認值。
public class SessionProxy
{
private HttpSessionState session; // use dependency injection for testability
public SessionProxy( HttpSessionState session )
{
this.session = session; //might need to throw an exception here if session is null
}
public DateTime LastUpdate
{
get { return this.session["LastUpdate"] != null
? (DateTime)this.session["LastUpdate"]
: DateTime.MinValue; }
set { this.session["LastUpdate"] = value; }
}
public string UserLastName
{
get { return (string)this.session["UserLastName"]; }
set { this.session["UserLastName"] = value; }
}
}
我也喜歡在屬性中包裝會話變量。 這里的 setter 是微不足道的,但我喜歡編寫 get 方法,因此它們只有一個退出點。 為此,我通常會檢查 null 並將其設置為默認值,然后再返回會話變量的值。 像這樣的東西:
string Name
{
get
{
if(Session["Name"] == Null)
Session["Name"] = "Default value";
return (string)Session["Name"];
}
set { Session["Name"] = value; }
}
}
這樣就可以檢查這樣的密鑰是否可用
if (Session.Dictionary.ContainsKey("Sessionkey")) --> return Bool
您在使用 .NET 3.5 嗎? 創建一個 IsNull 擴展方法:
public static bool IsNull(this object input)
{
input == null ? return true : return false;
}
public void Main()
{
object x = new object();
if(x.IsNull)
{
//do your thing
}
}
如果您知道它是一個字符串,則可以使用 String.IsEmptyOrNull() 函數。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.