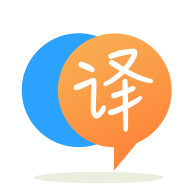
[英]Using Selenium WebDriver in Python to Open Link in New Tab/Window
[英]How to open a new window on a browser using Selenium WebDriver for python?
我正在尝试在浏览器中使用 selenium 为 python 打开一个新选项卡或一个新的 window。打开一个新选项卡或一个新的 window 并不重要,打开浏览器的第二个实例才重要。
我已经尝试了几种不同的方法,但都没有成功。
切换到一个不存在的 window,希望在找不到 window 时打开一个新的 window:
driver.switch_to_window(None)
迭代open windows(虽然目前只有一个)
for handle in driver.window_handles: driver.switch_to_window(handle)
试图模拟键盘按键
from selenium.webdriver.common.keys import Keys driver.send_keys(Keys.CONTROL + 'T')
这个特别的问题是似乎无法将密钥直接发送到浏览器,只能发送到这样的特定元素:
driver.find_element_by_id('elementID').send_keys(Keys.CONTROL + 'T')
然而,当像这样的命令被发送到一个元素时,它似乎什么都不做。 我试图找到页面上最顶部的 HTML 元素并将密钥发送到该元素,但再次遇到失败:
driver.find_element_by_id('wrapper').send_keys(Keys.CONTROL + 'T')
我在网上找到的另一个版本,无法验证其有效性或缺乏有效性,因为我不确定需要导入哪个类/模块
act = ActionChains(driver)
act.key_down(browserKeys.CONTROL)
act.click("").perform()
act.key_up(browserKeys.CONTROL)
使用不同语法的东西非常相似(我不确定其中一个或两个是否是正确的语法)
actions.key_down(Keys.CONTROL)
element.send_keys('t')
actions.key_up(Keys.CONTROL)
你怎么做这样的事情
driver = webdriver.Firefox() #First FF window
second_driver = webdriver.Firefox() #The new window you wanted to open
根据要与之交互的窗口,相应地发送命令
print driver.title #to interact with the first driver
print second_driver.title #to interact with the second driver
对于所有失望的选民:
OP要求“ it is only important that a second instance of the browser is opened.
”。 这个答案并不包含每个用例的所有可能需求。 以下其他答案可能适合您的特定需求。
您可以使用execute_script
打开新窗口。
driver = webdriver.Firefox()
driver.get("https://linkedin.com")
# open new tab
driver.execute_script("window.open('https://twitter.com')")
print driver.current_window_handle
# Switch to new window
driver.switch_to.window(driver.window_handles[-1])
print " Twitter window should go to facebook "
print "New window ", driver.title
driver.get("http://facebook.com")
print "New window ", driver.title
# Switch to old window
driver.switch_to.window(driver.window_handles[0])
print " Linkedin should go to gmail "
print "Old window ", driver.title
driver.get("http://gmail.com")
print "Old window ", driver.title
# Again new window
driver.switch_to.window(driver.window_handles[1])
print " Facebook window should go to Google "
print "New window ", driver.title
driver.get("http://google.com")
print "New window ", driver.title
我建议在Firefox上使用CTRL + N
命令来减少内存使用,而不是创建新的浏览器实例。
import selenium.webdriver as webdriver
from selenium.webdriver.common.keys import Keys
browser = webdriver.Firefox()
body = browser.find_element_by_tag_name('body')
body.send_keys(Keys.CONTROL + 'n')
Dhiraj已经提到了切换和控制窗口的方法。
driver = webdriver.Chrome()
driver.execute_script("window.open('');")
driver.get('first url')
driver.switch_to.window(driver.window_handles[1])
driver.get('second url')
driver.switch_to.new_window()
会新开一个window。
然后你可以搜索web
driver.get("https://google.com")
在JavaScript中,window和tab没有区别。
driver.window_handles
认为两者相同。
但是,如果需要打开一个新的 window,有几种方法:(所需的导入见[3])
模拟 ctrl+N。 在新版本中不起作用。 看到这个评论。
( ActionChains(driver).key_down(Keys.CONTROL).send_keys('n').key_up(Keys.CONTROL).perform() )
或者
body = driver.find_element_by_tag_name('body') body.send_keys(Keys.CONTROL + 'n')
按住 shift 键,然后单击页面上的链接——仅当页面上有任何链接时才有效。
( ActionChains(driver).key_down(Keys.SHIFT).click(driver.find_element_by_tag_name("a")).key_up(Keys.SHIFT).perform() )
如果没有,可以创建一个...(修改当前页面!)
driver.execute_script('''{ let element=document.createElement("a"); element.href="about:home"; element.id="abc" element.text="1"; document.body.appendChild(element); element.scrollIntoView(); }''') ( ActionChains(driver).key_down(Keys.SHIFT).click(driver.find_element_by_id("abc")).key_up(Keys.SHIFT).perform() )
...或导航到一个页面。 (如果您在 session 的开头执行此操作,或者打开一个新选项卡执行此操作并稍后将其关闭,这不是问题)
driver.get("data:text/html,<a href=about:blank>1</a>") ( ActionChains(driver).key_down(Keys.SHIFT).click(driver.find_element_by_tag_name("a")).key_up(Keys.SHIFT).perform() )
看起来像黑客。
(对于 Firefox)关闭“在选项卡而不是新窗口中打开链接”选项,然后执行window.open()
。
从设置到在新标签页中打开浏览器链接,在新标签页中打开外部链接 window | Firefox 支持论坛 | Mozilla 支持和Browser.link.open_newwindow - MozillaZine 知识库:
browser.link.open_newwindow - 用于 Firefox 选项卡中的链接
3 = 将新的 window 转移到新标签页(默认)
2 = 允许链接打开一个新的 window
1 = 强制将新的 window 放入同一个选项卡
此选项应设置为 2。 [2]
请注意,根据https://github.com/SeleniumHQ/selenium/issues/2106#issuecomment-320238039-permalink ,无法使用FirefoxProfile
[1] 设置该选项。
options=webdriver.FirefoxOptions() options.set_preference("browser.link.open_newwindow", 2) driver=Firefox(firefox_options=options) driver.execute_script("window.open()")
对于 Selenium 4.0.0(预发布版。目前您可以安装它,例如pip install selenium==4.0.0b4
),可以执行以下操作:
driver.execute( selenium.webdriver.remote.command.Command.NEW_WINDOW, {"type": "window"} )
取自这个答案的技术。
[1]:在当前版本中,出于某种原因,这有效并将browser.link.open_newwindow
设置为 2
fp = webdriver.FirefoxProfile()
fp.set_preference("browser.link.open_newwindow", 3)
fp.default_preferences["browser.link.open_newwindow"]=3
fp.DEFAULT_PREFERENCES["browser.link.open_newwindow"]=3
driver=Firefox(firefox_profile=fp)
虽然这使期权价值保持在 3
driver=Firefox()
[2]:如果该值设置为3
, window.open()
将在新选项卡中打开,而不是在新的 window 中打开。
[3]:所有必需的进口:
from selenium import webdriver
from selenium.webdriver import Firefox, FirefoxProfile
from selenium.webdriver.common.keys import Keys
from selenium.webdriver.common.action_chains import ActionChains
[4]:此答案仅涵盖如何打开新的 window。要切换到新的 window 并返回,请使用WebDriverWait
和driver.switch_to.window(driver.window_handles[i])
。 有关详细信息,请参阅https://stackoverflow.com/a/51893230/5267751 。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.