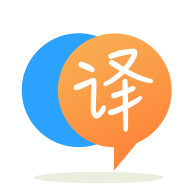
[英]Why does fork() fail on MacOs Big Sur if the executable that runs it is deleted?
[英]How/Why can fork() fail
我目前正在研究 C 中的 fork() 函数。我理解它的作用(我认为)。 我的问题是为什么我们在下面的程序中检查它?
#include <stdio.h>
#include <unistd.h>
#include <stdlib.h>
int main()
{
int pid;
pid=fork();
if(pid<0)/* why is this here? */
{
fprintf(stderr, "Fork failed");
exit(-1);
}
else if (pid == 0)
{
printf("Printed from the child process\n");
}
else
{
printf("Printed from the parent process\n");
wait(pid);
}
}
在这个程序中,我们检查返回的 PID 是否 < 0,这表示失败。 为什么 fork() 会失败?
从手册页:
Fork() will fail and no child process will be created if:
[EAGAIN] The system-imposed limit on the total number of pro-
cesses under execution would be exceeded. This limit
is configuration-dependent.
[EAGAIN] The system-imposed limit MAXUPRC (<sys/param.h>) on the
total number of processes under execution by a single
user would be exceeded.
[ENOMEM] There is insufficient swap space for the new process.
(这是来自 OS X 手册页,但其他系统上的原因类似。)
fork
可能会失败,因为你生活在现实世界中,而不是一些无限递归的数学幻想世界,因此资源是有限的。 特别是, sizeof(pid_t)
是有限的,这对fork
可能成功的次数(没有任何进程终止)设置了 256^sizeof(pid_t) 的硬上限。 除此之外,您还有其他资源需要担心,例如内存。
可能没有足够的可用内存来创建新进程。
显然它可能会失败(不是真的失败,而是无限挂起),因为以下事情结合在一起:
也可以看看
https://github.com/jvm-profiling-tools/async-profiler/issues/97
https://github.com/gperftools/gperftools/issues/704
https://bugzilla.redhat.com/show_bug.cgi?id=645528
例子:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
int main()
{
size_t sz = 32*(size_t)(1024*1024*1024);
char *p = (char*)malloc(sz);
memset(p, 0, sz);
fork();
return 0;
}
建造:
gcc -pg tmp.c
跑:
./a.out
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.