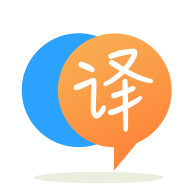
[英]Can abstract class be override in derived class without implementing in base class
[英]Can you override one of the abstract methods in a base class with another base class?
我有一个具有一些抽象方法的基类,并且有21个类从该基类继承。 现在,对于那些抽象方法之一,我想使用21个类中的6个的通用实现来实现它,因此我考虑创建另一个可以做到这一点的基类。
我乐于接受建议,但我的主要目的是在当前基类和21个类之间创建另一个基类,以防止不必要时在21个类中的6个中重复相同的代码。
这是代码示例,说明这种情况:
public abstract class FooBase
{
public abstract string Bar();
public abstract string SomeMethod();
public virtual string OtherMethod()
{
return this.SomeMethod();
}
}
public abstract class AnotherBase : FooBase
{
public abstract string Bar();
public abstract string SomeMethod();
public override OtherMethod()
{
//this is the common method used by 6 of the classes
return "special string for the 6 classes";
}
}
public class Foo1 : FooBase
{
public override string Bar()
{
//do something specific for the Foo1 class here
return "Foo1 special string";
}
public override string SomeMethod()
{
//do something specific for the Foo1 class here
return "Foo1 special string";
}
}
public class Another2 : AnotherBase
{
public override string Bar()
{
//do something specific for the Another2 class here
return "Another special string";
}
public override string SomeMethod()
{
//do something specific for the Another2 class here
return "Another2 special string";
}
}
是的,您可以从另一个抽象类派生一个抽象类
public abstract class FooBase
{
//Base class content
}
public abstract class AnotherBase : FooBase
{
//it is "optional" to make the definition of the abstract methods of the Parent class in here
}
当我们说在子类内部定义父类的抽象方法是可选的时 ,子类应该是abstract是强制性的 。
public abstract class FooBase
{
public abstract string Bar();
public abstract string SomeMethod();
public abstract string OtherMethod();
}
public abstract class AnotherBase : FooBase
{
public override string OtherMethod()
{
//common method that you wanted to use for 6 of your classes
return "special string for the 6 classes";
}
}
//child class that inherits FooBase where none of the method is defined
public class Foo1 : FooBase
{
public override string Bar()
{
//definition
}
public override string SomeMethod()
{
//definition
}
public override string OtherMethod()
{
//definition
}
}
//child class that inherits AnotheBase that defines OtherMethod
public class Another2 : AnotherBase
{
public override string Bar()
{
//definition
}
public override string SomeMethod()
{
//definition
}
}
因此,我猜测还会有5个类,例如Another2
,它从AnotherBase
继承, OtherMethod
定义一个通用的定义
是的,这是完全可能的,而且经常这样做。 没有规则说在类层次结构的最底层只能有一个基类。 该类的子类也可以是抽象的,从而成为间接从您的常规基类派生的一组类的基类(在某种程度上更专门)。
您应该指定这些类的确切功能,但是..鉴于您提供的信息:
正如Head First Design Patterns一书中给出的示例所示,这是Strategy模式旨在解决的确切问题。
您有一个抽象的Duck
类,其他的鸭子(例如RedheadDuck
, MallardDuck
)都派生RedheadDuck
MallardDuck
。 Duck
类具有Quack
方法,该方法仅在屏幕上显示字符串“ quack”。
现在,告诉您添加RubberDuck
。 这个家伙不嘎嘎! 所以你会怎么做? 使Quack
抽象化,让子类决定如何实现这一点? 不,这将导致代码重复。
相反,您可以使用Quack
方法定义IQuackBehaviour
接口。 从那里,您可以导出两个类QuackBehaviour
和SqueakBehaviour
。
public class SqueakBehaviour: IQuackBehaviour
{
public void Quack(){
Console.WriteLine("squeak");
}
}
public class QuackBehaviour: IQuackBehaviour
{
public void Quack(){
Console.WriteLine("quack");
}
}
现在,您可以根据需要使用以下行为来编写鸭子:
public class MallardDuck : Duck
{
private IQuackBehaviour quackBehaviour = new QuackBehaviour();
public override void Quack()
{
quackBehaviour.Quack();
}
}
public class RubberDuck : Duck
{
private IQuackBehaviour quackBehaviour = new SqueakBehaviour();
public override void Quack()
{
quackBehaviour.Quack();
}
}
如果您希望鸭子在运行时更改其行为,甚至可以通过属性注入IQuackBehaviour
的实例。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.