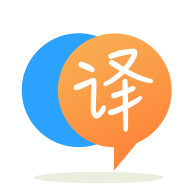
[英]Can abstract class be override in derived class without implementing in base class
[英]Can you override one of the abstract methods in a base class with another base class?
我有一個具有一些抽象方法的基類,並且有21個類從該基類繼承。 現在,對於那些抽象方法之一,我想使用21個類中的6個的通用實現來實現它,因此我考慮創建另一個可以做到這一點的基類。
我樂於接受建議,但我的主要目的是在當前基類和21個類之間創建另一個基類,以防止不必要時在21個類中的6個中重復相同的代碼。
這是代碼示例,說明這種情況:
public abstract class FooBase
{
public abstract string Bar();
public abstract string SomeMethod();
public virtual string OtherMethod()
{
return this.SomeMethod();
}
}
public abstract class AnotherBase : FooBase
{
public abstract string Bar();
public abstract string SomeMethod();
public override OtherMethod()
{
//this is the common method used by 6 of the classes
return "special string for the 6 classes";
}
}
public class Foo1 : FooBase
{
public override string Bar()
{
//do something specific for the Foo1 class here
return "Foo1 special string";
}
public override string SomeMethod()
{
//do something specific for the Foo1 class here
return "Foo1 special string";
}
}
public class Another2 : AnotherBase
{
public override string Bar()
{
//do something specific for the Another2 class here
return "Another special string";
}
public override string SomeMethod()
{
//do something specific for the Another2 class here
return "Another2 special string";
}
}
是的,您可以從另一個抽象類派生一個抽象類
public abstract class FooBase
{
//Base class content
}
public abstract class AnotherBase : FooBase
{
//it is "optional" to make the definition of the abstract methods of the Parent class in here
}
當我們說在子類內部定義父類的抽象方法是可選的時 ,子類應該是abstract是強制性的 。
public abstract class FooBase
{
public abstract string Bar();
public abstract string SomeMethod();
public abstract string OtherMethod();
}
public abstract class AnotherBase : FooBase
{
public override string OtherMethod()
{
//common method that you wanted to use for 6 of your classes
return "special string for the 6 classes";
}
}
//child class that inherits FooBase where none of the method is defined
public class Foo1 : FooBase
{
public override string Bar()
{
//definition
}
public override string SomeMethod()
{
//definition
}
public override string OtherMethod()
{
//definition
}
}
//child class that inherits AnotheBase that defines OtherMethod
public class Another2 : AnotherBase
{
public override string Bar()
{
//definition
}
public override string SomeMethod()
{
//definition
}
}
因此,我猜測還會有5個類,例如Another2
,它從AnotherBase
繼承, OtherMethod
定義一個通用的定義
是的,這是完全可能的,而且經常這樣做。 沒有規則說在類層次結構的最底層只能有一個基類。 該類的子類也可以是抽象的,從而成為間接從您的常規基類派生的一組類的基類(在某種程度上更專門)。
您應該指定這些類的確切功能,但是..鑒於您提供的信息:
正如Head First Design Patterns一書中給出的示例所示,這是Strategy模式旨在解決的確切問題。
您有一個抽象的Duck
類,其他的鴨子(例如RedheadDuck
, MallardDuck
)都派生RedheadDuck
MallardDuck
。 Duck
類具有Quack
方法,該方法僅在屏幕上顯示字符串“ quack”。
現在,告訴您添加RubberDuck
。 這個家伙不嘎嘎! 所以你會怎么做? 使Quack
抽象化,讓子類決定如何實現這一點? 不,這將導致代碼重復。
相反,您可以使用Quack
方法定義IQuackBehaviour
接口。 從那里,您可以導出兩個類QuackBehaviour
和SqueakBehaviour
。
public class SqueakBehaviour: IQuackBehaviour
{
public void Quack(){
Console.WriteLine("squeak");
}
}
public class QuackBehaviour: IQuackBehaviour
{
public void Quack(){
Console.WriteLine("quack");
}
}
現在,您可以根據需要使用以下行為來編寫鴨子:
public class MallardDuck : Duck
{
private IQuackBehaviour quackBehaviour = new QuackBehaviour();
public override void Quack()
{
quackBehaviour.Quack();
}
}
public class RubberDuck : Duck
{
private IQuackBehaviour quackBehaviour = new SqueakBehaviour();
public override void Quack()
{
quackBehaviour.Quack();
}
}
如果您希望鴨子在運行時更改其行為,甚至可以通過屬性注入IQuackBehaviour
的實例。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.