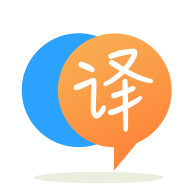
[英]plotting the spectrum of a wavfile in pyqtgraph using scipy.signal.spectrogram
[英]Plotting spectrum of a signal
from numpy.fft import fft
from numpy import array
a = array([1.0, 1.0, 1.0, 1.0, 0.0, 0.0, 0.0, 0.0]
print( ' '.join("%5.3f" % abs(f) for f in fft(a)) )
我正在使用此代码来获取信号的fft,但是如何绘制fft。 谢谢
这是我编写的用于绘制wav
文件的示例。 它稍微复杂一点,因为它通过平均左右声道来处理立体声文件。 并绘制信号和fft。
from __future__ import print_function, division
import wave
import numpy as np
import matplotlib.pyplot as plt
wr = wave.open('input.wav', 'r')
sz = wr.getframerate()
q = 5 # time window to analyze in seconds
c = 12 # number of time windows to process
sf = 1.5 # signal scale factor
for num in range(c):
print('Processing from {} to {} s'.format(num*q, (num+1)*q))
avgf = np.zeros(int(sz/2+1))
snd = np.array([])
# The sound signal for q seconds is concatenated. The fft over that
# period is averaged to average out noise.
for j in range(q):
da = np.fromstring(wr.readframes(sz), dtype=np.int16)
left, right = da[0::2]*sf, da[1::2]*sf
lf, rf = abs(np.fft.rfft(left)), abs(np.fft.rfft(right))
snd = np.concatenate((snd, (left+right)/2))
avgf += (lf+rf)/2
avgf /= q
# Plot both the signal and frequencies.
plt.figure(1)
a = plt.subplot(211) # signal
r = 2**16/2
a.set_ylim([-r, r])
a.set_xlabel('time [s]')
a.set_ylabel('signal [-]')
x = np.arange(44100*q)/44100
plt.plot(x, snd)
b = plt.subplot(212) # frequencies
b.set_xscale('log')
b.set_xlabel('frequency [Hz]')
b.set_ylabel('|amplitude|')
plt.plot(abs(avgf))
plt.savefig('simple{:02d}.png'.format(num))
plt.clf()
以下是它生成的图之一。 由于我自定义了matplotlibrc
因此Colors et cetera与默认设置有所不同。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.