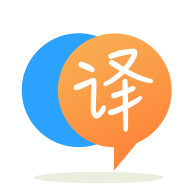
[英]Javascript: convert 24-hour time-of-day string to 12-hour time with AM/PM and no timezone
[英]Display 12-hour and 24-hour time
我想制作一个显示当前时间的网页。 单击“12 小时格式”按钮时,div 区域将显示 12 小时制的时间。 单击“24 小时格式”按钮时,时间将在 div 区域显示为 24 小时制。 当前单击这些按钮时没有任何反应。 帮助!
HTML:
<html>
<head>
<title>Clock</title>
<script type="text/javascript" src="clock.js"></script>
</head>
<body>
<div id="textbox"></div>
<br/>
<button type="radio" onclick="getTwelveHrs()">12 Hour Format</button>
<button type="radio" onclick="getTwentyFourHrs()">24 Hour Format</button>
</body>
</html>
JavaScript:
function getTwelveHours{
if (i < 10) {
i = "0" + i;
}
return i;
}
function startTime() {
var today = new Date();
var h = today.getHours();
var m = today.getMinutes();
var s = today.getSeconds();
m = checkTime(m);
s = checkTime(s);
document.getElementById('textbox').innerHTML = h + ":" + m + ":" + s;
t = setTimeout(function () {
startTime()
}, 500);
}
startTime();
function getTwentyFourHrs() {
var today = new Date();
var h = today.getHours();
var m = today.getMinutes();
var s = today.getSeconds();
m = checkTime(m);
s = checkTime(s);
document.getElementById('textbox').innerHTML = h + ":" + m + ":" + s;
var t = setTimeout(function() {
startTime()
}, 500);
}
function checkTime(i) {
if (i < 10) {
i = "0" + i
};
return i;
}
为什么不使用像 Moment.js 这样的库来为你做这件事。
H, HH 24 hour time
h, or hh 12 hour time (use in conjunction with a or A)
所以在使用 moment.js 时只需在 JavaScript 中使用此代码
moment() 方法以您的特定格式返回当前日期。 因此,当您用户单击按钮时,您可以在每个按钮上调用以下方法
moment().format("YYYY-MM-DD HH:mm"); // 24H clock
moment().format("YYYY-MM-DD hh:mm"); // 12H clock
没有测试过这个,但它应该可以工作
12 小时格式可以通过使用moment js 来获取,这是一个很好的执行时间和日期操作的库。
moment().format('YYYY-MM-DD, hh:mm:ss A')
where Post 或 ante meridiem (注意只有一个字符 ap 也被认为是有效的)
Moment Js 的链接:-
同意其他人的意见,是的,该代码存在问题,但对于时间转换部分 - 也许您可以使用 JavaScript 内置函数执行这样的简单操作:
对于 12 小时格式:
let formattedTime = new Date().toLocaleTimeString('en-US'); console.log(formattedTime)
对于 24 小时格式:
let currentDateTime = new Date(); let formattedTime = currentDateTime.getHours() + ":" + currentDateTime.getMinutes() +":" + currentDateTime.getSeconds(); console.log(formattedTime)
const time = new Date().getHours('en-US',{hour12:false});
const time = new Date().getHours('en-US',{hour12:true});
老实说,我不完全确定您是如何努力实现这一目标的,但我想我理解您想要发生的事情。
试试这个:
window.onload = function() {
var h, m, s;
document.getElementById('twelveHrs').style.display = 'none';
document.getElementById('twentyFourHrs').style.display = 'none';
getTwelveHrs();
getTwentyFourHrs();
function getTwelveHrs() {
var tag = 'AM';
checkTime();
if(h > 12) {
h -= 12
tag = 'PM';
}
document.getElementById('twelveHrs').innerHTML = h + ':' + m + ':' + s + ' ' + tag;
t = setTimeout(function() {
getTwelveHrs()
}, 1000);
}
function getTwentyFourHrs() {
checkTime();
document.getElementById('twentyFourHrs').innerHTML = h + ':' + m + ':' + s;
var t = setTimeout(function() {
getTwentyFourHrs()
}, 1000);
}
function checkTime() {
var today = new Date();
h = today.getHours();
m = today.getMinutes();
s = today.getSeconds();
if(h < 10)
h = '0' + h;
if(m < 10)
m = '0' + m;
if(s < 10)
s = '0' + s;
return h, m, s;
}
}
function displayTwelveHrs() {
document.getElementById('twentyFourHrs').style.display = 'none';
document.getElementById('twelveHrs').style.display = '';
}
function displayTwentyFourHrs() {
document.getElementById('twelveHrs').style.display = 'none';
document.getElementById('twentyFourHrs').style.display = '';
}
然后将您的 HTML 替换为:
<div id="twelveHrs"></div>
<div id="twentyFourHrs"></div>
<br />
<button type="radio" onclick="displayTwelveHrs()">12 Hour Format</button>
<button type="radio" onclick="displayTwentyFourHrs()">24 Hour Format</button>
基本上,当页面加载时,它会启动时钟并隐藏相应的div
标签。 然后你点击一个按钮,它会显示你想要的div
,同时隐藏另一个。
可以在以下位置找到工作的 JSFiddle: http : //jsfiddle.net/fp3Luwzc/
由于工作截止日期即将到来,而且现在真的快到了,我决定回答这个 5 年前的问题。
大多数人推荐使用 Moment.js 库,这真的很好,因为在大多数情况下,重新发明轮子是没有意义的,相信每周有 9,897,199 次 npm 下载的库无疑是一个明智的选择。
但是,由于基于 OP 代码提供解决方案的唯一答案似乎存在一些错误; 我虚心提出我的解决方案:
const FORMATS = { TwelveHours: 12, TwentyFourHours: 24 } class Clock { format = FORMATS.TwentyFourHours; constructor(clockDivId) { this.clockDivId = clockDivId; this.clockInterval = setInterval(() => { document.getElementById(clockDivId).innerHTML = this.getCurrentTime().format(this.format); }, 500) } getCurrentTime() { let today = new Date(); return new Time(today.getHours(), today.getMinutes(), today.getSeconds()); } switchTo12HourFormat() { this.format = FORMATS.TwelveHours } switchTo24HourFormat() { this.format = FORMATS.TwentyFourHours } destroy() { clearInterval(this.clockInterval); } } class Time { constructor(hours, minutes, seconds) { this.hours = hours; this.minutes = minutes; this.seconds = seconds; } format(type) { switch (type) { case FORMATS.TwentyFourHours: { return this.print(this.hours) } case FORMATS.TwelveHours: { let tag = this.hours >= 12 ? 'pm' : 'a.m'; let hours = this.hours % 12; if (hours == 0) { hours = 12; } return this.print(hours) + ' ' + tag; } } } //private to2Digits(number) { return number < 10 ? '0' + number : '' + number; } print(hours) { return this.to2Digits(hours) + ':' + this.to2Digits(this.minutes) + ':' + this.to2Digits(this.seconds); } } let clock = new Clock("clock");
<html> <head> <title>Clock</title> </head> <body> <div id="clock"></div> <br/> <button onclick="clock.switchTo12HourFormat()">12 Hour Format</button> <button onclick="clock.switchTo24HourFormat();">24 Hour Format</button> </body> </html>
哦不,哦不! 我已经写了“print”而不是“this.print”,我已经在谷歌浏览器中运行了它。
基本上 UI 被打印对话框挡住了,我已经丢失了所有代码,不得不重新编写它,现在我要回家睡觉,也许,也许是一集 HIMYM。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.