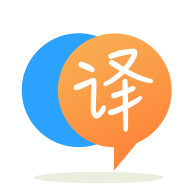
[英]Javascript -- Detect if user's locale are set to use 12-hour or 24-hour time format
[英]Detect if user's locale is set to 12-hour or 24-hour timeformat using javascript
如何使用 Javascript 检查用户是否使用 12 小时或 24 小时时间格式,有或没有使用像 moment.js 这样的第三方库我也尝试new Date().toLocaleString()
但它没有在 firefox 和谷歌浏览器中测试。 firefox 始终显示 12 小时制,而 chrome 始终显示 24 小时制
像这样的东西:
/*
* Detects browser's locale 24h time preference
* It works by checking whether hour output contains a space ('1 AM' or '01')
*/
const isBrowserLocale24h = () =>
!new Intl.DateTimeFormat(undefined, { hour: 'numeric' }).format(0).match(/\s/);
检查Intl.DateTimeFormat 的浏览器兼容性。
您也许可以回退到以下内容,但我不确定AM/PM
指定(无论使用哪个字符)是否位于所有语言环境的输出末尾。 这只是检查最后一个字符是否是数字:
Number.isFinite(Number((new Date()).toLocaleString().slice(-1)))
Mario Bonaci 的回答并没有像我预期的那样奏效,尽管它为我指明了正确的方向。 这是我最初尝试的:
/*
* Detects navigator locale 24h time preference
* It works by checking whether hour output contains AM ('1 AM' or '01 h')
*/
const isBrowserLocale24h = () =>
!new Intl.DateTimeFormat(undefined, { hour: "numeric" })
.format(0)
.match(/AM/);
它没有按预期工作,因为它显然采用浏览器的安装语言而不是用户首选语言。 将undefined
更改为navigator.language
。 在这里,我们根据用户的首选语言检查时间格式:
/*
* Detects navigator locale 24h time preference
* It works by checking whether hour output contains AM ('1 AM' or '01 h')
* based on the user's preferred language
*/
const isBrowserLocale24h = () =>
!new Intl.DateTimeFormat(navigator.language, { hour: "numeric" })
.format(0)
.match(/AM/);
@Marko Bonaci 的回答很有帮助。 我研究了他的评论“我不确定 AM/PM 指定(无论使用哪个字符)是否在所有语言环境的输出末尾。” 谷歌搜索列出了这个链接,说明了用户的评论:
拉丁语缩写 am 和 pm(通常写作“am”和“pm”、“AM”和“PM”或“AM”和“PM”)用于英语、葡萄牙语(巴西)和西班牙语。 希腊语中的等价物是 π.µ。 和µ.µ。 分别。
这可以从浏览器的控制台使用两个字母的ISO 639-1代码“el”或三个字母的 ISO 639-2 代码“ell”来验证:
new Intl.DateTimeFormat(["el"], { hour: "numeric" }).format();
// or
new Intl.DateTimeFormat("ell", { hour: "numeric" }).format();
这些行将返回一个带有本地化“AM”/“PM”字符串的值:
'7 π.μ.'
'7 μ.μ.'
我最终采纳了他的建议,使用了 JavaScript 中的内置“ Number ”对象。
// UK english
Number.isInteger(Number(new Intl.DateTimeFormat("en-UK", { hour: "numeric" }).format()));
// Returns 'true'
// Greek
Number.isInteger(Number(new Intl.DateTimeFormat("el", { hour: "numeric" }).format()));
// Returns 'false'
// U.S.
Number.isInteger(Number(new Intl.DateTimeFormat("en-US", { hour: "numeric" }).format()));
// Returns 'false'
这是我冗长的功能:
const isBrowserLocaleClockType24h = (languages) => {
// "In basic use without specifying a locale, DateTimeFormat
// uses the default locale and default options."
// Ref: developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Intl/DateTimeFormat#using_datetimeformat
// To be sure of the browser's language (set by the user
// and may be different than the operating system's default language)
// set the 'languages' parameter to 'navigator.language'.
// E.g. isBrowserLocaleClockType24h(navigator.language);
if (!languages) { languages = []; }
// The value of 'hr' will be in the format '0', '1', ... up to '24'
// for a 24-hour clock type (depending on a clock type of
// 'h23' or 'h24'. See:
// developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Intl/Locale
// Intl.Locale.prototype.hourCycles
// Returns an Array of hour cycle identifiers, indicating either
// the 12-hour format ("h11", "h12") or
// the 24-hour format ("h23", "h24").
// A 12-hour clock type value has the format '7 AM', '10 PM', or
// '7 π.μ.' (if the locale language is Greek as specified
// by the two letter ISO 639-1 code "el" or
// three letter ISO 639-2 code "ell").
const hr = new Intl.DateTimeFormat(languages, { hour: "numeric" }).format();
// If there's no space in the value of the 'hr' variable then
// the value is a string representing a number between and
// can include '0' and '24'. See comment above regarding "hourCycles".
// Return 'true' if a space exists.
//if (!hr.match(/\s/)) { return true; }
// Or simply:
// return !hr.match(/\s/);
// Alternatively, check if the value of 'hr' is an integer.
// E.g. Number.isInteger(Number('10 AM')) returns 'false'
// E.g. Number.isInteger(Number('7 π.μ.')) returns 'false'
// E.g. Number.isInteger(Number('10')) returns 'true'
return Number.isInteger(Number(hr));
};
用法:
const isBrowserLocaleClock24h = isBrowserLocaleClockType24h();
// or
const isBrowserLocaleClock24h = isBrowserLocaleClockType24h(navigator.language);
最后,对于更新的浏览器(2022 年 12 月的 Firefox 除外), MDN上列出了新的 Intl.Locale(navigator.language).hourCycles。
// developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/some#checking_whether_a_value_exists_in_an_array
const hourCycles = new Intl.Locale(navigator.language).hourCycles;
const isBrowserLocale24h = ["h23", "h24"].some(hourCycle => hourCycles.includes(hourCycle));
Intl.Locale.prototype.hourCycle 属性是一个访问器属性,它返回语言环境使用的计时格式约定。
世界上主要使用两种计时约定(时钟):12 小时制和 24 小时制。 hourCycle 属性使 JavaScript 程序员更容易访问特定区域设置使用的时钟类型。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.