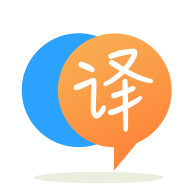
[英]Python Text-Based Game: Guessing Game Using While-Loop and Try-Except
[英]Simple Text-based game using classes. Trying to create a basic loop for if has a particular item in the inventory. Then open the door
我的问题实际上发生在 LockedRoom() 中:
python抛出的错误是:
Traceback (most recent call last):
File "C:\Users\Tank\AppData\Roaming\Microsoft\Windows\Start Menu\Programs\Enthought\CastleGame1.py", line 479, in <module>
a_game.play()
File "C:\Users\Tank\AppData\Roaming\Microsoft\Windows\Start Menu\Programs\Enthought\CastleGame1.py", line 33, in play
next_scene_name = current_scene.enter()
File "C:\Users\Tank\AppData\Roaming\Microsoft\Windows\Start Menu\Programs\Enthought\CastleGame1.py", line 147, in enter
has_key = "key" in Person.items
AttributeError: class Person has no attribute 'items'
因此,我认为问题在于检查或将“钥匙”放入该人的物品中。 然后我想检查这个列表,看看战士是否携带它。 如果他是,那么他应该能够打开门。 如果没有,那么门应该保持关闭。 我需要在 TowerRoom 的最后再重复一遍。 在这种情况下,我需要检查最多 3 个不同的项目。 我附上了代码。 希望这个错误是一个非常简单的错误。
from sys import exit
from random import randint
key = False
brick = False
candle = False
Cup = False
class Scene(object):
def enter(self):
print "This scene is not yet configured."
exit(1)
class Person:
def __init__(self, name):
self.name = name
self.items = []
class Engine(object):
def __init__(self, scene_map):
self.scene_map = scene_map
def play(self):
current_scene = self.scene_map.opening_scene()
last_scene = self.scene_map.next_scene('finished')
while current_scene != last_scene:
next_scene_name = current_scene.enter()
current_scene = self.scene_map.next_scene(next_scene_name)
current_scene.enter()
class Death(Scene):
quips =[
"You really suck at this.",
"Why don't you try a little harder next time.",
"Well... that was a very stupid thing to do.",
"I have a small puppy that's better at this than you.",
"why don't you just give up now.",
"Please, stop killing me!",
"Your mom would be proud of you... if she were smarter."
]
def enter(self):
print Death.quips[randint(0, len(self.quips)-1)]
exit(0)
class MainGate(Scene):
def enter(self):
print "Welcome to Luke's first Text based game."
print "It currently has no name, nor will it ever have one."
print "Because it kind of sucks a little bit."
print"----------------------------------------"
print "Evil Dr. Onion has stollen the Mushroom Kingdoms princess"
print "You have chased him down for months through rain, snow"
print "and desserts. You have finally tracked him down."
print "In front of you is a huge castle. You go through the giant"
print "entrance to begin your search for the princess. Nobody"
print "know what may await you ahead."
print "Do you want to enter the Castle?"
print "1. Yes"
print "2. No"
action = raw_input(">")
if action == '1':
return 'central_corridor'
elif action == '2':
print "What? You are leaving and giving up on the princess"
print "already? God will certainly smite you for this!!!"
return 'death'
else:
print "Please choose an action numerically using the numpad"
print "on your computer."
return 'MainGate'
class CentralCorridor(Scene):
def enter(self):
print "You enter the castle. In fron of you stands a giant onion"
print "monster. It looks at you with its big, bulging eye. Your"
print "own eyes begin to weep as you look at this... thing."
print "The giant onion monster begins to charge at you, waving its"
print "sword over what I suppose would be its head."
print "what do you do?"
print "1. Try and fight the giant onion with your sword."
print "2. Try and dodge out of the way of the onion and run past it."
print "3. Throw something at the onion."
print "4. Stand there and scream at the onion."
action = raw_input(">")
if action == '1':
print "You take your sword out of its sheath. Waiting for the onion"
print "to reach you so that you can stab it in its giant eye."
print "however as it gets closer its stench gets worse and worse."
print "It gets so bad that you can hardly see!"
print "You try and swing at the onion, and miss!"
print "You feel a stabbing pain in your right shoulder"
print "and then everything goes black."
return 'death'
elif action == '2':
print "As the onion charges you, you jump out of the way."
print "However, the giant onion is much quicker than you expected."
print "You look down to see a sword protruding from your stomach."
print "You scream in pain and die."
return 'death'
elif action == '3':
print "You look in your pockets to find something to throw at"
print "the onion."
print "You find a rubber duck and throw it at the onion. Unfortunately, it"
print "does nothing but distracts the onion from you for a few"
print "short seconds. After it focuses its attention back on you,"
print "it eats your face off."
return 'death'
elif action == '4':
print "You choose to stand and scream at the onion at the highest"
print "pitch your girly little voice will allow."
print "The onion, hearing your scream, is terrified. It turns"
print "around and rolls away as quickly as its little legs and can"
print "carry it. You quickly catch up with it and cut its little"
print "legs right off, before hurrying to the next room."
return 'locked_room'
else:
print "please choose an appropriate action dear sir. Otherwise,"
print "you will never be able to rescue the princess."
return 'central_corridor'
class LockedRoom(Scene):
def enter(self):
print "You are standing in a room with just a door in front of you."
print "What do you do?"
print "1. Try and open the door."
has_key = "key" in Person.items
if not has_key: print "2. pick up the key"
action = raw_input(">")
if (action == '1' and has_key):
print "You unlock and open the door."
return 'monty_hall_room'
elif (action == '1' and not has_key):
print "the door will not budge"
return 'locked_room'
elif (action == '2' and not has_key):
print "you picked up the key."
Person.items.append("key")
return 'locked_room'
else:
print "Please enter a numeric value as something to do."
print "Otherwise we might be here all day."
return 'locked_room'
class Finished(Scene):
def enter(self):
print "You won! Good job."
return 'finished'
class Map(object):
scenes = {
'main_gate': MainGate(),
'central_corridor': CentralCorridor(),
'locked_room': LockedRoom(),
'monty_hall_room': MontyHallRoom(),
'pickel_room': PickleRoom(),
'death': Death(),
'finished': Finished(),
}
def __init__(self, start_scene):
self.start_scene = start_scene
def next_scene(self, scene_name):
val = Map.scenes.get(scene_name)
return val
def opening_scene(self):
return self.next_scene(self.start_scene)
warrior = Person("Soldat")
a_map = Map('central_corridor')
a_game = Engine(a_map)
a_game.play()
我只需要一种方法来使用类“列表”并将东西放入和取出其他类中。 然后,使用该列表中包含的任何内容打开门或杀死怪物。
任何人都可以提供的任何帮助将不胜感激。
您需要创建一个“人”实例(您已经这样做了),而不是使用类:
warrior = Person.new("penne12")
或者使用你创建的
然后你可以使用你的“战士”:
warrior.name #=> "penne12"
warrior.items #=> []
这是因为类本身不存储名称或项目,类的实例(在您执行Person.new
时创建)包含项目数组和名称。 试图获取类的属性是行不通的。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.