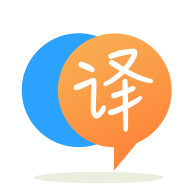
[英]Count different non-zero values of two 2D arrays in Python numpy
[英]How do I compare non-zero values over multiple 2D numpy arrays?
假设我有两个2D数组,其中填充了随机整数。 我希望第一个数组保留所有值为leq 5的索引,第二个数组保留所有值为leq 6的索引。为简单起见,可以将所有其他索引设置为零。
table1 = np.array(([1,3],[5,7]))
table2 = np.array(([2,4],[6,8]))
print table1
print table2 ,'\n'
table1_tol_i = np.where(table1[:,:] > 5)
table1[table1_tol_i] = 0
print table1
table2_tol_i = np.where(table2[:,:] > 4)
table2[table2_tol_i] = 0
print table2, '\n'
[[1 3]
[5 7]]
[[2 4]
[6 8]]
[[1 3]
[5 0]]
[[2 4]
[0 0]]
然后,我只想保留两个表都具有非零值的索引。 我想要
[[1 3]
[0 0]]
[[2 4]
[0 0]]
我尝试了以下方法,但没有给我想要的东西
nonzero_i = (np.where(table1 != 0) and (np.where(table2 != 0 )))
print table1[nonzero_i]
print table2[nonzero_i]
[1 3]
[2 4]
您只保留两个表都具有非零值的索引-这就是为什么当您使用nonzero_i
索引table1
和table2
时,仅返回第一行(仅包含非零值)的原因。
通过构造布尔索引数组,可以更简单地表达这种逻辑:
# boolean array that is True wherever both table1 & table2 are nonzero
valid = (table1 != 0) & (table2 != 0)
# or alternatively:
# valid = np.logical_and(table1, table2)
您可以使用它直接索引到table1
和table2
。
似乎您实际上想要执行的操作是将table1
或table2
为0的任何位置赋值为0。
如果你想要一个副本,您可以使用np.where(condition, val_where_true, val_where_false)
的语法np.where
:
print(repr(np.where(valid, table1, 0)))
# array([[1, 3],
# [0, 0]])
print(repr(np.where(valid, table2, 0)))
# array([[2, 4],
# [0, 0]])
或者,如果您想修改table1
和table2
,则可以执行
table1[~valid] = 0
table2[~valid] = 0
( ~
是“按位非”运算符,它会反转布尔索引数组)。
获取表1为0的所有索引:
table1_zeros = np.where(table1[:,:] == 0)
获取表2为0的所有索引:
table2_zeros = np.where(table2[:,:] == 0)
将两个表的值都设置为0:
table1[table1_zeros] = 0
table1[table2_zeros] = 0
table2[table1_zeros] = 0
table2[table2_zeros] = 0
您也可以使用tableX_tol_i
代替tableX_zeros
一步来完成此tableX_zeros
。
table1 = np.array(([1,3],[5,7]))
table2 = np.array(([2,4],[6,8]))
table3 = np.zeros((2,2))
for k in range(0,2):
for j in range(0,2):
if table1[k][j] > 5:
table1[k][j] = 0
if table1[k][j] <= 5:
table1[k][j] = table1[k][j]
if table2[k][j] > 6:
table2[k][j] = 0
if table2[k][j] <= 6:
table2[k][j] = table2[k][j]
x=0
y=0
for k in range(0,2):
for j in range(0,2):
if table1[k][j] != 0:
table3[x,y] = table1[k][j]
x = x+1
x=0
y=1
for k in range(0,2):
for j in range(0,2):
if table2[k][j] != 0:
table3[x,y] = table2[k][j]
x = x+1
print (table3)
我认为这是最简单的方法,您想要什么。 如果阵列大小更改,则可以修改代码。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.