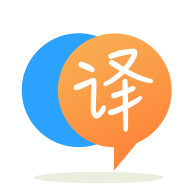
[英]Is dispatch_async(dispatch_get_main_queue(), …) necessary in this case?
[英]Why does the block after dispatch_async(dispatch_get_main_queue() never get called?
我想异步执行一些代码,因此,已经开始将GCD用于OSX / iOS。
当前,我正在使用函数dispatch_async() 。
当我想在另一个线程上同时执行某些操作时,可以使用函数dispatch_get_global_queue() 。
当我想将结果分派到主线程时,我使用了函数dispatch_get_main_queue() 。
但是结果永远不会到达主线程。
在调试器中(在dispatch_async行上)设置断点时,函数dispatch_async(dispatch_get_global_queue(DISPATCH_QUEUE_PRIORITY_DEFAULT,0)之后的块有时仅执行,但调试器通常会忽略它。
当该块确实被执行并且执行流到达dispatch_async(dispatch_get_main_queue()处的断点时,此后的块总是被忽略。
如果没有dispatch_async(dispatch_get_global_queue)和dispatch_async(dispatch_get_main_queue),则代码将按预期执行,即使是同步执行。
我的问题是,为什么dispatch_async(dispatch_get_main_queue()的dispatch_async(dispatch_get_global_queue内()块从未得到执行?
同时,为什么每次都不会执行dispatch_async(dispatch_get_global_queue()块) ?
我的开发环境是
作业系统: OS X 10.11.3
IDE: Xcode 7.2
编译器: Apple LLVM版本7.0.2(clang-700.1.81)
目标: x86_64-apple-darwin15.3.0
这是一个重现不稳定行为的简单示例(OS X控制台应用程序):
TestClass.h
#ifndef TestClass_h
#define TestClass_h
@interface TestClass : NSObject {
}
- (void)testMethod:(NSString *)testString withCompletionBlock:(void(^)(NSString *blockResult, NSError __autoreleasing *error))completionBlock;
@end
#endif
TestClass.m
#import <Foundation/Foundation.h>
#import "TestClass.h"
@implementation TestClass
- (void)testMethod:(NSString *)testString withCompletionBlock:(void(^)(NSString *blockResult, NSError __autoreleasing *error))completionBlock {
__block NSString *stringResult = nil;
if (completionBlock) {
__block NSError *resultError = nil;
dispatch_async(dispatch_get_global_queue(DISPATCH_QUEUE_PRIORITY_DEFAULT, 0), ^{
// THIS BLOCK IS CALLED ONLY SOMETIMES, MOST OF THE TIME IT IS IGNORED.
if ([testString isEqual: @"Error string"]) {
NSDictionary *errorUserInfo = @{ NSLocalizedDescriptionKey: @"This is an error.", NSLocalizedFailureReasonErrorKey: @"", NSLocalizedRecoverySuggestionErrorKey: @"" };
resultError = [[NSError alloc] initWithDomain:@"com.test.TestErrorDomain" code:10 userInfo:errorUserInfo];
}
else {
stringResult = testString;
}
dispatch_async(dispatch_get_main_queue(), ^{
// THIS BLOCK NEVER GETS EXECUTED.
completionBlock(stringResult, resultError);
});
});
}
}
@end
的main.m
#import <Foundation/Foundation.h>
#import "TestClass.h"
int main(int argc, const char * argv[]) {
@autoreleasepool {
__block NSString *resultString = nil;
TestClass * testObject = [[TestClass alloc] init];
// Output for this call should be: The result string is: Test string.
[testObject testMethod:@"Test string" withCompletionBlock:^(NSString *blockString, NSError __autoreleasing *error) {
resultString = blockString;
if (resultString) {
NSLog(@"The result string is: %@.", resultString);
}
}];
// Output for this call should be: Error: This is an error.
[testObject testMethod:@"Error string" withCompletionBlock:^(NSString *blockString, NSError __autoreleasing *error) {
resultString = blockString;
if (!resultString) {
if (error) {
NSLog(@"Error: %@", [error localizedDescription]);
}
else {
NSLog(@"Error not recognized!");
}
}
}];
}
return 0;
}
这是因为一旦main()函数退出,您的TestClass对象就会被释放。 主要是异步的,testMethod:不会阻塞main()中的指针。
尝试在main()的末尾添加一个信号量。 该信号量应在testMethod dispatch_async(dispatch_get_main_queue(), ^{});
发出信号dispatch_async(dispatch_get_main_queue(), ^{});
块。
这是带有信号量的工作示例,如@CharlesThierry的答案所建议。 我还包含了一些有助于说明执行流程的NSLogs:
TestClass.h
#ifndef TestClass_h
#define TestClass_h
@interface TestClass : NSObject {
}
- (void)testMethod:(NSString *)testString withSemaphore:(dispatch_semaphore_t)sem withCompletionBlock:(void(^)(NSString *blockResult, NSError __autoreleasing *error))completionBlock;
@end
#endif
TestClass.m
#import <Foundation/Foundation.h>
#import "TestClass.h"
@implementation TestClass
- (void)testMethod:(NSString *)testString withSemaphore:(dispatch_semaphore_t)sem withCompletionBlock:(void(^)(NSString *blockResult, NSError __autoreleasing *error))completionBlock {
__block NSString *stringResult = nil;
if (completionBlock) {
__block NSError *resultError = nil;
NSLog(@"INSIDE TEST METHOD, OUTSIDE DISPATCH ASYNC");
dispatch_async(dispatch_get_global_queue(DISPATCH_QUEUE_PRIORITY_DEFAULT, 0), ^{
NSLog(@"INSIDE THE DISPATCH ASYNC TO THE GLOBAL QUEUE");
if ([testString isEqual: @"Error string"]) {
NSDictionary *errorUserInfo = @{ NSLocalizedDescriptionKey: @"This is an error.", NSLocalizedFailureReasonErrorKey: @"", NSLocalizedRecoverySuggestionErrorKey: @"" };
resultError = [[NSError alloc] initWithDomain:@"com.test.TestErrorDomain" code:10 userInfo:errorUserInfo];
}
else {
stringResult = testString;
}
dispatch_async(dispatch_get_main_queue(), ^{
NSLog(@"INSIDE THE DISPATCH ASYNC TO THE MAIN QUEUE!");
completionBlock(stringResult, resultError);
dispatch_semaphore_signal(sem);
});
});
}
}
@end
的main.m
#import <Foundation/Foundation.h>
#import "TestClass.h"
int main(int argc, const char * argv[]) {
@autoreleasepool {
NSLog(@"BEFORE BLOCK");
__block NSString *resultString = nil;
TestClass * testObject = [[TestClass alloc] init];
dispatch_semaphore_t sem = dispatch_semaphore_create(0);
[testObject testMethod:@"Test string" withSemaphore:sem withCompletionBlock:^(NSString *blockString, NSError __autoreleasing *error)
{
NSLog(@"INSIDE FIRST BLOCK");
resultString = blockString;
if (resultString)
{
NSLog(@"The result string is: %@.", resultString);
}
}];
[testObject testMethod:@"Error string" withSemaphore:sem withCompletionBlock:^(NSString *blockString, NSError __autoreleasing *error)
{
NSLog(@"INSIDE SECOND BLOCK");
resultString = blockString;
if (!resultString)
{
if (error)
{
NSLog(@"Error: %@", [error localizedDescription]);
}
else
{
NSLog(@"Error not recognized!");
}
}
}];
NSLog(@"AFTER BLOCK!");
while (dispatch_semaphore_wait(sem, DISPATCH_TIME_NOW))
[[NSRunLoop currentRunLoop] runMode:NSDefaultRunLoopMode beforeDate:[NSDate dateWithTimeIntervalSinceNow:10]];
}
return 0;
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.