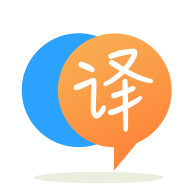
[英]how can I subtract the amount Randint generates in the while loop from enemies HP without getting 2 different numbers?
[英]How can I get new results from randint while in a loop?
到目前为止,我的代码:
from random import randint
Dice1 = randint(1,6)
Dice2 = randint(1,6)
Dice3 = randint(1,6)
DiceRoll2 = Dice1 + Dice2
DiceRoll3 = Dice1 + Dice2 + Dice3
class Item(object):
def __init__(self, name, value, desc):
self.name = name
self.value = value
self.desc = desc
sword = Item("Sword", 2, "A regular sword.")
class Monster(object):
def __init__(self, name, health, attack):
self.name = name
self.health = health
self.attack = attack
monster = Monster("Monster", 50, DiceRoll2)
Damage = DiceRoll3 + sword.value
NewHealth = monster.health - Damage
print("You see a monster!")
while True:
action = input("? ").lower().split()
if action[0] == "attack":
print("You swing your", sword.name, "for", Damage, "damage!")
print("The", monster.name, "is now at", NewHealth, "HP!")
elif action[0] == "exit":
break
意图是,每次输入“攻击”都会得到DiceRoll3的随机结果(从1到6的三个随机数,即3次),加上剑的值,并从怪物的初始生命值中减去该值。 这很好,直到我第二次输入“攻击”为止,这会导致相同的损坏和减少的运行状况打印,而不是使用新的值。 我该怎么做呢?
将掷骰子放入一个单独的函数中,并在循环内计算Damage
和NewHealth
。 (另外,更新怪物的健康。:))
from random import randint
def dice_roll(times):
sum = 0
for i in range(times):
sum += randint(1, 6)
return sum
class Item(object):
def __init__(self, name, value, desc):
self.name = name
self.value = value
self.desc = desc
sword = Item("Sword", 2, "A regular sword.")
class Monster(object):
def __init__(self, name, health, attack):
self.name = name
self.health = health
self.attack = attack
monster = Monster("Monster", 50, dice_roll(2))
print("You see a monster!")
while True:
action = input("? ").lower().split()
if action[0] == "attack":
Damage = dice_roll(3) + sword.value
NewHealth = max(0, monster.health - Damage) #prevent a negative health value
monster.health = NewHealth #save the new health value
print("You swing your", sword.name, "for", Damage, "damage!")
print("The", monster.name, "is now at", NewHealth, "HP!")
elif action[0] == "exit":
break
if monster.health < 1:
print("You win!")
break
您需要每次都致电randint。 以下是一种方法
def Dice1(): return randint(1,6)
def Dice2(): return randint(1,6)
def Dice3(): return randint(1,6)
但是由于所有重复的代码,这并不是很好。 这是一个更好的方法
class Dice(object):
def roll_dice(self):
return randint(1,6)
Dice1 = Dice()
Dice2 = Dice()
Dice3 = Dice()
现在在您的代码中,无论您在哪里调用Dice1
, Dice2
或Dice3
; 而是调用Dice1.roll_dice()
, Dice2.roll_dice()
, Dice3.roll_dice()
。 这样就抽象了骰子实现,您以后可以随意更改它而不必更改代码。
如果您需要具有不同面数的骰子,则只需更改骰子类别
class Dice(object):
def __init__ (self, num_faces=6):
self.faces = num_faces
def roll_dice(self):
return randint(1,self.faces)
Dice1 = Dice() # 6 faced die
Dice2 = Dice(20) # 20 faced die
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.