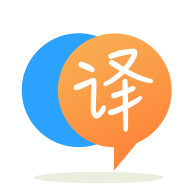
[英]Mismatched types when building a std::process::Command in a loop
[英]Mismatched types when displaying a matrix with a for loop
受到evilone在帖子中提供的代码的启发如何打印Vec? 。 为了显示矩阵,我编写了如下代码:
use std::{ops, fmt};
#[derive(PartialEq, Debug)]
pub struct Matrix<T> {
data: Vec<T>,
row: usize,
col: usize,
}
impl<T: Copy> Matrix<T> {
pub fn new(row: usize, col: usize, values: &[T]) -> Matrix<T> {
Matrix {
data: values.to_vec(),
row: row,
col: col,
}
}
}
//// Display
impl<T: fmt::Display> fmt::Display for Matrix<T> {
fn fmt(&self, f: &mut fmt::Formatter) -> fmt::Result {
let n_row = self.row;
let n_col = self.col;
let data = self.data;
for i in 0.. n_row {
let mut each_row = String::new();
for j in 0.. n_col {
let idx = i * n_col + j;
let each_element = data[idx];
each_row.push_str(&each_element.to_string());
each_row.push_str(" "); // seperated by space
}
write!(f, "{}", each_row)
}
}
}
fn main() {
let x = Matrix::new(2, 3, &[-6, -5, 0, 1, 2, 3]);
println!("{}", x);
}
我得到了错误:
rustc 1.13.0 (2c6933acc 2016-11-07)
error[E0308]: mismatched types
--> <anon>:40:13
|
40 | write!(f, "{}", each_row)
| ^^^^^^^^^^^^^^^^^^^^^^^^^ expected (), found enum `std::result::Result`
|
= note: expected type `()`
= note: found type `std::result::Result<(), std::fmt::Error>`
= note: this error originates in a macro outside of the current crate
error[E0308]: mismatched types
--> <anon>:31:9
|
31 | for i in 0.. n_row {
| ^ expected enum `std::result::Result`, found ()
|
= note: expected type `std::result::Result<(), std::fmt::Error>`
= note: found type `()`
1)我不明白为什么我会expected (), found enum `std::result::Result`
2)对于第二个错误,我认为这是由于未能实施第40行引起的。因此,如果修复第40行,将不再是问题。
有什么建议可以解决这个问题吗?
进行编程时,创建一个最小,完整和可验证的示例很有用。 这意味着您删除了与您所遇到的错误或问题无关的所有内容,并将其简化为纯粹的本质。 通常,您会发现这样做可以大大缩小问题的潜在范围。 通常,这可以让您自己回答问题,而有时,它增加了提出具有深刻见解的问题的机会。
这是针对此问题的MCVE:
fn foo() -> u8 {
for i in 0..1u8 {
i
}
}
fn main() {}
很小,不是吗? 它产生以下错误:
error[E0308]: mismatched types
--> src/main.rs:3:9
|
3 | i
| ^ expected (), found u8
|
= note: expected type `()`
= note: found type `u8`
error[E0308]: mismatched types
--> src/main.rs:2:5
|
2 | for i in 0..1u8 {
| ^ expected u8, found ()
|
= note: expected type `u8`
= note: found type `()`
看起来应该很熟悉。
现在您可以问自己一个问题: for
循环求值的类型和值是什么? (提示:编译器消息实际上告诉您是否以正确的方式阅读它们)
我们知道函数必须返回u8
,但是编译器告诉我们实际上正在返回()
-这是第二个错误。 这意味着for
循环的计算结果为()
! 由于for
循环的计算结果为()
,因此for
循环的块计算结果的值可能会发生什么? 如您所料,答案是该块无法返回值!
想想这个例子:
fn foo() -> u8 {
for i in 0..0u8 {
//
}
}
什么会这回? 是的,可能没什么好处。
从我们的MCVE回到原始问题,您需要显式返回内部故障,并在循环结束时显式返回成功:
for /* ... */ {
// ...
try!(write!(f, "{}", each_row))
}
Ok(())
这只是打开代码中其他错误的大门,但是您可以找出这些错误!
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.