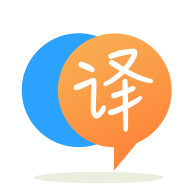
[英]Python input validation: how to limit user input to a specific range of integers?
[英]How do I limit user input to specific integers, and keep track of exceptions, in Python 2.7?
编辑:建议的重复对于基本输入验证非常有用。 尽管确实涵盖了很多内容,但我的特定问题(未能将int(evaluation)
分配给变量)仅在此处明确解决。 如果其他人犯了类似的愚蠢错误,则我将单独标记它:)
在过去的几周中,我一直在使用Python 2.7玩耍,并且玩得很开心。 要了解有关while
循环的更多信息,我创建了一个小脚本,该脚本要求用户输入1到10之间的整数。
我的目标是能够响应用户使用意外输入(例如非整数或超出指定范围的整数)做出响应的情况。 在其他StackOverflow线程的帮助下,我已经能够解决很多问题,但是现在我很困惑。
首先,我创建了一个变量idiocy
,以跟踪异常。 the one it's making fun of.) (该脚本本来应该很活泼,但是直到我能正常工作为止, 那个正在取笑的人。)
idiocy = 0
while 1:
evaluation = raw_input("> ")
try:
int(evaluation)
if evaluation < 1 or evaluation > 10:
raise AssertionError
except ValueError:
idiocy += 1
print "\nEnter an INTEGER, dirtbag.\n"
except AssertionError:
idiocy += 1
print "\nI said between 1 and 10, moron.\n"
else:
if idiocy == 0:
print "\nOkay, processing..."
else:
print "\nDid we finally figure out how to follow instructions?"
print "Okay, processing..."
break
如您所见,我正在尝试处理两个不同的错误-输入类型的ValueError
和整数范围的AssertionError
并跟踪它们引发了多少次。 (真的,我只关心知道它们是否至少被提升过一次;这就是我侮辱用户的全部。)
无论如何,当我以当前形式运行脚本时,错误响应就可以正常工作(对于非整数,“ dirtbag”为“ dirtbag”,对于超出范围,则为“ moron”)。 问题是,即使我输入了有效的整数,我仍然会收到超出范围的AssertionError
。
我怀疑我的问题与我的while
逻辑有关,但我不确定该怎么做。 我在这里或那里添加了一个break
,但这似乎无济于事。 有什么建议或明显的错误吗? 同样,这里是Python的新手,所以我将其付诸实践。
//如果有人有更简单,更清洁或更漂亮的方法来进行此操作,请随时告诉我。 我在这里学习!
你的问题是你不保存int
版本evaluation
,以evaluation
是这样的:
idiocy = 0
while 1:
evaluation = raw_input("> ")
try:
evaluation = int(evaluation) <--- here
if evaluation < 1 or evaluation > 10:
raise AssertionError
except ValueError:
idiocy += 1
print "\nEnter an INTEGER, dirtbag.\n"
except AssertionError:
idiocy += 1
print "\nI said between 1 and 10, moron.\n"
else:
if idiocy == 0:
print "\nDid we finally figure out how to follow instructions?"
print "Okay, processing..."
else:
print "\nOkay, processing..."
如果要跟踪引发的异常类型,则可以使用collections.Counter
进行idiocy
并更改如下代码:
from collections import Counter
idiocy = Counter()
while 1:
evaluation = raw_input("> ")
try:
evaluation = int(evaluation)
if evaluation < 1 or evaluation > 10:
raise AssertionError
except ValueError as e:
idiocy[e.__class__] += 1
print "\nEnter an INTEGER, dirtbag.\n"
except AssertionError as e:
idiocy[e.__class__] += 1
print "\nI said between 1 and 10, moron.\n"
else:
if idiocy == 0:
print "\nDid we finally figure out how to follow instructions?"
print "Okay, processing..."
else:
print "\nOkay, processing..."
>>> idiocy
Counter({AssertionError: 2, ValueError: 3})
您可以通过idiocy[AssertionError]
类的键来访问错误计数
您有int(evalutation)
,但没有将其分配给任何东西。
尝试
try:
evaluation = int(evaluation)
assert 0 < evaluation < 10
except ValueError:
...
except AssertionError:
您的范围测试可以重构为
assert 1 <= evaluation <= 10
你可以把侮辱记在字典里
insults = {AssertionError : "\nI said between 1 and 10, moron.\n",
ValueError : "\nEnter an INTEGER, dirtbag.\n"
}
然后像这样写try / except
try:
...
except (AssertionError, ValueError) as e:
print(insults[type(e)])
将用户输入更改为int时,需要将其分配给某些内容
evaluation = int(evaluation)
assert是用于调试的-您未正确使用它。
在您的代码中: int(evaluation)
不是将评估变量类型转换为int类型。 输出为:
> 2
<type 'str'>
I said between 1 and 10, moron.
尝试这个:
idiocy = 0
while 1:
try:
evaluation = int(raw_input("> "))
if evaluation < 1 or evaluation > 10:
raise AssertionError
except ValueError:
idiocy += 1
print "\nEnter an INTEGER, dirtbag.\n"
except AssertionError:
idiocy += 1
print "\nI said between 1 and 10, moron.\n"
else:
if idiocy == 0:
print "\nOkay, processing..."
else:
print "\nDid we finally figure out how to follow instructions?"
print "Okay, processing..."
break
顺便说一下,您可以使用元组来存储所有异常。 例:
idiocy = 0
all_exceptions = (ValueError, AssertionError)
while 1:
try:
evaluation = int(raw_input("> "))
if evaluation < 1 or evaluation > 10:
raise AssertionError("\nI said between 1 and 10, moron.\n")
except all_exceptions as e:
idiocy += 1
print str(e)
else:
if idiocy == 0:
print "\nOkay, processing..."
else:
print "\nDid we finally figure out how to follow instructions?"
print "Okay, processing..."
break
希望能帮助到你。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.