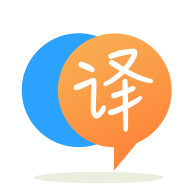
[英]Python input validation: how to limit user input to a specific range of integers?
[英]How do I limit user input to specific integers, and keep track of exceptions, in Python 2.7?
編輯:建議的重復對於基本輸入驗證非常有用。 盡管確實涵蓋了很多內容,但我的特定問題(未能將int(evaluation)
分配給變量)僅在此處明確解決。 如果其他人犯了類似的愚蠢錯誤,則我將單獨標記它:)
在過去的幾周中,我一直在使用Python 2.7玩耍,並且玩得很開心。 要了解有關while
循環的更多信息,我創建了一個小腳本,該腳本要求用戶輸入1到10之間的整數。
我的目標是能夠響應用戶使用意外輸入(例如非整數或超出指定范圍的整數)做出響應的情況。 在其他StackOverflow線程的幫助下,我已經能夠解決很多問題,但是現在我很困惑。
首先,我創建了一個變量idiocy
,以跟蹤異常。 the one it's making fun of.) (該腳本本來應該很活潑,但是直到我能正常工作為止, 那個正在取笑的人。)
idiocy = 0
while 1:
evaluation = raw_input("> ")
try:
int(evaluation)
if evaluation < 1 or evaluation > 10:
raise AssertionError
except ValueError:
idiocy += 1
print "\nEnter an INTEGER, dirtbag.\n"
except AssertionError:
idiocy += 1
print "\nI said between 1 and 10, moron.\n"
else:
if idiocy == 0:
print "\nOkay, processing..."
else:
print "\nDid we finally figure out how to follow instructions?"
print "Okay, processing..."
break
如您所見,我正在嘗試處理兩個不同的錯誤-輸入類型的ValueError
和整數范圍的AssertionError
並跟蹤它們引發了多少次。 (真的,我只關心知道它們是否至少被提升過一次;這就是我侮辱用戶的全部。)
無論如何,當我以當前形式運行腳本時,錯誤響應就可以正常工作(對於非整數,“ dirtbag”為“ dirtbag”,對於超出范圍,則為“ moron”)。 問題是,即使我輸入了有效的整數,我仍然會收到超出范圍的AssertionError
。
我懷疑我的問題與我的while
邏輯有關,但我不確定該怎么做。 我在這里或那里添加了一個break
,但這似乎無濟於事。 有什么建議或明顯的錯誤嗎? 同樣,這里是Python的新手,所以我將其付諸實踐。
//如果有人有更簡單,更清潔或更漂亮的方法來進行此操作,請隨時告訴我。 我在這里學習!
你的問題是你不保存int
版本evaluation
,以evaluation
是這樣的:
idiocy = 0
while 1:
evaluation = raw_input("> ")
try:
evaluation = int(evaluation) <--- here
if evaluation < 1 or evaluation > 10:
raise AssertionError
except ValueError:
idiocy += 1
print "\nEnter an INTEGER, dirtbag.\n"
except AssertionError:
idiocy += 1
print "\nI said between 1 and 10, moron.\n"
else:
if idiocy == 0:
print "\nDid we finally figure out how to follow instructions?"
print "Okay, processing..."
else:
print "\nOkay, processing..."
如果要跟蹤引發的異常類型,則可以使用collections.Counter
進行idiocy
並更改如下代碼:
from collections import Counter
idiocy = Counter()
while 1:
evaluation = raw_input("> ")
try:
evaluation = int(evaluation)
if evaluation < 1 or evaluation > 10:
raise AssertionError
except ValueError as e:
idiocy[e.__class__] += 1
print "\nEnter an INTEGER, dirtbag.\n"
except AssertionError as e:
idiocy[e.__class__] += 1
print "\nI said between 1 and 10, moron.\n"
else:
if idiocy == 0:
print "\nDid we finally figure out how to follow instructions?"
print "Okay, processing..."
else:
print "\nOkay, processing..."
>>> idiocy
Counter({AssertionError: 2, ValueError: 3})
您可以通過idiocy[AssertionError]
類的鍵來訪問錯誤計數
您有int(evalutation)
,但沒有將其分配給任何東西。
嘗試
try:
evaluation = int(evaluation)
assert 0 < evaluation < 10
except ValueError:
...
except AssertionError:
您的范圍測試可以重構為
assert 1 <= evaluation <= 10
你可以把侮辱記在字典里
insults = {AssertionError : "\nI said between 1 and 10, moron.\n",
ValueError : "\nEnter an INTEGER, dirtbag.\n"
}
然后像這樣寫try / except
try:
...
except (AssertionError, ValueError) as e:
print(insults[type(e)])
將用戶輸入更改為int時,需要將其分配給某些內容
evaluation = int(evaluation)
assert是用於調試的-您未正確使用它。
在您的代碼中: int(evaluation)
不是將評估變量類型轉換為int類型。 輸出為:
> 2
<type 'str'>
I said between 1 and 10, moron.
嘗試這個:
idiocy = 0
while 1:
try:
evaluation = int(raw_input("> "))
if evaluation < 1 or evaluation > 10:
raise AssertionError
except ValueError:
idiocy += 1
print "\nEnter an INTEGER, dirtbag.\n"
except AssertionError:
idiocy += 1
print "\nI said between 1 and 10, moron.\n"
else:
if idiocy == 0:
print "\nOkay, processing..."
else:
print "\nDid we finally figure out how to follow instructions?"
print "Okay, processing..."
break
順便說一下,您可以使用元組來存儲所有異常。 例:
idiocy = 0
all_exceptions = (ValueError, AssertionError)
while 1:
try:
evaluation = int(raw_input("> "))
if evaluation < 1 or evaluation > 10:
raise AssertionError("\nI said between 1 and 10, moron.\n")
except all_exceptions as e:
idiocy += 1
print str(e)
else:
if idiocy == 0:
print "\nOkay, processing..."
else:
print "\nDid we finally figure out how to follow instructions?"
print "Okay, processing..."
break
希望能幫助到你。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.