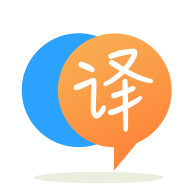
[英]LIBGDX/BOX2D: Re-sizing Touchpad is not working when the Table.setBounds is called
[英]Scaling a touchpad for use with Box2d in Libgdx
我正尝试按照PPM值缩放内容,就像我正在看的教程一样,但是后来我意识到我有触摸板,而该教程没有,所以我不知道该如何做才能将其与其他所有内容一起缩放。 我尝试过的所有方法都无效。
这是我的播放屏幕文件:如您所见,我的触摸板在其中,但没有缩放,其他所有都缩放并且可以正常工作。 注意:我的PPM值是100的浮点数
package com.cyan.rdm.Screens;
import com.badlogic.gdx.Gdx;
import com.badlogic.gdx.Input;
import com.badlogic.gdx.Screen;
import com.badlogic.gdx.graphics.Color;
import com.badlogic.gdx.graphics.GL20;
import com.badlogic.gdx.graphics.OrthographicCamera;
import com.badlogic.gdx.graphics.Texture;
import com.badlogic.gdx.graphics.g2d.BitmapFont;
import com.badlogic.gdx.maps.MapObject;
import com.badlogic.gdx.maps.objects.RectangleMapObject;
import com.badlogic.gdx.maps.tiled.TiledMap;
import com.badlogic.gdx.maps.tiled.TmxMapLoader;
import com.badlogic.gdx.maps.tiled.renderers.OrthogonalTiledMapRenderer;
import com.badlogic.gdx.math.Rectangle;
import com.badlogic.gdx.math.Vector2;
import com.badlogic.gdx.math.Vector3;
import com.badlogic.gdx.physics.box2d.Body;
import com.badlogic.gdx.physics.box2d.BodyDef;
import com.badlogic.gdx.physics.box2d.Box2DDebugRenderer;
import com.badlogic.gdx.physics.box2d.FixtureDef;
import com.badlogic.gdx.physics.box2d.PolygonShape;
import com.badlogic.gdx.physics.box2d.World;
import com.badlogic.gdx.scenes.scene2d.Stage;
import com.badlogic.gdx.scenes.scene2d.ui.Label;
import com.badlogic.gdx.scenes.scene2d.ui.Skin;
import com.badlogic.gdx.scenes.scene2d.ui.Table;
import com.badlogic.gdx.scenes.scene2d.ui.Touchpad;
import com.badlogic.gdx.scenes.scene2d.utils.Drawable;
import com.badlogic.gdx.utils.viewport.StretchViewport;
import com.badlogic.gdx.utils.viewport.Viewport;
import com.cyan.rdm.Sprites.Char;
import com.cyan.rdm.rdmGame;
public class PlayScreen implements Screen{
private rdmGame game;
private Stage stage;
private OrthographicCamera cam;
private Viewport stretchport;
private Char character;
private Label speedLabel;
private boolean speedLabelVisibility = false;
private boolean touchPadVisibility = true;
// Map Loader
private TmxMapLoader mploader;
private TiledMap map;
private OrthogonalTiledMapRenderer renderer;
// Box 2D
private World world;
private Box2DDebugRenderer b2dr;
// TouchPad
public Touchpad touchpad;
public Touchpad.TouchpadStyle touchpadStyle;
public Skin touchpadSkin;
public Drawable touchBackground;
public Drawable touchKnob;
public PlayScreen(rdmGame game) {
this.game = game;
cam = new OrthographicCamera();
stretchport = new StretchViewport(rdmGame.V_WIDTH / rdmGame.PPM, rdmGame.V_HEIGHT / rdmGame.PPM, cam);
stage = new Stage(stretchport, game.batch);
Gdx.input.setInputProcessor(stage);
Label.LabelStyle font = new Label.LabelStyle(new BitmapFont(), Color.WHITE);
Table table = new Table();
table.setPosition(15 / rdmGame.PPM, 185 / rdmGame.PPM);
speedLabel = new Label(String.format("%03d", character.SPEED), font);
table.add(speedLabel).expandX();
// Map
mploader = new TmxMapLoader();
map = mploader.load("temp_tileset2.tmx");
renderer = new OrthogonalTiledMapRenderer(map, 1 / rdmGame.PPM);
cam.position.set(stretchport.getWorldWidth() / 2,stretchport.getWorldHeight() /2, 0);
// Box 2D
world = new World(new Vector2(0, 0), true);
b2dr = new Box2DDebugRenderer();
character = new Char(world);
BodyDef bodyDef = new BodyDef();
PolygonShape hitbox = new PolygonShape();
FixtureDef fixtureDef = new FixtureDef();
Body body;
// Normal Wall Collision
for(MapObject object : map.getLayers().get(2).getObjects().getByType(RectangleMapObject.class)) {
Rectangle rectangle = ((RectangleMapObject) object).getRectangle();
bodyDef.type = BodyDef.BodyType.StaticBody;
bodyDef.position.set((rectangle.getX() + rectangle.getWidth() /2) / rdmGame.PPM ,(rectangle.getY() + rectangle.getHeight() / 2) / rdmGame.PPM);
body = world.createBody(bodyDef);
hitbox.setAsBox(rectangle.getWidth() / 2 / rdmGame.PPM, rectangle.getHeight() / 2 / rdmGame.PPM);
fixtureDef.shape = hitbox;
body.createFixture(fixtureDef);
}
// Red Wall Collision
for(MapObject object : map.getLayers().get(3).getObjects().getByType(RectangleMapObject.class)) {
Rectangle rectangle = ((RectangleMapObject) object).getRectangle();
bodyDef.type = BodyDef.BodyType.StaticBody;
bodyDef.position.set((rectangle.getX() + rectangle.getWidth() / 2) / rdmGame.PPM, (rectangle.getY() + rectangle.getHeight() / 2) / rdmGame.PPM);
body = world.createBody(bodyDef);
hitbox.setAsBox(rectangle.getWidth() / 2 / rdmGame.PPM, rectangle.getHeight() / 2 / rdmGame.PPM);
fixtureDef.shape = hitbox;
body.createFixture(fixtureDef);
}
//TouchPad
touchpadSkin = new Skin();
touchpadSkin.add("touchBackground", new Texture("touchBackground2.png"));
touchpadSkin.add("touchKnob", new Texture("touch_circle2.png"));
touchpadStyle = new Touchpad.TouchpadStyle();
touchBackground = touchpadSkin.getDrawable("touchBackground");
touchKnob = touchpadSkin.getDrawable("touchKnob");
touchpadStyle.background = touchBackground;
touchpadStyle.knob = touchKnob;
touchpad = new Touchpad(10, touchpadStyle);
touchpad.setBounds(8, 8, 64, 64);
touchpad.scaleBy(1 / rdmGame.PPM,1 / rdmGame.PPM);
stage.addActor(table);
stage.addActor(touchpad);
touchpad.setVisible(touchPadVisibility);
}
@Override
public void show() {
}
public void update(float dt) {
// System.out.println("Char: " + character.b2Body.getPosition());
// System.out.println("Touch: " + touchpad.);
speedLabel.setText(String.format("%03d", character.SPEED));
speedLabel.setVisible(speedLabelVisibility);
handeInput(dt);
world.step(1/60f, 6, 6);
cam.update();
renderer.setView(cam);
}
@Override
public void render(float delta) {
update(delta);
Gdx.gl.glClearColor(255 ,165, 0, 0);
Gdx.gl.glClear(GL20.GL_COLOR_BUFFER_BIT);
stage.draw();
renderer.render();
b2dr.render(world, cam.combined);
//Batch
game.batch.setProjectionMatrix(cam.combined);
game.batch.begin();
game.batch.end();
stage.act(Gdx.graphics.getDeltaTime());
stage.draw();
}
public void handeInput(float dt){
if(Gdx.input.isKeyJustPressed(Input.Keys.ESCAPE)){
game.setScreen(new MenuScreen(game));
}
if(Gdx.input.isKeyJustPressed(Input.Keys.UP)){
}
if(Gdx.input.isKeyJustPressed(Input.Keys.DOWN))
if(Gdx.input.isKeyJustPressed(Input.Keys.F3)){
speedLabelVisibility = !speedLabelVisibility;
}
if(touchpad.getKnobPercentX() == 0 && touchpad.getKnobPercentY() == 0) {
character.b2Body.setLinearVelocity(new Vector2(0, 0));
} else {
character.b2Body.setLinearVelocity(new Vector2(character.b2Body.getLinearVelocity().x + touchpad.getKnobPercentX()*character.SPEED, character.b2Body.getLinearVelocity().y + touchpad.getKnobPercentY()*character.SPEED));
}
}
@Override
public void resize(int width, int height) {
stretchport.update(width, height);
}
@Override
public void pause() {
}
@Override
public void resume() {
}
@Override
public void hide() {
}
@Override
public void dispose() {
stage.dispose();
}
}
我建议您使用两个不同的视口。 一个用于您的主要阶段,除以PPM,另一个用于具有正常分辨率的UI。
您不在触摸板上使用ppm。 Ppm在物理世界中仅为每米像素。 Ppm与Gui无关。 您仍然使用Gui的屏幕坐标和大小。 因此,请使用像素/ ppm大小的摄像头和视口为游戏项目使用主舞台,然后对Gui使用另一个舞台。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.