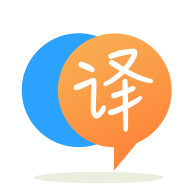
[英]I'm trying to figure out what is the best way to prevent from the player to move through walls and other objects?
[英]How can i find the walls boundaries and compare them to the 4 directions from the player to find what directions the player can move to?
我有一个立方体网格。 在这种情况下,网格大小为10x10。每个多维数据集大小为1x1。每个墙都是在墙下的子级,在每个墙上都有作为子级的墙体。
墙的父GameObject位置为X = 29.4 Y = 0.5 Z = 10.9并且每个墙位置为0,0,0
创建网格后,我先找到墙壁,然后获取玩家当前位置,然后从玩家+ 1.5f距离获取每个方向。
接下来,我想找到玩家可以移动的方向。 向前,向左,向右,向后,因为每次每次在墙壁边缘一次运行游戏时都会产生玩家,则不能向一个或两个方向移动。
问题是如何找到不能移动的方向和可以移动的方向?
private void Directions()
{
GameObject walls = GameObject.Find("Walls");
Vector3 playerPosition = player.position;
Vector3 rightOnePointFive = player.localPosition + player.right * 1.5f;
Vector3 leftOnePointFive = player.localPosition - player.right * 1.5f;
Vector3 forwardOnePointFive = player.localPosition + player.forward * 1.5f;
Vector3 backOnePointFive = player.localPosition - player.forward * 1.5f;
}
这就是我创建网格和墙的方式:
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class GridGenerator : MonoBehaviour
{
public GameObject gridBlock;
public int gridWidth = 10;
public int gridHeight = 10;
public GameObject[] allBlocks;
private GameObject[] wallsParents = new GameObject[4];
void Start()
{
wallsParents[0] = GameObject.Find("Top Wall");
wallsParents[1] = GameObject.Find("Left Wall");
wallsParents[2] = GameObject.Find("Right Wall");
wallsParents[3] = GameObject.Find("Bottom Wall");
GenerateGrid();
allBlocks = GameObject.FindGameObjectsWithTag("Blocks");
var findpath = GetComponent<PathFinder>();
findpath.FindPath();
}
public void AutoGenerateGrid()
{
allBlocks = GameObject.FindGameObjectsWithTag("Blocks");
for (int i = 0; i < allBlocks.Length; i++)
{
DestroyImmediate(allBlocks[i]);
}
var end = GameObject.FindGameObjectWithTag("End");
DestroyImmediate(end);
GenerateGrid();
allBlocks = GameObject.FindGameObjectsWithTag("Blocks");
var findpath = GetComponent<PathFinder>();
findpath.FindPath();
}
public void GenerateGrid()
{
for (int x = 0; x < gridWidth; x++)
{
for (int z = 0; z < gridHeight; z++)
{
GameObject block = Instantiate(gridBlock, Vector3.zero, gridBlock.transform.rotation) as GameObject;
block.transform.parent = transform;
block.transform.name = "Block";
block.transform.tag = "Blocks";
block.transform.localPosition = new Vector3(x * 1.5f, 0, z * 1.5f);
block.GetComponent<Renderer>().material.color = new Color(241, 255, 0, 255);
if (x == 0)//TOP
{
block.transform.parent = wallsParents[0].transform;
block.transform.name = "TopWall";
block.transform.tag = "Blocks";
}
else if (z == 0)//LEFT
{
block.transform.parent = wallsParents[1].transform;
block.transform.name = "LeftWall";
block.transform.tag = "Blocks";
}
else if (z == gridHeight - 1)//RIGHT
{
block.transform.parent = wallsParents[2].transform;
block.transform.name = "RightWall";
block.transform.tag = "Blocks";
}
else if (x == gridWidth - 1)//BOTTOM
{
block.transform.parent = wallsParents[3].transform;
block.transform.name = "BottomWall";
block.transform.tag = "Blocks";
}
}
}
}
}
更新:
我尝试了这种方法:
private GridGenerator gridgenerator;
public void FindDirection()
{
gridgenerator = GetComponent<GridGenerator>();
GenerateStartEnd();
Directions();
}
private void Directions()
{
Vector3 playerPosition;
playerPosition = player.localPosition;
if (playerPosition.x > 0)
{
// can go left
possibleDirections[0] = "Can go left";
}
if (playerPosition.x + 1 < gridgenerator.gridWidth)
{
// can go right
possibleDirections[1] = "Can go right";
}
if (playerPosition.z > 0)
{
// can go forward
possibleDirections[2] = "Can go forward";
}
if (playerPosition.z + 1 < gridgenerator.gridHeight)
{
// can go backward
possibleDirections[3] = "Can go backward";
}
}
但是在运行游戏时:
我不能走的唯一方向是左,但这是说在检查员中我可以走。 可以前进,但不能后退和正确。
我搞砸了
在这种情况下,gridWidth和gridHeight均为10,但可以为23x5或9x7或12x12之类的任何大小
最简单的方法是使用二维数组。
这样,通过保存当前玩家位置很容易检查玩家可以去哪里。 这意味着,如果您的玩家在位置[0,5]上,他将无法向左侧移动。
另外,您还可以自动生成该字段并轻松调整其大小。
编辑:但是,如果您确实想保留当前的解决方案,则可以在每道墙中循环,并在想向左移动时检查x位置是否小于当前玩家位置。 其他方向也一样。
看看这张美丽的图画,这是一个更好的例子。
如果您有一个二维数组,则可以存储当前玩家位置,然后执行以下代码。
好的,所以也许我不够清楚,但是已经是凌晨4点了,我有点累了。 您需要做的是存储当前的playerPosition,它只能是整数。 因此,可能性例如为:[0,0],[3,0],[2,3]。 每当您将播放器向左移动时,您都必须更新其在网格上的位置,例如,当您向左移动时,playerPosition.X--;
Vector2 playerPos = new Vector(0, 3);
int maxWidthOfGrid = 5;
private void Directions()
{
if(playerPos.X > 0) {
//player can go left
}
if(playerPos.X+1 < maxWidthOfGrid) {
//player can go right
}
}
我没有测试代码,所以也许您需要对其进行一些修改。
底部和顶部方向也是如此。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.