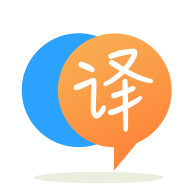
[英]Object Oriented Python Program calculating volume and Surface area of a sphere
[英]How to find the area and volume of Sphere and cylinder in same program in python
任务是找到形状的面积和体积。 设计代码时,请考虑以下形状:球形和圆柱体。 该类应该只有一个构造函数。 另外,我们应该创建一个名为Shape的类,下面是我编写的代码并返回输出,但是如何使用新类进行形状并继承到测试或其他方法中。
import math
#class Shapes
class Test:
def __init__(self, *args):
self.radius = args[0]
if (len(args) == 2):
self.height = args[1]
self.area = 0
self.volume = 0
return super().__init__()
def Area(self,val):
radius = self.radius
if (val == "Sphere"):
area = 4 * 3.14 * radius ** 2
elif (val =="Cylinder"):
height = self.height
area = ((2 * 3.14 * radius) * (radius + height))
else:
area = 0
return area
def Volume(self,val):
radius = self.radius
if (val == "Sphere"):
volume = (4/3) * (3.14 * radius ** 2)
elif (val == "Cylinder"):
height = self.height
volume = 3.14 * radius * radius * height
else:
volume = 0
return volume
def main():
cylinder=Test(2,4)
print('Cylinder area:',cylinder.Area(enter code here'Cylinder'))
print('Cylinder volume:',cylinder.Volume('Cylinder'))
sphere=Test(3)
print('Sphere area:',sphere.Area('Sphere'))
print('Sphere volume:',sphere.Volume('Sphere'))
if __name__=='__main__':
main()
如问题中所指定:
class Shape
。 class Shape
派生一个class Sphere
和一个class Cylinder
class Shape
。 然后,为了使用形状
下面的示例可以做到这一点。
import math
class Shape:
"""abstract class for shapes"""
def __init__(self, radius):
self.radius = radius
def get_surface(self):
raise NotImplementedError
def get_volume(self):
raise NotImplementedError
class Sphere(Shape): # <-- inherits from Shape
def __init__(self, radius):
Shape.__init__(self, radius) # <-- can also use super().__init__(radius)
def get_surface(self):
return 4 * math.pi * self.radius**2 # <-- use math.pi constant i/o hard coding the value of pi
def get_volume(self):
return math.pi * self.radius**3 * 4/3
class Cylinder(Shape): # <-- inherits from Shape
def __init__(self, radius, height):
Shape.__init__(self, radius) # <-- can also use super().__init__(radius)
self.height = height
def get_surface(self):
return 2 * math.pi * self.radius * self.height + 2 * math.pi * self.radius**2
def get_volume(self):
return math.pi * self.radius**2 * self.height
def main():
cyl = Cylinder(2, 4)
print('Cylinder area:', cyl.get_surface()) # <-- call the methods on the object
print('Cylinder volume:', cyl.get_volume())
sph = Sphere(5)
print('Sphere area:',sph.get_surface())
print('Sphere volume:',sph.get_volume())
if __name__=='__main__':
main()
Cylinder area: 75.3982236862
Cylinder volume: 50.2654824574
Sphere area: 314.159265359
Sphere volume: 523.598775598
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.