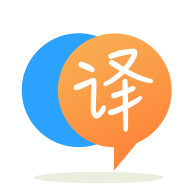
[英]How to extend one sublist by another sublist if they share a common id at the same index in both sublists?
[英]How do I merge into one sublist the two sublists with the same index 0?
这与拼合列表不同。
我有以下列表列表:
listoflists = [[853, 'na'], [854, [1, 2, 3, 4, 5]], [854, [2, 4, 6, 8]]
我希望合并具有相同索引0(在本例中为854)的那些子列表,但不要使其扁平化,如下所示:
listoflists_v2 = [[853, 'na'], [854, [1, 2, 3, 4, 5], [2, 4, 6, 8]]]
我怎么做?
如果顺序很重要,请使用OrderedDict
并按键收集值:
from collections import OrderedDict
d = OrderedDict()
for k, v in listoflists:
d.setdefault(k, []).append(v)
listoflists_v2 = [[k, *v] for k, v in d.items()]
如果没有,请使用defaultdict
,您会获得更好的性能:
from collections import defaultdict
d = defaultdict(list)
for k, v in listoflists:
d[k].append(v)
listoflists_v2 = [[k, *v] for k, v in d.items()]
另一个选择是使用itertools.groupby
:
from itertools import groupby
from operator import itemgetter
listoflists.sort(key=itemgetter(0)) # Do this if keys aren't consecutive.
listoflists_v2 = [
[k, *map(itemgetter(1), g)]
for k, g in groupby(listoflists, key=itemgetter(0))
]
print(listoflists_v2)
[[853, 'na'], [854, [1, 2, 3, 4, 5], [2, 4, 6, 8]]]
尽管我不建议这样做,但这是另一种解决方法。 我猜它对学习有好处。
# orginal list
listoflists = [[853, 'na'], [854, [1, 2, 3, 4, 5]], [854, [2, 4, 6, 8]]]
# new list with combined data
new_list = []
# loop through all sublists
for sub_list in listoflists:
# check if new_list is empty to see if its data should be compared
# with the orinal if not add sublist to new_list
if new_list:
# check all the lists in new_list
for list_ in new_list:
# if the list in new_list and one of the original lists
# first element match, add the values of the original list
# starting from the first elemnt to the new_list
if sub_list[0] == list_[0]:
list_.append(sub_list[1:])
else:
list_.append(sub_list)
else:
new_list.append(sub_list)
print(new_list)
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.