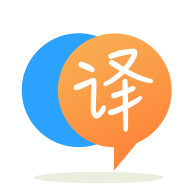
[英]How do I bring a processes window to the foreground on X Windows? (C++)
[英]How do I bring a polygon to the foreground in OpenGL?
下面的代码创建了2个方形多边形,红色和绿色。
我正试图在绿色的顶部放置一个红色方块,但我不能。
声明深度缓冲区,必要时清理,正确配置正交系统。
如果我指定一个超出范围(2; -2)的值,则多边形将按原样消失。
#include <...>
constexpr auto FPS_RATE = 120;
int windowHeight = 600, windowWidth = 600, windowDepth = 600;
void init();
void idleFunction();
void displayFunction();
double getTime();
double getTime()
{
using Duration = std::chrono::duration<double>;
return std::chrono::duration_cast<Duration>(
std::chrono::high_resolution_clock::now().time_since_epoch()
).count();
}
const double frame_delay = 1.0 / FPS_RATE;
double last_render = 0;
void init()
{
glutDisplayFunc(displayFunction);
glutIdleFunc(idleFunction);
glMatrixMode(GL_PROJECTION);
glLoadIdentity();
glOrtho(-windowWidth / 2, windowWidth / 2, -windowHeight / 2, windowHeight / 2, 2, -2);
glClearColor(0.0, 0.0, 0.0, 0.0);
}
void idleFunction()
{
const double current_time = getTime();
if ((current_time - last_render) > frame_delay)
{
last_render = current_time;
glutPostRedisplay();
}
}
void displayFunction()
{
glClear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT);
glPushMatrix();
//move the red square to the foreground
glTranslatef(-32.5, -32.5, 2);
glColor3f(1, 0, 0);
glBegin(GL_POLYGON);
glVertex3i(-150, 150, 0);
glVertex3i(150, 150, 0);
glVertex3i(150, -150, 0);
glVertex3i(-150, -150, 0);
glEnd();
glPopMatrix();
glPushMatrix();
//move the green square to the background
glTranslatef(32.5, 32.5, -2);
glColor3f(0, 1, 0);
glBegin(GL_POLYGON);
glVertex3i(-150, 150, 0);
glVertex3i(150, 150, 0);
glVertex3i(150, -150, 0);
glVertex3i(-150, -150, 0);
glEnd();
glPopMatrix();
glutSwapBuffers();
}
int main(int argc, char* argv[])
{
glutInit(&argc, argv);
glutInitDisplayMode(GLUT_DOUBLE | GLUT_RGB);
glutInitWindowSize(windowWidth, windowHeight);
glutInitWindowPosition((GetSystemMetrics(SM_CXSCREEN) - windowWidth) / 2, (GetSystemMetrics(SM_CYSCREEN) - windowHeight) / 2);
glutCreateWindow("Window");
init();
glutMainLoop();
return 0;
}
你要启用深度测试 :
glEnable( GL_DEPTH_TEST );
默认深度测试功能( glDepthFunc
)为<
( GL_LESS
)。
如果到远平面的距离为2.0并且使用z坐标为2.0绘制几何图形,则几何图形将被远平面剪切,因为几何图形的深度不小于深度缓冲区的初始化深度。
将深度函数更改为<=
( GL_LEQUAL
):
glDepthFunc( GL_LEQUAL );
在右手系统中,视图空间z轴指向视口外。 因此,如果z坐标是“小于”,则该对象在另一个对象“后面”。
投影矩阵从视图空间转换为规范化设备空间。 与视图空间相比,规范化设备空间是左手系统,其中z轴指向视口中。 范围[-1,1](从前到后)的归一化设备z坐标被映射到深度值(通常在范围[0,1]中),其用于深度测试。
要处理glOrtho
反转z轴,如果near参数设置小于far参数(这是建议使用的函数)。
这导致当几何体从视图空间转换为规范化设备空间时,深度(z)顺序不会改变。
注意, glOrtho(-w, w, -h, h, -z, z)
与glScaled(1.0/w, 1.0/h, -1.0/z)
相同glScaled(1.0/w, 1.0/h, -1.0/z)
由于z轴在您的示例中未被正投影反转,因为近 > 远 ,
glOrtho(-windowWidth / 2, windowWidth / 2, -windowHeight / 2, windowHeight / 2, 2, -2);
z坐标必须更大,才能“落后”。
如果绿色矩形应该在红色矩形后面,那么你必须改变正交投影( 靠近 < 远 )。 例如:
glOrtho(-windowWidth / 2, windowWidth / 2, -windowHeight / 2, windowHeight / 2, -2, 2);
如果您不想更改投影,则需要交换几何的z坐标:
glPushMatrix();
//move the red square to the foreground
glTranslatef(-32.5, -32.5, -2.0); // foreground because near > far
// ...
glPopMatrix();
glPushMatrix();
//move the green square to the background
glTranslatef(32.5, 32.5, 2.0); // background because near > far
// ...
glPopMatrix();
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.