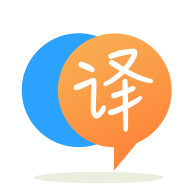
[英]how to get index range for zero and non-zero value from list in python?
[英]Get the index of the next non-zero value in a list in python
假设您有一个列表[1,2,3,4,5,0,0,0,7,8, ...]
。 给定当前索引,获得下一个非零索引的最佳方法是什么? 例如,如果 index 是 2,那么下一个非零的一个是 3; 如果为 4,则为 8。
a = [1,2,3,4,5,0,0,0,7,8]
def nextZeroIndex(array, start):
nextItems = array[start+1:]
for i, x in enumerate(nextItems):
if x != 0:
return 1 + start + i
print(nextZeroIndex(a, 2)) # 3
print(nextZeroIndex(a, 5)) # 8
print(nextZeroIndex(a, 4)) # 8
使用过滤器查找列表中的第一个非零项
i+list(filter(lambda x: x[1]!=0, enumerate(arr[i:])))[0][0]
# for i=5, the result is 8
常规循环
arr = [1,2,3,4,5,0,0,0,7,8,0]
def firstNonZero(arr,i):
for k,v in enumerate(arr[i:]):
if v:
break
else :
return None
return k+i
print(firstNonZero(arr,5)) # 8
print(firstNonZero(arr,10)) # None
def get_next_index(lst, index):
try:
return next(i + index + 1 for i, n in enumerate(lst[index + 1:]) if n != 0)
except StopIteration:
return None
lst = [1, 2, 3, 4, 5, 0, 0, 0, 7, 8]
get_next_index(lst, 2) # 3
get_next_index(lst, 9) # None
get_next_index(lst, 100) # None
从指定索引开始遍历列表,直到找到一个不为零的数字,如果索引超出范围或找到非零返回None
您也可以使用 itertools 实现此目的:
from itertools import compress,count
start = 4
next = list(compress(count(),l[start+1:]))[0]+start+1
print(next)
output:
8
你可以有一个 class 存储所有“有效”索引(非零值),当你想从给定索引开始查找非零值的下一个索引时,你只需搜索下一个有效索引大于您的起始索引
from bisect import bisect_right
class NextIndexNonZero:
def __init__(self, your_numbers: list):
# filter out the indices for zero values/numbers
valid_indices = filter(lambda x: x[1] != 0, enumerate(your_numbers))
self.valid_indices = list(map(lambda x: x[0], valid_indices))
self.max_index = self.valid_indices[-1]
def find(self, start_index: int) -> int:
if start_index >= self.max_index:
return f'There is no index non-zero greater than {start_index}'
next_index = bisect_right(self.valid_indices, start_index)
return self.valid_indices[next_index]
a = [1, 0, 0, 0, 1, 0, 0 ]
n = NextIndexNonZero(a)
print(n.find(2))
print(n.find(4))
output:
4
There is no index non-zero greater than 4
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.