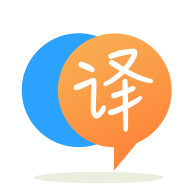
[英]how to display 2 buttons and 2 labels using pyqt5 in python?
[英]How to display a timer using PyQt5 for Python
我正在制作一款游戏,我希望在用户点击“新游戏”后在屏幕上显示一个计时器,以跟踪他们玩了多长时间。 我有一个单独运行计时器的类,但是当我将它合并到我的游戏的其余部分然后最重要的是,尝试显示计时器的更新值时,UI 中的任何值都不会更新并且打印输出计时器甚至不会出现在终端中。 我试过在与游戏设置过程相同的线程中运行计时器,我也尝试过创建一个新线程来运行计时器,但都不起作用。 游戏加载并运行正常,除了计时器不向上计数并且不显示更新的计时器值。 我哪里错了?
这是我的独立 Timer 类,它本身也能正常工作。
from PyQt5 import QtCore
import sys
def startThread(functionName, *args, **kwargs):
print(args)
if len(args) == 0:
t = threading.Thread(target=functionName)
else:
try:
t = threading.Thread(target=functionName, args=args, kwargs=kwargs)
except:
try:
if args is None:
t = threading.Thread(target=functionName, kwargs=kwargs)
except:
t = threading.Thread(target=functionName)
t.daemon = True
t.start()
class Timer(object):
def __init__(self):
super(Timer, self).__init__()
def start_timer(self):
print("Starting timer...")
Timer.timer = QtCore.QTimer()
Timer.time = QtCore.QTime(0, 0, 0)
Timer.timer.timeout.connect(self.tick)
Timer.timer.start(1000)
def tick(self):
Timer.time = Timer.time.addSecs(1)
self.update_UI('%s' % Timer.time.toString("hh:mm:ss"))
def update_UI(self, text_string):
print(text_string)
# This is where the text would be sent to try and update the UI
Timer().start_timer()
这或多或少是我的游戏设置类的结构——目前我展示的是使用线程的版本:
class BuildUI(PyQt5.QtWidgets.QMainWindow, Ui_game):
def __init__(self):
super(BuildUI, self).__init__()
self.setupUi(self)
self.easy_mode = 38
self.user_available_cells = []
self.start_easy_game.triggered.connect(lambda: self.setup_game(self.easy_mode))
def setup_game(self, hidden_count):
def create_game_board():
startThread(Timer().start_timer)
self.game = BuildGame()
#The BuildGame class is not deliberately not shown
startThread(create_game_board)
class GAME(object):
def __init__(self):
GAME.app = PyQt5.QtWidgets.QApplication(sys.argv)
GAME.UI = BuildUI()
GAME.UI.show()
GAME.app.exec_()
def main():
GAME()
if __name__ == '__main__':
main()
让它工作的关键是使用信号。 完全保留Timer
类,唯一要做的修改是在GAME
类的初始化中,需要在 BuildUI 类的开头添加一个信号,然后使用 emit() 在self.game = BuildGame()
调用。
class BuildUI(PyQt5.QtWidgets.QMainWindow, sudoku_ui.Ui_sudoku_game):
# This signal just triggers a msgbox to display, telling the user the game is loading
process_start = PyQt5.QtCore.pyqtSignal()
# this is called to automatically close the msgbox window
process_finished = PyQt5.QtCore.pyqtSignal()
# This signal, when called will start the timer
start_game_timer = PyQt5.QtCore.pyqtSignal()
def __init__(self):
super(BuildUI, self).__init__()
self.setupUi(self)
self.easy_mode = 38
self.user_available_cells = []
self.start_easy_game.triggered.connect(lambda: self.setup_game(self.easy_mode))
def setup_game(self, hidden_count):
def create_game_board():
self.game = BuildGame()
# Now that the game is built, the timer can start
# This is the emit which will start the timer
GAME.UI.start_game_timer.emit()
startThread(create_game_board)
class GAME(object):
def __init__(self):
GAME.app = PyQt5.QtWidgets.QApplication(sys.argv)
GAME.UI = BuildUI()
GAME.dialog_box = MsgPrompt()
# This is the key right here - initializing the timer class
GAME.timer = Timer()
# This line below attaches a function to the emit() call - which will kick off the timer
GAME.UI.start_game_timer.connect(GAME.timer.start_timer)
# The below referenced class is deliberately omitted from this post
GAME.UI.process_start.connect(GAME.dialog_box.show_dialog_box)
GAME.UI.process_finished.connect(GAME.dialog_box.hide_dialog_box)
GAME.UI.show()
GAME.app.exec_()
def main():
GAME()
if __name__ == '__main__':
main()
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.