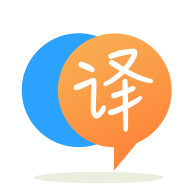
[英]Permutations of a list with 16 integers but only if 4 conditions are fulfilled
[英]Permutations with multiple list and Conditions
我想生成两个列表的每 5 个字符排列的所有可能字符串的列表
List 1: [A-Z] all caps Alphabets
List 2: [0-9] Digits
字符串的条件
示例输出:
B9B61
6F084
7C9DA
9ECF9
E7ACF
我试过了,但我知道到目前为止我无法应用条件,现在很困惑,请帮忙
from itertools import permutations
perm = [''.join(p) for p in permutations('ABCDEFGHIJKLMOPQRSTUVWXYZ0123456789', 5)]
for i in list(perm):
with open("list.txt", "a+") as file:
file.write(i)
file.write("\n")
以下将打印出所有大写字母和数字的所有 5 个字符组合,任何字符或数字不超过 3 个。
import string
import itertools
possible_combinations = itertools.permutations(string.ascii_uppercase * 3 + string.digits * 3, 5)
for possible_str in (''.join(chars) for chars in possible_combinations):
if possible_str.isnumeric() or possible_str.isalpha():
continue
else:
print(possible_str)
为了获得不超过 3 个重复的字母和数字的所有组合,我们首先查看itertools.permutations
。 给定一个可迭代的字符(一个字符串),它将返回给定长度的这些字符的所有组合
>>> [''.join(x) for x in itertools.permutations('ABC', 3)]
['ABC', 'ACB', 'BAC', 'BCA', 'CAB', 'CBA']
如果我们传递每个字符的 2 个,排列将返回每个字符最多 2 个的所有组合
>>> [''.join(x) for x in itertools.permutations('AABBCC', 3)]
['AAB', 'AAB', 'AAC', 'AAC', 'ABA', 'ABB', 'ABC', 'ABC', 'ABA', 'ABB', 'ABC', 'ABC', 'ACA', 'ACB', 'ACB', 'ACC', 'ACA', 'ACB', 'ACB', 'ACC', 'AAB', 'AAB', 'AAC', 'AAC', 'ABA', 'ABB', 'ABC', 'ABC', 'ABA', 'ABB', 'ABC', 'ABC', 'ACA', 'ACB', 'ACB', 'ACC', 'ACA', 'ACB', 'ACB', 'ACC', 'BAA', 'BAB', 'BAC', 'BAC', 'BAA', 'BAB', 'BAC', 'BAC', 'BBA', 'BBA', 'BBC', 'BBC', 'BCA', 'BCA', 'BCB', 'BCC', 'BCA', 'BCA', 'BCB', 'BCC', 'BAA', 'BAB', 'BAC', 'BAC', 'BAA', 'BAB', 'BAC', 'BAC', 'BBA', 'BBA', 'BBC', 'BBC', 'BCA', 'BCA', 'BCB', 'BCC', 'BCA', 'BCA', 'BCB', 'BCC', 'CAA', 'CAB', 'CAB', 'CAC', 'CAA', 'CAB', 'CAB', 'CAC', 'CBA', 'CBA', 'CBB', 'CBC', 'CBA', 'CBA', 'CBB', 'CBC', 'CCA', 'CCA', 'CCB', 'CCB', 'CAA', 'CAB', 'CAB', 'CAC', 'CAA', 'CAB', 'CAB', 'CAC', 'CBA', 'CBA', 'CBB', 'CBC', 'CBA', 'CBA', 'CBB', 'CBC', 'CCA', 'CCA', 'CCB', 'CCB']
如果我们增加字符的数量以及我们重复它们的数量,情况也是如此。 所以我们有string.ascii_uppercase * 3 + string.digits * 3
它返回一个字符串,其中所有大写字母重复 3 次,所有数字重复 3 次
最后一件事是过滤掉没有数字或字母的字符串。 如果所有字符都是数字,则 string.isnumeric() 返回 true,如果所有字符都是字母,则 string.isalpha() 返回 true
您可以通过应用一些检查来进行permutations
:
from itertools import permutations
from collections import Counter
for i in permutations('ABCDEFGHIJKLMOPQRSTUVWXYZ0123456789', 5):
combo = "".join(i)
result = Counter(i)
if any(s>=3 for s in result.values()) \
or sum(c.isdigit() for c in i)<1 \
or sum(c.isalpha() for c in i)<1:
continue
print (combo)
import random
output = [] #always initiate as empty
alphanum = ['A','B','C','D','E','F','G','H','I','J','K','L','M','O','P','Q','R','S','T','U','V','W','X','Y','Z',0,1,2,3,4,5,6,7,8,9]
while len(output) < 5:#5 is max
output.append(random.choice(alphanum)) #pick random from list
counter_i = counter_s = 0 #counter for integers and characters
for element in output:
if isinstance(element,int):
counter_i +=1
elif isinstance(element,str):
counter_s +=1
if (counter_i > 3 or counter_s >3) : #max 3 for numbers or strings
output.pop() #remove from list if it exceeds the limits
print(output)
['M', 'Z', 4, 'B', 9]
result = ''
for l in output:
result +=str(l)
print(result)
MZ4B9
你可以试试这个功能。 它返回唯一且至少一个字母和一位数字。
import random
alphabets = "ABCDEFGHIJKLMOPQRSTUVWXYZ"
digits = "0123456789"
def generate_code(length):
alp_len = random.randint(1,length-1)
dig_len = length-alp_len
selected = list(random.sample(alphabets,alp_len))
selected.extend (random.sample(digits,dig_len))
random.shuffle ( selected )
return ''.join(selected)
print (generate_code(5))
random.sample(人口,k)
返回从种群序列中选择的唯一元素的 ak 长度列表。 用于不放回的随机抽样。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.