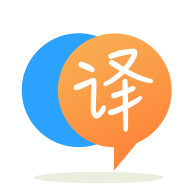
[英]Error in execution of my OpenGL C program to implement Bresenham's Line Drawing algorithm. Aborted (core dumped)
[英]How to implement Bresenham's line algorithm in C when trying to draw a line in bmp file?
我正在尝试在 bmp 文件中绘制基于用户答案的行。 为此,我在 Matrix 变量中表示文件的像素。 在阅读了 Wikipedia 中的 Bresenham's line algorithm 值和 rosettacode 示例后,我不确定我是否完全理解。 对于这个给定的代码:
void line(int x0, int y0, int x1, int y1, int** Matrix) {
int dx = abs(x1-x0), sx = x0<x1 ? 1 : -1;
int dy = abs(y1-y0), sy = y0<y1 ? 1 : -1;
int err = (dx>dy ? dx : -dy)/2, e2;
for(;;){
setPixel(x0,y0,Matrix); //color the pixel in the matrix for later use.
if (x0==x1 && y0==y1) break;
e2 = err;
if (e2 >-dx) { err -= dy; x0 += sx; }
if (e2 < dy) { err += dx; y0 += sy; }
}
}
假设:x0,y0,x1 和 y1 的值总是:3 < x < 80, 3 < y < 80。
给定的数字将始终是正整数。
真正的问题是:
如果用户设置垂直\水平线,例如 (4,5) 到 (4,20) 或 (4,5) 到 (80,5) 会发生什么,我该如何克服这个问题?
此代码适用于 dy/dx 的任何方向吗? 如:(5,5)到(40,60),
(40,60) 至 (5,5),
(20,70) 到 (50,30),
(50,30) 到 (20,70)...
在代表矩阵中画线后,写入bmp文件完全由代码中的不同function完成。 非常感谢您阅读本文。
1 如果用户设置在垂直\水平线中会发生什么,例如......我该如何克服这个问题?
只要int
值不是极端的,代码就可以正常工作。 确保减法不会溢出并且不尝试abs(INT_MIN)
。
2 此代码适用于 dy/dx 的任何方向吗? 如: ...
是的
很容易将setPixel()
更改为printf()
并检查结果。
void line_test(int x0, int y0, int x1, int y1, int **Matrix) {
printf("(%2d, %2d) to (%2d, %2d): ", x0, y0, x1, y1);
int dx = abs(x1 - x0), sx = x0 < x1 ? 1 : -1;
int dy = abs(y1 - y0), sy = y0 < y1 ? 1 : -1;
int err = (dx > dy ? dx : -dy) / 2, e2;
for (;;) {
// setPixel(x0,y0,Matrix);
(void) Matrix; // Matrix not used in this test function
printf("(%d,%d), ", x0, y0);
if (x0 == x1 && y0 == y1)
break;
e2 = err;
if (e2 > -dx) {
err -= dy;
x0 += sx;
}
if (e2 < dy) {
err += dx;
y0 += sy;
}
}
printf("\n");
}
int main(void) {
line_test(4, 5, 4, 20, NULL);
line_test(4, 5, 80, 5, NULL);
line_test(5, 5, 40, 60, NULL);
line_test(40, 60, 5, 5, NULL);
line_test(20, 70, 50, 30, NULL);
line_test(50, 30, 20, 70, NULL);
return 0;
}
Output
( 4, 5) to ( 4, 20): (4,5), (4,6), (4,7), (4,8), (4,9), (4,10), (4,11), (4,12), (4,13), (4,14), (4,15), (4,16), (4,17), (4,18), (4,19), (4,20),
( 4, 5) to (80, 5): (4,5), (5,5), (6,5), (7,5), (8,5), (9,5), (10,5), (11,5), (12,5), (13,5), (14,5), (15,5), (16,5), (17,5), (18,5), (19,5), (20,5), (21,5), (22,5), (23,5), (24,5), (25,5), (26,5), (27,5), (28,5), (29,5), (30,5), (31,5), (32,5), (33,5), (34,5), (35,5), (36,5), (37,5), (38,5), (39,5), (40,5), (41,5), (42,5), (43,5), (44,5), (45,5), (46,5), (47,5), (48,5), (49,5), (50,5), (51,5), (52,5), (53,5), (54,5), (55,5), (56,5), (57,5), (58,5), (59,5), (60,5), (61,5), (62,5), (63,5), (64,5), (65,5), (66,5), (67,5), (68,5), (69,5), (70,5), (71,5), (72,5), (73,5), (74,5), (75,5), (76,5), (77,5), (78,5), (79,5), (80,5),
( 5, 5) to (40, 60): (5,5), (6,6), (6,7), (7,8), (8,9), (8,10), (9,11), (9,12), (10,13), (11,14), (11,15), (12,16), (13,17), (13,18), (14,19), (15,20), (15,21), (16,22), (16,23), (17,24), (18,25), (18,26), (19,27), (20,28), (20,29), (21,30), (22,31), (22,32), (23,33), (23,34), (24,35), (25,36), (25,37), (26,38), (27,39), (27,40), (28,41), (29,42), (29,43), (30,44), (30,45), (31,46), (32,47), (32,48), (33,49), (34,50), (34,51), (35,52), (36,53), (36,54), (37,55), (37,56), (38,57), (39,58), (39,59), (40,60),
(40, 60) to ( 5, 5): (40,60), (39,59), (39,58), (38,57), (37,56), (37,55), (36,54), (36,53), (35,52), (34,51), (34,50), (33,49), (32,48), (32,47), (31,46), (30,45), (30,44), (29,43), (29,42), (28,41), (27,40), (27,39), (26,38), (25,37), (25,36), (24,35), (23,34), (23,33), (22,32), (22,31), (21,30), (20,29), (20,28), (19,27), (18,26), (18,25), (17,24), (16,23), (16,22), (15,21), (15,20), (14,19), (13,18), (13,17), (12,16), (11,15), (11,14), (10,13), (9,12), (9,11), (8,10), (8,9), (7,8), (6,7), (6,6), (5,5),
(20, 70) to (50, 30): (20,70), (21,69), (21,68), (22,67), (23,66), (24,65), (24,64), (25,63), (26,62), (27,61), (27,60), (28,59), (29,58), (30,57), (30,56), (31,55), (32,54), (33,53), (33,52), (34,51), (35,50), (36,49), (36,48), (37,47), (38,46), (39,45), (39,44), (40,43), (41,42), (42,41), (42,40), (43,39), (44,38), (45,37), (45,36), (46,35), (47,34), (48,33), (48,32), (49,31), (50,30),
(50, 30) to (20, 70): (50,30), (49,31), (49,32), (48,33), (47,34), (46,35), (46,36), (45,37), (44,38), (43,39), (43,40), (42,41), (41,42), (40,43), (40,44), (39,45), (38,46), (37,47), (37,48), (36,49), (35,50), (34,51), (34,52), (33,53), (32,54), (31,55), (31,56), (30,57), (29,58), (28,59), (28,60), (27,61), (26,62), (25,63), (25,64), (24,65), (23,66), (22,67), (22,68), (21,69), (20,70),
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.