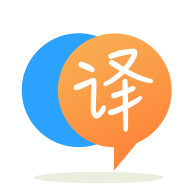
[英]How to change the horizontal movement direction of the player in Unity2D?
[英]Unity. How to turn the player in the direction of movement
正如您在此视频中看到的,object 可向任何方向移动,但其 model 不会沿移动方向旋转。 怎么解决??
视频链接
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class MoveController : MonoBehaviour
{
private CharacterController controller = null;
private Animator animator = null;
private float speed = 5f;
void Start()
{
controller = gameObject.GetComponent<CharacterController>();
animator = gameObject.GetComponent<Animator>();
}
void Update()
{
float x = Input.GetAxis("Horizontal");
float z = Input.GetAxis("Vertical");
Vector3 move = (transform.right * x) + (transform.forward * z);
controller.Move(move * speed * Time.deltaTime);
var angle = Mathf.Atan2(move.z, move.x) * Mathf.Rad2Deg;
if (x != 0 || z != 0) animator.SetTrigger("run");
if (x == 0 && z == 0) animator.SetTrigger("idle");
}
}
不要使用transform.forward
和transform.right
来制作您的移动矢量,只需在世界空间中制作即可。 然后,您可以将transform.forward
设置为移动方向。
此外,正如derHugo在下面的评论中提到的,您应该
避免使用完全相等来比较浮点数。 而是使用Mathf.Approximately
或使用您自己的阈值,如下所示
避免每帧设置触发器。 相反,您可以使用标志来确定您是否已经空闲或已经在运行,并且仅在您尚未执行此操作时才设置触发器。
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class MoveController : MonoBehaviour
{
private CharacterController controller = null;
private Animator animator = null;
private float speed = 5f;
bool isIdle;
void Start()
{
controller = gameObject.GetComponent<CharacterController>();
animator = gameObject.GetComponent<Animator>();
isIdle = true;
}
void Update()
{
float x = Input.GetAxis("Horizontal");
float z = Input.GetAxis("Vertical");
Vector3 move = new Vector3(x, 0f, z);
controller.Move(move * speed * Time.deltaTime);
var angle = Mathf.Atan2(move.z, move.x) * Mathf.Rad2Deg;
if (move.magnitude > idleThreshold)
{
transform.forward = move;
if (isIdle)
{
animator.SetTrigger("run");
isIdle = false;
}
}
else if (!isIdle)
{
animator.SetTrigger("idle");
isIdle = true;
}
}
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.