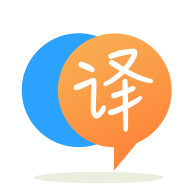
[英]How do I write a function that reads a .data file and returns an np array in python?
[英]How to properly write a python function that reads keys from a separate json file
我正在编写一个 python 脚本,它将读取 json 并查找特定的键。 到目前为止,如果我将我的 json 值作为变量包含在内,我就可以让它工作。 但是,我的最终目标是从文件中读取 json 值。 这是我一直在研究的代码:
import jwt
import os
jwks = {
"keys": [
{
"kty": "RSA",
"use": "sig",
"kid": "123456ABCDEF",
"x5t": "123456ABCDEF",
"n": "qa9f6h6h52XbX0iAgxKgEDlRpbJw",
"e": "AQAB",
"x5c": [
"43aw7PQjxt4/MpfNMS2BfZ5F8GVSVG7qNb352cLLeJg5rc398Z"
]
},
{
"kty": "RSA",
"use": "sig",
"kid": "987654ghijklmnoP",
"x5t": "987654ghijklmnoP",
"n": "qa9f6h6h52XbX0iAgxKgEDlRpbJw",
"e": "AQAB",
"x5c": [
"1234R46Qjxt4/MpfNMS2BfZ5F8GVSVG7qNb352cLLeJg5rc398Z"
]
}
]
}
class InvalidAuthorizationToken(Exception):
def __init__(self, details):
super().__init__('Invalid authorization token: ' + details)
def get_jwk(kid):
for jwk in jwks.get('keys'):
if jwk.get('kid') == kid:
print ('This is jwk:', jwk)
return jwk
raise InvalidAuthorizationToken('kid not recognized')
#Execute
get_jwk('123456ABCDEF')
get_jwk('987654ghijklmnoP')
在这里,我想要做的是替换这些相同的值并将它们存储在单独的文件 (jwks-keys) 中并将其作为变量读入。 但是,我收到以下错误并且不明白我做了什么。 我如何正确构造这个函数?
这是跟踪:
Traceback (most recent call last):
File "printjwks2.py", line 59, in <module>
get_jwk('123456ABCDEF')
File "printjwks2.py", line 51, in get_jwk
for jwk in jwks.get('keys'):
AttributeError: 'str' object has no attribute 'get'
这是函数:
def get_jwk(kid):
with open('testkeys/jwks-keys', 'r') as az:
jwks = az.read()
for jwk in jwks.get('keys'):
if jwk.get('kid') == kid:
print (jwk)
return jwk
raise InvalidAuthorizationToken('kid not recognized')
with open('testkeys/jwks-keys', 'r') as az:
jwks = az.read()
这里jwks
只是一个字符串对象,因为az.read()
将它作为 string 返回。 因此,您必须在应用jwks.get('keys')
之前将 JSON 数据反序列化为 python 对象。
>>> sample_json = '{"json_key": "json_value"}'
>>> sample_json.get("json_key")
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
AttributeError: 'str' object has no attribute 'get'
>>> import json
>>> json.loads(sample_json).get("json_key")
'json_value'
由于您正在从文件中读取 JSON,您可以使用json.load
将fp
(支持 .read() 的文本文件或包含 JSON 文档的二进制文件)反序列化为 Python 对象
>>> import json
>>> with open('testkeys/jwks-keys', 'r') as az:
... jwks = json.load(az)
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.