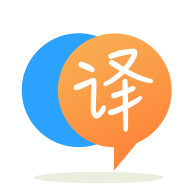
[英]How do I implement start, stop and reset features on a tkinter countdown timer?
[英]tkinter countdown app stop and reset button not working
我在 tkinter 倒计时应用程序上写了第一次尝试,我正在努力解决停止和重置按钮以及界面设计的部分问题。
我的停止按钮什么也不做,而我的重置按钮会重置值,但会立即继续倒计时。 我怀疑这是因为我在 init 方法中将self.running
设置为True
并且我的应用程序一次又一次地循环遍历这些行。 我如何防止这种情况发生?
另一个问题是我所有输入字段中的宽度参数似乎都被忽略了。 如何为所有三个字段分配相同的宽度?
谢谢你的帮助。
from tkinter import *
import time
from tkinter import messagebox
class Application(Frame):
def __init__(self,master):
super(Application,self).__init__(master)
self.place()
self.widgets()
self.running = True
def widgets(self):
self.hour = StringVar()
self.hour.set("00")
# Why is the width ignored?
self.hour_entry = Entry(width = 7, font=("Calibri",14,""), textvariable = self.hour)
self.hour_entry.place(x = 10, y = 10)
self.minute = StringVar()
self.minute.set("00")
self.minute_entry = Entry(width = 7, font=("Calibri",14,""), textvariable = self.minute)
self.minute_entry.place(x = 30, y = 10)
self.second = StringVar()
self.second.set("00")
self.seconds_entry = Entry(width = 7, font=("Calibri",14,""), textvariable = self.second)
self.seconds_entry.place(x = 50, y = 10)
self.start_btn = Button(text = "Start", command=self.clock)
self.start_btn.place(x = 30, y = 100)
self.stop_btn = Button(text = "Stop", command=self.stop)
self.stop_btn.place(x = 70, y = 100)
self.reset_btn = Button(text = "Reset", command=self.reset)
self.reset_btn.place(x = 110, y = 100)
def clock(self):
if self.running == True:
self.time_total = int(self.hour_entry.get())*3600 + int(self.minute_entry.get())*60 + int(self.seconds_entry.get())
while self.time_total > -1:
# returns 3600/60 = 60 with 0 left: so that's 60 min, 0 seconds
self.mins, self.secs = divmod(self.time_total,60)
self.hours = 0
if self.mins > 60:
self.hours, self.mins = divmod(self.mins, 60)
self.hour.set("{0:02d}".format(self.hours))
self.minute.set("{0:02d}".format(self.mins))
self.second.set("{0:02d}".format(self.secs))
self.time_total -= 1
root.update()
time.sleep(1)
if self.time_total == 0:
messagebox.showinfo("Time Countdown", "Time's up!")
def start(self):
self.running = True
self.clock()
def stop(self):
self.running = False
def reset(self):
self.running = False
self.hour.set("00")
self.minute.set("00")
self.second.set("00")
if __name__ == '__main__':
root = Tk()
app = Application(root)
mainloop()
对于计时器,使用after
方法而不是sleep
。
正在使用条目宽度,但place
方法将一个条目放在另一个条目的顶部。
此外 - 从按钮单击调用start
方法以重置running
标志。
试试这个代码:
from tkinter import *
import time
from tkinter import messagebox
class Application(Frame):
def __init__(self,master):
super(Application,self).__init__(master)
self.place()
self.widgets()
self.running = False
def widgets(self):
self.hour = StringVar()
self.hour.set("00")
# Why is the width ignored?
self.hour_entry = Entry(width = 4, font=("Calibri",14,""), textvariable = self.hour)
self.hour_entry.place(x = 10, y = 10)
self.minute = StringVar()
self.minute.set("00")
self.minute_entry = Entry(width = 4, font=("Calibri",14,""), textvariable = self.minute)
self.minute_entry.place(x = 60, y = 10)
self.second = StringVar()
self.second.set("00")
self.seconds_entry = Entry(width = 4, font=("Calibri",14,""), textvariable = self.second)
self.seconds_entry.place(x = 110, y = 10)
self.start_btn = Button(text = "Start", command=self.start)
self.start_btn.place(x = 30, y = 100)
self.stop_btn = Button(text = "Stop", command=self.stop)
self.stop_btn.place(x = 70, y = 100)
self.reset_btn = Button(text = "Reset", command=self.reset)
self.reset_btn.place(x = 110, y = 100)
def clock(self):
if self.running == True:
# returns 3600/60 = 60 with 0 left: so that's 60 min, 0 seconds
self.mins, self.secs = divmod(self.time_total,60)
self.hours = 0
if self.mins > 60:
self.hours, self.mins = divmod(self.mins, 60)
self.hour.set("{0:02d}".format(self.hours))
self.minute.set("{0:02d}".format(self.mins))
self.second.set("{0:02d}".format(self.secs))
root.update()
#time.sleep(1)
root.after(1000, self.clock) # wait 1 second, re-call clock
if self.time_total == 0:
self.running = False
messagebox.showinfo("Time Countdown", "Time's up!")
self.time_total -= 1
def start(self):
self.time_total = int(self.hour_entry.get())*3600 + int(self.minute_entry.get())*60 + int(self.seconds_entry.get())
self.running = True
self.clock()
def stop(self):
self.running = False
def reset(self):
self.running = False
self.hour.set("00")
self.minute.set("00")
self.second.set("00")
if __name__ == '__main__':
root = Tk()
app = Application(root)
mainloop()
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.