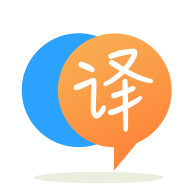
[英]how to extract values from an objects nested inside array JAVASCRIPT
[英]Extract matching values from array of objects in javascript
我有一个对象数组,我正在尝试创建一个过滤器,其中用户键入几个字母并获取所有匹配记录的列表。
users = [{office: "J Limited", contact: {first_name: "James", last_name: "Wilson", address: Canada}},{office: "Q Limited", contact: {first_name: "Quin", last_name: "Ross", address: Australia}},{office: "N Limited", contact: {first_name: "Nancy", last_name: "Mathew"}, address: "England"}]
我有一个文本字段,用户在其中键入以获取结果,并假设用户键入ja,因此结果应该搜索字段 office、contact first_name 和 last_name,如果任何此类字段包含匹配的字母,则应该是请求,在这种情况下,结果输出应该是
J Limited, James, Wilson
请帮助我实现这一目标。
要获得匹配的用户,您可以执行以下操作:
const searchString = 'ja'
const matchingUsers = users.filter(user =>
user.office.contains(searchString) ||
user.contact.first_name.contains(searchString) ||
user.contact.last_name.contains(searchString)
)
然后您可以随意格式化匹配用户列表
编辑: contains
可能无法在一些JS版本的工作(在Chrome作品),所以更换contains
有includes
:
const searchString = 'ja'
const matchingUsers = users.filter(user =>
user.office.includes(searchString) ||
user.contact.first_name.includes(searchString) ||
user.contact.last_name.includes(searchString)
)
let users = [{
office: "J Limited",
contact: { first_name: "James", last_name: "Wilson", address: "Canada" }
}, {
office: "Q Limited",
contact: { first_name: "Quin", last_name: "Ross", address: "Australia" }
}, {
office: "N Limited",
contact: { first_name: "Nancy", last_name: "Mathew", address: "England"},
}];
// ig: i for case-insensitive, g for global
const regEx = new RegExp('ja', 'ig');
const result = users.filter(
each =>
each.office.match(regEx) ||
each.contact.first_name.match(regEx) ||
each.contact.last_name.match(regEx)
)
.map(
each => [
each.office,
each.contact.first_name,
each.contact.last_name
]
);
console.log(result);
您可以遍历数组并搜索对象。
users = [{ office: "J Limited", contact: { first_name: "James", last_name: "Wilson", address: "Canada" } }, { office: "Q Limited", contact: { first_name: "Quin", last_name: "Ross", address: "Australia" } }, { office: "N Limited", contact: { first_name: "Nancy", last_name: "Mathew" }, address: "England" }, { office: "J Limited", contact: { first_name: "Jacob", last_name: "Wilson", address: "Canada" } } ] function search(searchKey) { searchKey = searchKey.toLowerCase(); results = []; for (var i = 0; i < users.length; i++) { if (users[i].contact.first_name.toLowerCase().includes(searchKey) || users[i].contact.last_name.toLowerCase().includes(searchKey) || users[i].office.toLowerCase().includes(searchKey)) { results.push(users[i]); } } return results; } var resultObject = search("ja"); if (resultObject) { for(i in resultObject){ console.log(resultObject[i].office, resultObject[i].contact.first_name, resultObject[i].contact.last_name) } }
const filterUser = users.filter(user =>{ return user.office.toLowerCase().includes((searchField).toLowerCase()) });
您的 searchField 是 input 的值,现在可以使用 filteruser ,因为它将返回搜索中包含 office 名称的用户,或者如果 searchField 中未输入任何内容则返回 all 。
您现在可以通过 filterUser 进行映射,以便能够使用包含作为您的搜索字段的输入值的用户。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.