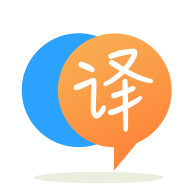
[英]How do I get my player to collide with the platform then go back to the ground after jumping off
[英]How do I make my idle animation play when my player touches the ground
所以我为我的播放器做了一个跳跃 animation,我一直想知道如何做到这一点,以便当我的播放器接触地面/平台时,它将停止播放我的跳跃 animation 并播放我的空闲 animation。我试图让它播放animation 当玩家按下空格键并且玩家的跌倒 > 0 但这会使 animation 变短,我也尝试使用计时器让 animation 播放一段时间,但这也没有用。 我只希望它在跳跃时播放 jump animation 并在它接触地面时停止播放。
我正在努力知道,这就是它正在做的事情: https://gyazo.com/5cfda577628e5596d033b11a7486bbf9?token=6f82939da2c6b2363232b3e3f379aa07
collide = False
for Platform in platforms:
if playerman.get_rect().colliderect(Platform.rect):
collide = True
playerman.isJump = False
playerman.y = Platform.rect.top - playerman.height
if playerman.rect.right > Platform.rect.left and playerman.rect.left < Platform.rect.left - playerman.width:
playerman.x = Platform.rect.left - playerman.width
if playerman.rect.left < Platform.rect.right and playerman.rect.right > Platform.rect.right + playerman.width:
playerman.x = Platform.rect.right
else:
if playerman.direction == "walk":
playerman.direction = "jump"
else:
if playerman.direction == "lwak":
playerman.direction = "ljump"
我的完整代码
import pygame
pygame.init()
# The screen width and height
window = pygame.display.set_mode((700,500))
# The name of the screen
pygame.display.set_caption("Game")
# Player class
class Player():
def __init__(self,x,y,width,height,color):
self.x = x
self.y = y
self.width = width
self.height = height
self.color = color
# Animation for player
self.idle = [pygame.image.load("HRI1.png"),
pygame.image.load("HRI2.png"),
pygame.image.load("HRI3.png"),
pygame.image.load("HRI4.png"),]
self.idlel = [pygame.image.load("HLI1.png"),
pygame.image.load("HLI2.png"),
pygame.image.load("HLI3.png"),
pygame.image.load("HLI4.png")]
self.walk = [pygame.image.load("HRW1.png"),
pygame.image.load("HRW2.png"),
pygame.image.load("HRW3.png"),
pygame.image.load("HRW4.png"),
pygame.image.load("HRW5.png"),
pygame.image.load("HRW6.png")]
self.lwalk = [pygame.image.load("HLW1.png"),
pygame.image.load("HLW2.png"),
pygame.image.load("HLW3.png"),
pygame.image.load("HLW4.png"),
pygame.image.load("HLW5.png"),
pygame.image.load("HLW6.png")]
self.jump = [pygame.image.load("HRJ1.png"),
pygame.image.load("HRJ2.png"),
pygame.image.load("HRJ3.png")]
self.ljump = [pygame.image.load("HLJ1.png"),
pygame.image.load("HLJ2.png"),
pygame.image.load("HLJ3.png")]
self.direction = "idle"
self.direction = "idlel"
self.direction = "walk"
self.direction = "lwalk"
self.direction = "jump"
self.direction = "ljump"
self.speed = 5
self.isJump = False
self.JumpCount = 10
self.fall = 0
self.rect = pygame.Rect(x,y,width,height)
self.next_frame_time = 0
self.fps = 10
self.clock = pygame.time.Clock
self.anim_index = 0
self.idle = [pygame.transform.scale(image,(image.get_width()*3,image.get_width()*3))for image in self.idle]
self.idlel = [pygame.transform.scale(image,(image.get_width()*3,image.get_width()*3))for image in self.idlel]
self.walk = [pygame.transform.scale(image,(image.get_width()*3,image.get_width()*3))for image in self.walk]
self.lwalk = [pygame.transform.scale(image,(image.get_width()*3,image.get_width()*3))for image in self.lwalk]
self.jump = [pygame.transform.scale(image,(image.get_width()*3,image.get_width()*3))for image in self.jump]
self.ljump = [pygame.transform.scale(image,(image.get_width()*3,image.get_width()*3))for image in self.ljump]
def get_rect(self):
self.rect.topleft = (self.x,self.y)
return self.rect
def draw(self):
self.rect.topleft = (self.x,self.y)
pygame.draw.rect(window,self.color,self.rect)
if self.direction == "idle":
image_list = self.idle
if self.direction == "idlel":
image_list = self.idlel
if self.direction == "walk":
image_list = self.walk
if self.direction == "lwalk":
image_list = self.lwalk
if self.direction == "jump":
image_list = self.jump
if self.direction == "ljump":
image_list = self.ljump
# Is it time to show next frame?
time_now = pygame.time.get_ticks()
if (time_now > self.next_frame_time):
# time till the nect frame
inter_time_delay = 1000 // self.fps
self.next_frame_time = time_now + inter_time_delay
# Show the next frame
self.anim_index += 1
if self.anim_index >= len(image_list):
self.anim_index = 0
if self.anim_index >= len(image_list):
self.anim_index = 0
player_image = image_list[self.anim_index]
player_rect = player_image.get_rect(center = self.get_rect().center)
player_rect.centerx += 3
player_rect.centery -= 3
window.blit(player_image, player_rect)
# Platform class
class Platform:
def __init__(self,x,y,width,height,color):
self.x = x
self.y = y
self.width = width
self.height = height
self.color = color
self.rect = pygame.Rect(x,y,width,height)
def draw(self):
self.rect.topleft = (self.x,self.y)
pygame.draw.rect(window,self.color,self.rect)
# The color of **** hitbox
white = (255,255,255)
# Player size,cords, and hitbox color
playerman = Player(255,255,40,40,white)
# Platform size,cords,and hitbox color
platform1 = Platform(0,470,400,30,white)
# Platform list
platforms = [platform1]
#redrawing window so player dose not make the screen a mess
def redrawwindow():
window.fill((0,0,0))
playerman.draw()
# making it so I do not have to draw every platform 1 by 1
for Platform in platforms:
Platform.draw()
# Fps of the game
fps = 30
clock = pygame.time.Clock()
# Runing the game/ the main loop
run = True
while run:
# Making game run with fps
clock.tick(fps)
for event in pygame.event.get():
if event.type == pygame.QUIT:
run = False
# telling what to do when we say the word 'key'
keys = pygame.key.get_pressed()
# Shortining playerman.x and playerman.y
px,py = playerman.x,playerman.y
# player 'A' movment
if keys[pygame.K_a] and px > playerman.speed:
px -= playerman.speed
playerman.direction = "lwalk"
# player 'D' movment
elif keys[pygame.K_d] and playerman.x < 700 - playerman.width - playerman.speed:
px += playerman.speed
playerman.direction = "walk"
# Playing idle animation
else:
if playerman.direction == "walk":
playerman.direction = "idle"
else:
if playerman.direction == "lwalk":
playerman.direction = "idlel"
# player 'W' movment
if keys[pygame.K_w] and py > playerman.speed:
py -= playerman.speed
# player 'S' movment
if keys[pygame.K_s] and py < 500 - playerman.height - playerman.speed:
py += playerman.speed
platform_rect_list = [p.rect for p in platforms]
player_rect = playerman.get_rect()
player_rect.topleft = (px, py)
playerman.y = py
if player_rect.collidelist(platform_rect_list) < 0:
playerman.x = px
if not playerman.isJump:
playerman.y += playerman.fall
playerman.fall += 0.5
playerman.isJump = False
# For player to get on top of platform
collide = False
for Platform in platforms:
if playerman.get_rect().colliderect(Platform.rect):
collide = True
playerman.isJump = False
playerman.y = Platform.rect.top - playerman.height
if playerman.rect.right > Platform.rect.left and playerman.rect.left < Platform.rect.left - playerman.width:
playerman.x = Platform.rect.left - playerman.width
if playerman.rect.left < Platform.rect.right and playerman.rect.right > Platform.rect.right + playerman.width:
playerman.x = Platform.rect.right
else:
if playerman.direction == "walk":
playerman.direction = "jump"
else:
if playerman.direction == "lwak":
playerman.direction = "ljump"
# Making it so player wont fall out of the map
if playerman.rect.bottom >= 500:
collide = True
playerman.isJump = False
playerman.JumpCount = 10
playerman.y = 500 - playerman.height
# Player jumping
if collide:
if keys[pygame.K_SPACE]:
playerman.isJump = True
playerman.fall = 0
# What will happen when player jumps
else:
if playerman.JumpCount >= 0:
playerman.y -= (playerman.JumpCount*abs(playerman.JumpCount))*0.3
playerman.JumpCount -= 1
else:
playerman.isJump = False
playerman.JumpCount = 10
# redrawing the window
redrawwindow()
# updating the game
pygame.display.update()
# quiting the game
pygame.quit()
当玩家与平台发生碰撞时切换到“空闲”animation,当前 animation 为“跳跃”:
collide = False
for Platform in platforms:
if playerman.get_rect().colliderect(Platform.rect):
collide = True
playerman.isJump = False
if playerman.direction == "jump":
playerman.direction = "idle":
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.