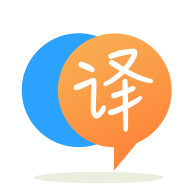
[英]AttributeError: '<Class name>' object has no attribute 'request'
[英]AttributeError 'class name' object has no attribute 'method'
import numpy as np
import math
class CellLinked ():
def __init__ (self, box_len: float, r_cut: float) :
"""
This class initialize with simulation box (L*L*L).
Here, L=box_len and r_cut is a cut-off radius.
no_cells is number of cell per axis
"""
self.box_len = box_len
self.r_cut = r_cut
self.no_cells = math.floor (self.box_len/self.r_cut)
def __call__ (self, postate: np.ndarray) :
"""
Compute the head and list array using simulation box length, cut-off radius, and the position state.
Parameters:
postate (np.ndarray): position of particles
Returns
head_arr (list): head array in the linked list (head_arr.shape==number of cells)
list_arr (list): list array in the linked list (list_arr.shape==number of particles)
"""
# Initialize a Head array with -1, size = the total number of cells
head_arr = [-1] * self.no_cells*self.no_cells*self.no_cells
# Initialize a list array with -1, size = the total number of particles
list_arr = [-1] * postate.shape[0]
for i in range (0, postate.shape[0]) :
# calculate cell index of each particles based on the particle position
index = self._cellindex (postate[i,:])
# calculate list array
list_arr [i] = head_arr [index]
# calculate head array
head_arr [index] = i
return head_arr, list_arr
def _cellindex (self, postate) :
""" Calculate the cell index based on the i, j, and k index
"""
i = math.floor (postate[0]/self.r_cut)
j = math.floor (postate[1]/self.r_cut)
k = math.floor (postate[2]/self.r_cut)
return int (k+j*self.no_cells+i*self.no_cells*self.no_cells)
def _find_neighbor_index (self, cellind) :
"""
calculate neighbor cell index based on the cell index
"""
# calculate i, j, and k index based on the cell index
i_counter = math.floor (cellind/self.no_cells**2)
j_counter = math.floor ((cellind - i_counter*self.no_cells**2)/self.no_cells)
k_counter = math.floor (cellind - j_counter*self.no_cells - i_counter*self.no_cells**2)
# calculate neighbor cell index for each cell index
index = [-1,0,1]
neighbor_cell_inx = []
for ii in index :
for jj in index :
for kk in index :
if 0 <= ii + i_counter < self.no_cells and 0 <= jj + j_counter < self.no_cells and 0 <= kk + k_counter < self.no_cells :
new_index = (kk + k_counter) + (jj + j_counter) *self.no_cells + (ii + i_counter) *self.no_cells*self.no_cells
neighbor_cell_inx.append(new_index)
return neighbor_cell_inx
def _find_neighbor_cells (self, head_arr) :
"""
calculate neighbor cells based on head array
"""
neighbor_cells = []
for j in range (0, len (head_arr)) :
neighbor_cell_inx = self._find_neighbor_index (j)
neighbor_cells.append(neighbor_cell_inx)
return neighbor_cells
def _find_particles_inside_neighbor_cells (self, list_arr, head_arr, neighbor_cells) :
"""
find particles in each cell and neighbor cells are identified
"""
new_head_arr =[]
for i in neighbor_cells :
new_head_arr.append (head_arr[i])
temporary_state = np.zeros((1,3))
for ii in new_head_arr:
if ii > -1 :
cell_state = np.zeros((1,3))
cell_state = np.concatenate((cell_state, np.array ([postate[ii]])))
while list_arr[ii] != -1 :
cell_state = np.concatenate((cell_state, np.array ([postate[list_arr[ii]]])))
ii = list_arr[ii]
cell_state = np.flip(cell_state[1:, :], 0)
temporary_state = np.concatenate((temporary_state, cell_state))
temporary_state = temporary_state[1:, :]
return temporary_state
if __name__=="__main__":
box_len=3
r_cut= 1
postate = box_len * np.random.random_sample((50, 3))
model = CellLinked (box_len, r_cut)
head_arr, list_arr = model (postate)
neighbor_cells = model._find_neighbor_cells(head_arr)
neighbor_cells = neighbor_cells[13]
temporary_state = model._find_particles_inside_neighbor_cells (list_arr, head_arr, neighbor_cells)
mask = np.isin(postate, temporary_state)
assert np.all(mask == True) == True, 'particles in neighbor cells has been indetified wrongly'
print ("the head array is : \n ", head_arr)
print ("list array is : \n ", list_arr)
嗨,伙计们,通过从这个 class 创建一个 object 来调用“_find_particles_inside_neighbor_cells”时,我遇到了一个愚蠢的问题。 当我在这里使用“if name ==" main ":" 运行此代码时,代码运行成功。 但是当我想在 python 文件中的另一个文件中导入这个 class 时,如下所示:
import numpy as np
from cell_linked_list import CellLinked
box_len=3
r_cut= 1
postate = box_len * np.random.random_sample((50, 3))
model = CellLinked (box_len, r_cut)
head_arr, list_arr = model (postate)
neighbor_cells = model._find_neighbor_cells(head_arr)
neighbor_cells = neighbor_cells[13]
temporary_state = model._find_particles_inside_neighbor_cells (list_arr, head_arr, neighbor_cells)
mask = np.isin(postate, temporary_state)
assert np.all(mask == True) == True, 'particles in neighbor cells has been indetified wrongly'
print ('\n')
print ('--------------------------------------------------')
print ('the CellLinked class is verified by the given test')
print ('--------------------------------------------------')
在使用上面的测试function时,出现这个错误:
temporary_state = model._find_particles_inside_neighbor_cells (list_arr, head_arr, neighbor_cells)
AttributeError: 'CellLinked' object has no attribute '_find_particles_inside_neighbor_cells'
如果您查看此错误并告诉我如何解决此错误,我将不胜感激。 谢谢
当我在我的机器上尝试它时,错误不是你得到的。
发生这种情况是因为您的全局变量postate
是在条件if __name__ == "__main__":
中定义的,当您在其他模块中导入此模块时不会执行。 为了克服这个问题,您必须在模块cell_linked_list
的全局级别在 if 条件之外定义postate
变量,以及变量box_len
和r_cut
。
box_len = 3
r_cut = 1
postate = box_len * np.random.random_sample((50, 3))
if __name__ == "__main__":
# rest of your code (which won't be executed if this module is imported in some other file)
还需要在第二个模块中进行更改,因为您需要更新导入模块cell_linked_list
的全局变量:
import numpy as np
import cell_linked_list as so
from cell_linked_list import CellLinked
so.box_len = 3
so.r_cut = 1
so.postate = so.box_len * np.random.random_sample((50, 3))
model = CellLinked(so.box_len, so.r_cut)
head_arr, list_arr = model(so.postate)
neighbor_cells = model._find_neighbor_cells(head_arr)
neighbor_cells = neighbor_cells[13]
temporary_state = model._find_particles_inside_neighbor_cells(
list_arr, head_arr, neighbor_cells)
mask = np.isin(so.postate, temporary_state)
assert np.all(
mask ==
True) == True, 'particles in neighbor cells has been indetified wrongly'
print('\n')
print('--------------------------------------------------')
print('the CellLinked class is verified by the given test')
print('--------------------------------------------------')
一个建议是,您应该将变量box_len
、 r_cut
和postate
作为类的变量,并在类的构造函数中初始化它们。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.