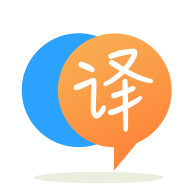
[英]In Python, how can I use a function's return statement within a second function without using global variables?
[英]Python, Tkinter: Can a list be modified within a button callback without using global variables or defining new classes?
理想情况下,我想使用像tk.StringVar
这样的内置方法来修改按钮命令中的(字符串)列表,但还需要修改后的列表继续存在,以便可以在额外的 function 调用中对其进行修改。 下面代码中的print(a)
行打印了一个类似列表的 object ('abc', 'gh', 'rstu')
,但是print(a[1])
行无法返回gh
并且a.append('xyz')
行a.append('xyz')
返回一个错误(包括在下面),表明我不能直接劫持tk.StringVar
来携带列表。 如果我应该避免使列表全局化的危险和定义 class 的开销,那么我最好的选择是什么?
import tkinter as tk
def append():
a = a_var.get()
print(a)
print(a[1])
a.append('xyz')
a_var.set(a)
a_arr = ['abc','gh','rstu']
print(a_arr)
window = tk.Tk()
a_var = tk.StringVar()
a_var.set(a_arr)
tk.Button(window, text='Append', command=append).pack()
window.mainloop()
为完整起见,上述代码返回的 output 和错误为
['abc', 'gh', 'rstu']
('abc', 'gh', 'rstu')
'
Exception in Tkinter callback
Traceback (most recent call last):
File "C:\Users\...\Python39\lib\tkinter\__init__.py", line 1884, in __call__
return self.func(*args)
File "c:\Users\...\TestAppend.py", line 7, in modify
a.append('xyz')
AttributeError: 'str' object has no attribute 'append'
如果您的目标是将 append 传递给a_arr
,则可以使用lambda
将其传递给回调。 回调不需要返回任何内容,因为您将修改 object,而不是用新的 object 替换它。
如果您对代码进行了以下修改,您会看到每次单击按钮时,数组都会增长 1。
def append(a):
a.append('xyz')
print("a:", a)
...
tk.Button(window, text='Append', command=lambda: append(a_arr)).pack()
当然,既然a_arr
已经是全局变量,你也可以直接修改
def append():
a_arr.append('xyz')
...
tk.Button(window, text='Append', command=append).pack()
如果您想使用列表,请将其转换为StringVar
import tkinter as tk
def append():
print(a_arr)
print(a_arr[1])
a_arr.append('xyz')
a_arr = ['abc','gh','rstu']
window = tk.Tk()
tk.Button(window, text='Append', command=append).pack()
window.mainloop()
按钮在没有 arguments 的情况下运行分配的 function - 但您可以使用lambda
更改它并将数组作为参数发送。 但是按钮也不知道如何处理返回值,因此您无法返回新数组作为return
- 在这里您必须使用全局变量。 当您将所有 GUI 编写为类时,您可以使用self.
然后还不错。
import tkinter as tk
class App():
def __init__(self):
self.a_arr = ['abc','gh','rstu']
self.window = tk.Tk()
tk.Button(self.window, text='Append', command=self.append).pack()
self.window.mainloop()
def append(self):
print(self.a_arr)
print(self.a_arr[1])
self.a_arr.append('xyz')
App()
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.