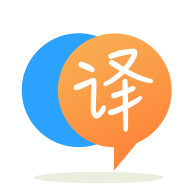
[英]How to change the height of the object by using DragGesture in SwiftUI?
[英]Change array sequence in SwiftUI DragGesture
我目前正在尝试构建一副卡片来代表用户卡片。
这是我的第一个原型,请忽略样式问题。 我希望用户能够更改卡片的顺序,我试图通过拖动手势来实现。
我的想法是,当拖动手势的平移大于卡片偏移量(或小于卡片负偏移量)时,我只需交换数组中的两个元素即可交换视图中的卡片(以及 viewModel 因为绑定)。 不幸的是,这不起作用,我不知道为什么。 这是我的观点:
struct CardHand: View {
@Binding var cards: [Card]
@State var activeCardIndex: Int?
@State var activeCardOffset: CGSize = CGSize.zero
var body: some View {
ZStack {
ForEach(cards.indices) { index in
GameCard(card: cards[index])
.offset(x: CGFloat(-30 * index), y: self.activeCardIndex == index ? -20 : 0)
.offset(activeCardIndex == index ? activeCardOffset : CGSize.zero)
.rotationEffect(Angle.degrees(Double(-2 * Double(index - cards.count))))
.zIndex(activeCardIndex == index ? 100 : Double(index))
.gesture(getDragGesture(for: index))
}
// Move the stack back to the center of the screen
.offset(x: CGFloat(12 * cards.count), y: 0)
}
}
private func getDragGesture(for index: Int) -> some Gesture {
DragGesture()
.onChanged { gesture in
self.activeCardIndex = index
self.activeCardOffset = gesture.translation
}
.onEnded { gesture in
if gesture.translation.width > 30, index < cards.count {
cards.swapAt(index, index + 1)
}
self.activeCardIndex = nil
// DEBUG: Try removing the card after drag gesture ended. Not working either.
cards.remove(at: index)
}
}
}
游戏卡:
struct GameCard: View {
let card: Card
var symbol: (String, Color) {
switch card.suit.name {
case "diamonds":
return ("♦", .red)
case "hearts":
return ("♥", .red)
case "spades":
return ("♠", .black)
case "clubs":
return ("♣", .black)
default:
return ("none", .black)
}
}
var body: some View {
ZStack {
RoundedRectangle(cornerRadius: 25)
.fill(Color.white)
.addBorder(Color.black, width: 3, cornerRadius: 25)
VStack {
Text(self.card.rank.type.description())
Text(self.symbol.0)
.font(.system(size: 100))
.foregroundColor(self.symbol.1)
}
}
.frame(minWidth: 20, idealWidth: 80, maxWidth: 80, minHeight: 100, idealHeight: 100, maxHeight: 100, alignment: .center)
}
}
后面的Model:
public struct Card: Identifiable, Hashable {
public var id: UUID = UUID()
public var suit: Suit
public var rank: Rank
}
public enum Type: Identifiable, Hashable {
case face(String)
case numeric(rankNumber: Int)
public var id: Type { self }
public func description() -> String{
switch self {
case .face(let description):
return description
case .numeric(let rankNumber):
return String(rankNumber)
}
}
}
public struct Rank: RankProtocol, Identifiable, Hashable {
public var id: UUID = UUID()
public var type: Type
public var value: Int
public var ranking: Int
}
public struct Suit: SuitProtocol, Identifiable, Hashable {
public var id: UUID = UUID()
public var name: String
public var value: Int
public init(name: String, value: Int) {
self.name = name
self.value = value
}
}
public protocol RankProtocol {
var type: Type { get }
var value: Int { get }
}
public protocol SuitProtocol {
var name: String { get }
}
谁能告诉我为什么这没有按我的预期工作? 我错过了什么基本的东西吗?
谢谢!
这里发生了很多小错误。 这是一个(粗略的)解决方案,我将在下面详细说明:
struct CardHand: View {
@Binding var cards: [Card]
@State var activeCardIndex: Int?
@State var activeCardOffset: CGSize = CGSize.zero
func offsetForCardIndex(index: Int) -> CGSize {
var initialOffset = CGSize(width: CGFloat(-30 * index), height: 0)
guard index == activeCardIndex else {
return initialOffset
}
initialOffset.width += activeCardOffset.width
initialOffset.height += activeCardOffset.height
return initialOffset
}
var body: some View {
ZStack {
ForEach(cards.indices) { index in
GameCard(card: cards[index])
.offset(offsetForCardIndex(index: index))
.rotationEffect(Angle.degrees(Double(-2 * Double(index - cards.count))))
.zIndex(activeCardIndex == index ? 100 : Double(index))
.gesture(getDragGesture(for: index))
}
// Move the stack back to the center of the screen
.offset(x: CGFloat(12 * cards.count), y: 0)
}
}
private func getDragGesture(for index: Int) -> some Gesture {
DragGesture(minimumDistance: 0, coordinateSpace: .local)
.onChanged { gesture in
self.activeCardIndex = index
self.activeCardOffset = gesture.translation
}
.onEnded { gesture in
if gesture.translation.width > 30 {
if index - 1 > 0 {
cards.swapAt(index, index - 1)
}
}
if gesture.translation.width < -30 {
cards.swapAt(index, index + 1)
}
self.activeCardIndex = nil
}
}
}
offset()
调用是有问题的。 我将两者组合成offsetForCardIndex
ZStack
,请记住cards
数组中的第一项将位于堆栈的底部并且朝向屏幕的右侧。 这影响了后来的逻辑swapAt
中检查数组的边界,以免最终尝试交换超出索引(这是之前发生的情况)我的“解决方案”仍然很粗糙——应该进行更多检查以确保阵列不会 go 越界。 此外,在您的原始版本和我的版本中,从用户体验的角度来看,能够交换超过 1 个 position 可能更有意义。 但是,在这个问题的 scope 之外,这是一个更大的问题。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.